Origin
Have you encountered situations where your Python code suddenly throws an error and stops running? As a Python developer, I deeply understand the importance of exception handling. I remember when I first started programming, I was always caught off guard by various unexpected situations. After years of practical experience, I gradually realized that an elegant exception handling mechanism not only makes programs more robust but also helps us quickly locate and solve problems.
Common Misconceptions
Many Python beginners have some misconceptions about exception handling. Some think "as long as the code runs, it's fine," while others feel "exception handling is too troublesome." I used to think this way too, until one time when a production system I developed crashed due to lack of proper exception handling, causing serious consequences. This made me realize that exception handling isn't just an optional decoration, but a standard feature of excellent code.
Basic Concepts
In Python, exceptions are special objects used to represent errors that occur during program execution. When the Python interpreter encounters an error, it creates an exception object. If this exception object isn't properly handled, the program will terminate and display a traceback.
Let's look at a simple example:
def divide_numbers(a, b):
try:
result = a / b
return result
except ZeroDivisionError:
return "Cannot divide by zero"
except TypeError:
return "Please input numbers"
print(divide_numbers(10, 2)) # Output: 5.0
print(divide_numbers(10, 0)) # Output: Cannot divide by zero
print(divide_numbers(10, "2")) # Output: Please input numbers
Deep Analysis
Exception handling is more than just catching errors. In my view, it's an art that requires deep understanding of its working mechanism. Python's exception handling mechanism mainly includes the following key components:
- try block: Contains code that might raise exceptions
- except block: Catches and handles specific types of exceptions
- else block: Runs when the code in the try block executes successfully
- finally block: Code that executes whether an exception occurs or not
Let me give you a practical example. Suppose we're developing a file processing system:
def process_file(filename):
try:
with open(filename, 'r', encoding='utf-8') as file:
content = file.read()
processed_data = content.upper()
except FileNotFoundError:
print(f"File {filename} does not exist")
return None
except UnicodeDecodeError:
print(f"File {filename} has encoding error")
return None
except Exception as e:
print(f"Unknown error occurred while processing file: {str(e)}")
return None
else:
print("File processing successful")
return processed_data
finally:
print("File processing operation completed")
result = process_file("test.txt")
Best Practices
Through years of development experience, I've summarized some best practices for exception handling:
Exception Granularity
The granularity of exception handling should be moderate. If too coarse, important error information might be missed; if too fine, the code becomes verbose and hard to maintain. My suggestion is to determine the granularity of exception handling based on the importance of business logic.
Here's a practical example:
class UserManager:
def create_user(self, username, email, password):
try:
self.validate_input(username, email, password)
user = self.save_to_database(username, email, password)
self.send_welcome_email(email)
return user
except ValidationError as e:
log.error(f"Input validation failed: {str(e)}")
raise
except DatabaseError as e:
log.error(f"Database operation failed: {str(e)}")
raise
except EmailError as e:
log.warning(f"Welcome email sending failed: {str(e)}")
# Email sending failure doesn't affect user creation
return user
Custom Exceptions
In actual development, Python's built-in exception types may not accurately describe error situations in business scenarios. This is when we need to define custom exception classes.
class BusinessError(Exception):
"""Business exception base class"""
def __init__(self, message, code=None):
self.message = message
self.code = code
super().__init__(self.message)
class UserNotFoundError(BusinessError):
"""User not found exception"""
def __init__(self, user_id):
message = f"User ID {user_id} does not exist"
super().__init__(message, code="USER_NOT_FOUND")
class InvalidParameterError(BusinessError):
"""Invalid parameter exception"""
def __init__(self, parameter_name, reason):
message = f"Parameter {parameter_name} is invalid: {reason}"
super().__init__(message, code="INVALID_PARAMETER")
Advanced Techniques
When handling complex business logic, we often need to use some advanced exception handling techniques.
