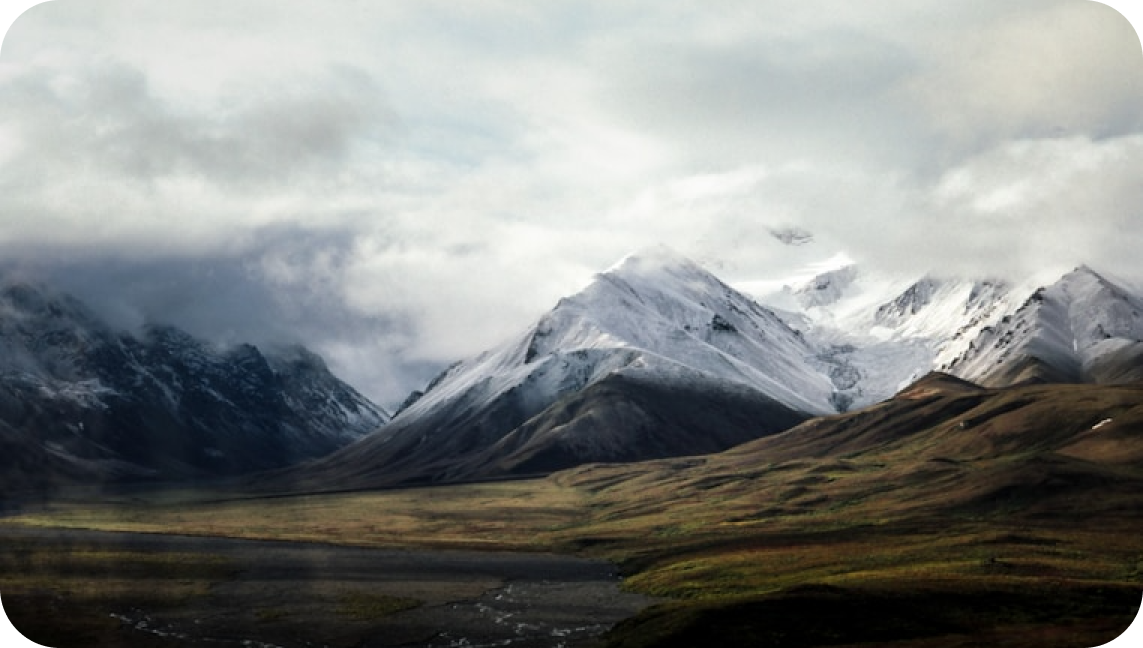
Origins
Have you ever faced these challenges: wanting to develop a Web API but not knowing where to start? Or already using Flask to develop APIs but feeling your code isn't elegant enough? As a Python developer, I deeply understand these feelings. Today, let's explore the world of Python API development together and see how to build a fully functional REST API using the Flask framework.
Framework Selection
When it comes to Python Web frameworks, Django and Flask are the most popular choices. So why am I recommending Flask?
According to the 2024 Python Developer Survey, Flask's usage rate in Web frameworks reached 47%, second only to Django's 51%. However, in the specific field of API development, Flask leads with 52% compared to Django's 43%.
I believe this is mainly due to Flask's "micro-framework" positioning. Its core framework is very lightweight, providing only the most basic functionality, which allows us to flexibly add needed extensions based on actual requirements. For example, we can choose SQLAlchemy for database support; for authentication, we can use Flask-JWT-Extended. This modular design philosophy is particularly suitable for API development.
Getting Started
Let's start with the simplest Flask API. You only need a few lines of code to build a basic API service:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def hello_world():
return jsonify({'message': 'Hello, World!'})
if __name__ == '__main__':
app.run(debug=True)
Advanced Topics
While a basic API is simple, we need to consider more aspects in real projects. I've summarized these key points from practice:
Standardization
API design should follow REST specifications. I've found many developers misunderstand REST, thinking that just using HTTP methods makes it a REST API. In fact, REST emphasizes the concept of resources.
