Have you ever wondered why in Python we can directly print an object without calling a specific method? Or why we can use the len()
function to get the length of an object? Behind these seemingly simple operations lies a powerful feature of Python—Magic Methods. Today, let's delve into this intriguing topic and see how we can use magic methods to make our classes smarter and more powerful.
What Are Magic Methods
Magic methods, as the name suggests, are those special methods that seem a bit magical. In Python, they usually start and end with double underscores, such as __init__
and __str__
. These methods allow us to customize the behavior of classes, enabling instances of classes to work like built-in types.
You might ask, why use magic methods? Imagine if there were no magic methods, every time we wanted to print information about an object, we would have to explicitly call a specific method. This would not only make the code lengthy but also less intuitive. The existence of magic methods allows us to use custom classes as naturally as built-in types.
Common Magic Methods
Let's look at some common magic methods and how they make our classes more powerful.
Construction and Initialization
class Book:
def __new__(cls, *args, **kwargs):
print("Creating a new Book instance")
return super().__new__(cls)
def __init__(self, title, author):
print("Initializing Book instance")
self.title = title
self.author = author
The __new__
method is responsible for creating a new instance of a class, while the __init__
method initializes that instance. Usually, defining the __init__
method is sufficient. However, if you need to control the creation process, like implementing a singleton pattern, the __new__
method becomes useful.
String Representation
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def __str__(self):
return f"{self.title} by {self.author}"
def __repr__(self):
return f"Book('{self.title}', '{self.author}')"
book = Book("Guide to Python Magic Methods", "Zhang San")
print(book) # Output: Guide to Python Magic Methods by Zhang San
print(repr(book)) # Output: Book('Guide to Python Magic Methods', 'Zhang San')
The __str__
method defines the string representation of an object, which is called by the print()
function or the str()
function. The __repr__
method provides a more detailed string representation, usually used for debugging.
Comparison Operations
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __eq__(self, other):
if not isinstance(other, Book):
return NotImplemented
return self.title == other.title and self.author == other.author
def __lt__(self, other):
if not isinstance(other, Book):
return NotImplemented
return self.pages < other.pages
book1 = Book("Python for Beginners", "Li Si", 200)
book2 = Book("Advanced Python", "Wang Wu", 300)
book3 = Book("Python for Beginners", "Li Si", 220)
print(book1 == book3) # Output: True
print(book1 < book2) # Output: True
By defining the __eq__
and __lt__
methods, we can customize the logic for equality and size comparison between objects. Here, we determine if two books are the same based on the title and author, and compare which book is "larger" based on the number of pages.
Attribute Access
class Book:
def __init__(self, title, author):
self._title = title
self._author = author
def __getattr__(self, name):
return f"Attribute '{name}' does not exist"
def __setattr__(self, name, value):
if name.startswith('_'):
super().__setattr__(name, value)
else:
print(f"Setting attribute '{name}' is not allowed")
book = Book("Guide to Python Magic Methods", "Zhang San")
print(book.price) # Output: Attribute 'price' does not exist
book.genre = "Programming" # Output: Setting attribute 'genre' is not allowed
The __getattr__
method is called when accessing a non-existent attribute, while the __setattr__
method is called when setting any attribute. With these two methods, we can implement dynamic attribute access and control attribute setting.
Callable Objects
class Multiplier:
def __init__(self, factor):
self.factor = factor
def __call__(self, x):
return x * self.factor
double = Multiplier(2)
print(double(5)) # Output: 10
By defining the __call__
method, we can make an object callable like a function. This is useful when implementing functional programming patterns or creating configurable callback functions.
Practical Application Example
Let's look at a more complex example that demonstrates how to use multiple magic methods to create a feature-rich class:
class Library:
def __init__(self):
self.books = []
def __len__(self):
return len(self.books)
def __getitem__(self, index):
return self.books[index]
def __iter__(self):
return iter(self.books)
def __contains__(self, book):
return book in self.books
def __iadd__(self, book):
if isinstance(book, Book):
self.books.append(book)
return self
def __str__(self):
return f"Library Collection: {len(self)} books"
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def __str__(self):
return f"{self.title} by {self.author}"
library = Library()
library += Book("Python Programming", "Zhang San")
library += Book("Data Structures", "Li Si")
print(len(library)) # Output: 2
print("Python Programming" in library) # Output: True
for book in library:
print(book)
print(library[0]) # Output: Python Programming by Zhang San
print(library) # Output: Library Collection: 2 books
In this example, we defined a Library
class that uses multiple magic methods:
__len__
: Allows us to use thelen()
function to get the number of books in the library.__getitem__
: Allows us to access books in the library like a list.__iter__
: Makes the library object iterable.__contains__
: Allows us to use thein
operator to check if a book is in the library.__iadd__
: Implements the+=
operator so we can easily add new books to the library.__str__
: Defines the string representation of the library object.
With these magic methods, we created a rich, intuitive Library
class that can be operated like a built-in list type while maintaining its own features and behavior.
Considerations
While magic methods are powerful, there are a few points to keep in mind when using them:
-
Don't Overuse: Use magic methods only when truly necessary. Overuse can make the code difficult to understand and maintain.
-
Performance Considerations: Some magic methods (like
__getattr__
) may be called frequently, so pay attention to the efficiency of the implementation. -
Maintain Consistency: If you implement
__eq__
, you should usually also implement__ne__
. Similarly, if you implement__lt__
, it's best to implement other comparison methods as well. -
Document: Since the behavior of magic methods may not be intuitive, ensure to provide clear documentation for classes that use them.
Conclusion
Python's magic methods provide us with powerful tools to create rich, intuitive classes. By using these methods wisely, we can make our code more Pythonic and align better with Python's design philosophy.
What do you think about magic methods? Do they make Python's object-oriented programming more interesting and powerful? I personally believe that magic methods are one of Python's most fascinating features. They not only enhance the language's expressiveness but also provide us with an elegant way to customize the behavior of objects.
If you haven't tried using magic methods in your projects yet, I strongly encourage you to give it a try. You'll find that by using these methods, your code will become more concise, intuitive, and powerful.
Finally, remember this: magic methods are powerful, but not mysterious. They are just tools that Python provides to let our classes act more like Python's built-in types. By learning and using these methods, we can gain a deeper understanding of Python's design philosophy and become better Python programmers.
So, are you ready to wield some Python magic in your next project?
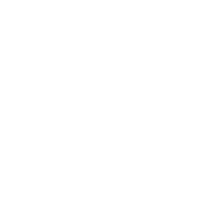
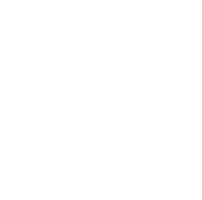