Context Managers
Python's with statement provides an elegant way to handle resource acquisition and release. We can ensure proper resource handling by implementing context managers:
class DatabaseConnection:
def __init__(self, connection_string):
self.connection_string = connection_string
self.connection = None
def __enter__(self):
try:
self.connection = self.connect_to_database()
return self
except Exception as e:
raise DatabaseError(f"Database connection failed: {str(e)}")
def __exit__(self, exc_type, exc_val, exc_tb):
if self.connection:
try:
self.connection.close()
except Exception as e:
print(f"Error occurred while closing database connection: {str(e)}")
return False # Return False to allow exception propagation
with DatabaseConnection("mysql://localhost/mydb") as db:
db.execute("SELECT * FROM users")
Exception Chaining
When handling exceptions, sometimes we need to preserve the original exception information while raising a new exception. Python provides an exception chaining mechanism to achieve this:
class DataProcessError(Exception):
pass
def process_data(data):
try:
return json.loads(data)
except json.JSONDecodeError as e:
raise DataProcessError("Data format error") from e
def handle_request(request):
try:
data = process_data(request.body)
# Process data...
except DataProcessError as e:
print(f"Error: {e}")
print(f"Original error: {e.__cause__}")
Practical Case
Let's combine all the knowledge we've learned through a complete practical case. This is a simplified file processing system:
import logging
from pathlib import Path
from typing import Optional, List, Dict
import json
class FileProcessingError(Exception):
"""Base class for file processing related exceptions"""
pass
class FileReader:
def __init__(self, base_path: str):
self.base_path = Path(base_path)
self.setup_logging()
def setup_logging(self):
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
self.logger = logging.getLogger(__name__)
def read_file(self, filename: str) -> Optional[Dict]:
"""Read and parse JSON file"""
file_path = self.base_path / filename
try:
if not file_path.exists():
raise FileNotFoundError(f"File {filename} does not exist")
if not file_path.suffix == '.json':
raise FileProcessingError(f"File {filename} is not JSON format")
with file_path.open('r', encoding='utf-8') as f:
try:
return json.load(f)
except json.JSONDecodeError as e:
raise FileProcessingError(f"JSON parsing error") from e
except Exception as e:
self.logger.error(f"Error occurred while processing file {filename}: {str(e)}")
if isinstance(e, FileProcessingError):
raise
raise FileProcessingError(f"File processing failed: {str(e)}")
def process_directory(self) -> List[Dict]:
"""Process all JSON files in the directory"""
results = []
for file_path in self.base_path.glob('*.json'):
try:
data = self.read_file(file_path.name)
if data:
results.append(data)
except FileProcessingError as e:
self.logger.warning(f"Skipping file {file_path.name}: {str(e)}")
continue
return results
if __name__ == "__main__":
reader = FileReader("data")
try:
results = reader.process_directory()
print(f"Successfully processed {len(results)} files")
except Exception as e:
print(f"Program execution failed: {str(e)}")
Experience Summary
Through years of Python development experience, I've summarized the following insights about exception handling:
-
Exception handling should be hierarchical. Lower-level functions throw specific exceptions, upper-level functions catch and convert them into business exceptions.
-
Logging is important. When exceptions occur, sufficient context information should be recorded for problem locating.
-
Don't abuse exception handling. In some cases, using if statements is more appropriate, such as checking if a dictionary key exists.
-
Maintain exception atomicity. Operations in a try block should be a complete transaction, either all succeed or all fail.
-
Consider performance in exception handling. Too many try-except blocks in frequently called code will affect performance.
Future Outlook
Python's exception handling mechanism continues to evolve. Python 3.10 introduced the match statement, providing new possibilities for exception handling. I believe that as Python language develops, the exception handling mechanism will become more powerful and flexible.
Conclusion
Exception handling is not just an error handling mechanism; it's key to writing robust code. Through this article's sharing, have you gained new insights into Python's exception handling? Feel free to share your thoughts and experiences in the comments.
Remember, excellent code should not only work under normal conditions but also handle exceptions gracefully. What do you think?
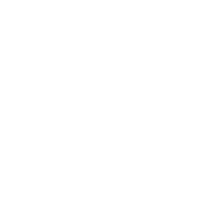
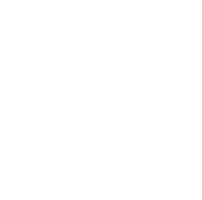