For example, if we're developing a blog API, the resource is posts. The corresponding endpoint design should be:
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///blog.db'
db = SQLAlchemy(app)
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
content = db.Column(db.Text)
@app.route('/posts', methods=['GET'])
def get_posts():
posts = Post.query.all()
return jsonify([{'id': p.id, 'title': p.title} for p in posts])
@app.route('/posts', methods=['POST'])
def create_post():
data = request.get_json()
post = Post(title=data['title'], content=data['content'])
db.session.add(post)
db.session.commit()
return jsonify({'id': post.id}), 201
@app.route('/posts/<int:post_id>', methods=['GET'])
def get_post(post_id):
post = Post.query.get_or_404(post_id)
return jsonify({'id': post.id, 'title': post.title, 'content': post.content})
Performance
API performance optimization is an often overlooked topic. From my experience, these points are particularly important:
- Database Query Optimization
When handling large amounts of data, be careful to avoid N+1 query problems. For example, getting all posts and their author information:
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100))
author_id = db.Column(db.Integer, db.ForeignKey('user.id'))
author = db.relationship('User', backref='posts')
@app.route('/posts', methods=['GET'])
def get_posts():
# Preload author information using join
posts = Post.query.options(db.joinedload(Post.author)).all()
return jsonify([{
'id': p.id,
'title': p.title,
'author': p.author.name
} for p in posts])
- Caching Strategy
For frequently accessed but rarely changed data, caching should be used. Flask-Caching extension provides simple cache implementation:
from flask_caching import Cache
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/posts/<int:post_id>', methods=['GET'])
@cache.cached(timeout=300) # Cache for 5 minutes
def get_post(post_id):
post = Post.query.get_or_404(post_id)
return jsonify({'id': post.id, 'title': post.title})
Security
API security is paramount. I recommend implementing at least these security measures:
- Authentication and Authorization
Using JWT (JSON Web Token) for user authentication:
from flask_jwt_extended import JWTManager, create_access_token, jwt_required
app.config['JWT_SECRET_KEY'] = 'your-secret-key' # Use secure key in production
jwt = JWTManager(app)
@app.route('/login', methods=['POST'])
def login():
username = request.json.get('username')
password = request.json.get('password')
if check_password(username, password): # Implement password verification logic
access_token = create_access_token(identity=username)
return jsonify({'token': access_token})
return jsonify({'error': 'Authentication failed'}), 401
@app.route('/protected', methods=['GET'])
@jwt_required()
def protected():
return jsonify({'message': 'This is a protected resource'})
- Request Rate Limiting
Prevent API abuse:
from flask_limiter import Limiter
from flask_limiter.util import get_remote_address
limiter = Limiter(
app,
key_func=get_remote_address,
default_limits=["200 per day", "50 per hour"]
)
@app.route('/posts', methods=['POST'])
@limiter.limit("2 per minute") # Maximum 2 posts created per minute
def create_post():
# Post creation logic
pass
Testing
API testing is equally important. I recommend using pytest for unit and integration testing:
import pytest
from app import app, db
@pytest.fixture
def client():
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///:memory:'
app.config['TESTING'] = True
with app.test_client() as client:
with app.app_context():
db.create_all()
yield client
db.session.remove()
db.drop_all()
def test_get_posts(client):
response = client.get('/posts')
assert response.status_code == 200
assert isinstance(response.json, list)
def test_create_post(client):
response = client.post('/posts',
json={'title': 'Test Post', 'content': 'Test Content'})
assert response.status_code == 201
assert 'id' in response.json
Experience
Developing APIs is a gradual process. I suggest starting with the most basic functionality, ensuring clear code structure, then gradually adding more features. During this process, maintaining code maintainability and testability is very important.
Remember, there's no perfect API, but there is an API that best fits your project needs. In actual development, we need to make trade-offs based on specific scenarios. For example, an internal API might not need particularly strict access control, while a public-facing API needs more security measures.
Finally, here's a tip: when developing APIs, it's recommended to use API testing tools like Postman. It not only helps you test APIs more conveniently but can also automatically generate API documentation. This can greatly improve development efficiency.
Looking Forward
Looking to the future, I believe API development will become increasingly important. With the popularity of microservice architecture and the mainstreaming of frontend-backend separation, mastering API development skills becomes particularly crucial.
Python, as a concise and elegant language, coupled with flexible frameworks like Flask, provides an excellent technical choice for API development. What do you think? Feel free to share your API development experience and insights in the comments.
After all this discussion, aren't you eager to try it yourself? I suggest starting with a simple project, like developing a todo list API. Learning through practice, I believe you'll quickly grasp the essence of Flask API development.
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
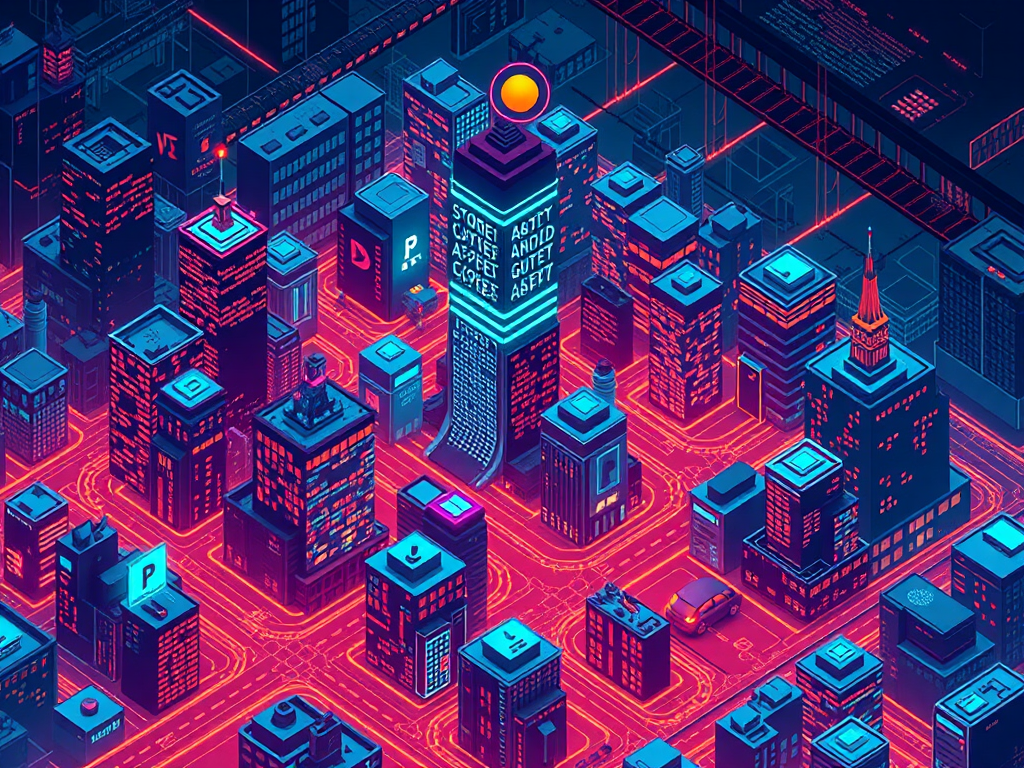
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
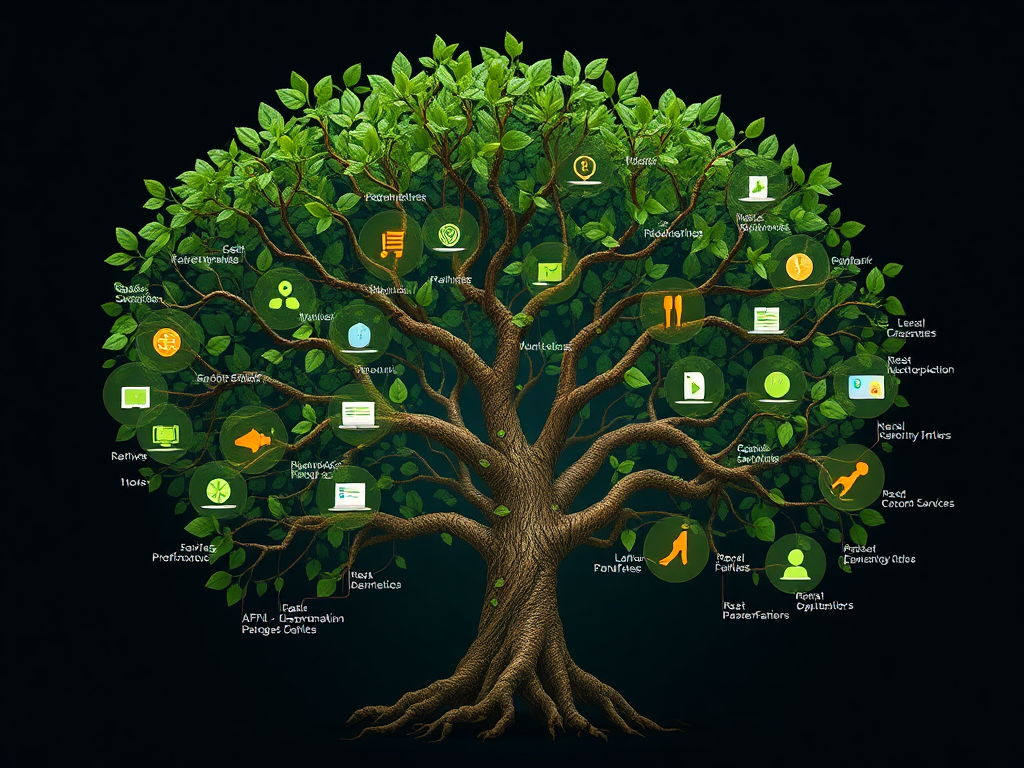
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
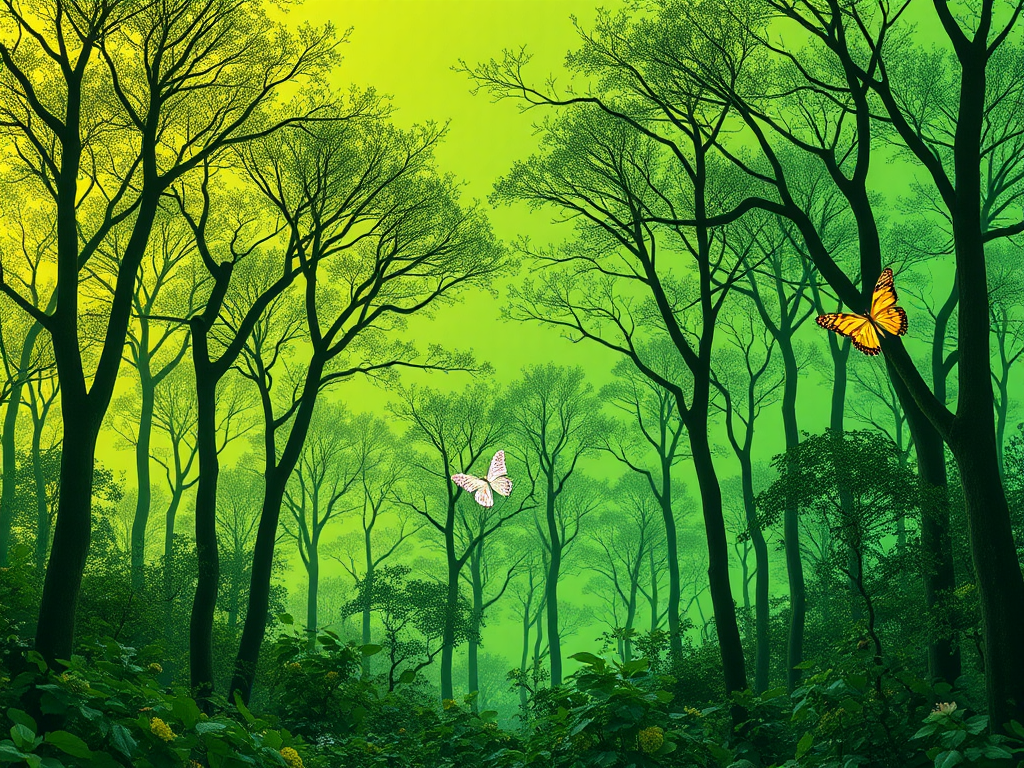
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services