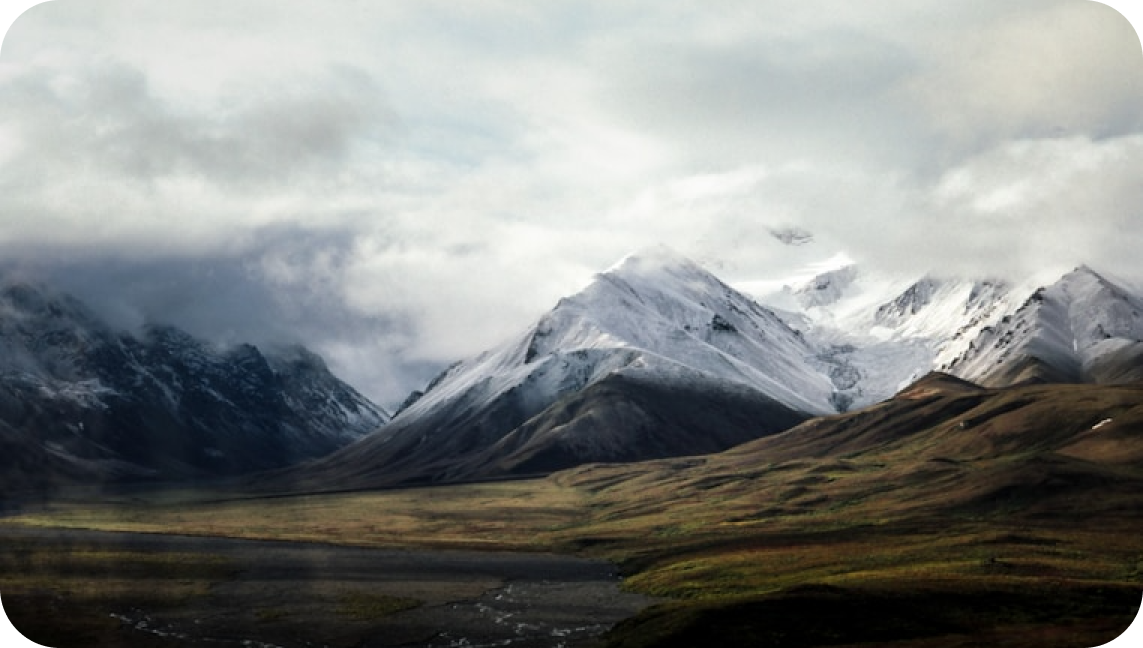
Origin
Have you encountered this frustration: you wrote a seemingly simple Python program, but it runs particularly slow? Especially when handling IO operations like network requests and file operations, the program feels stuck in a quagmire. This is actually due to the limitations of synchronous programming models.
I remember when I first started learning Python, I was often troubled by this issue. It wasn't until I encountered asynchronous programming that I truly found the solution. Today, I'll share with you the core knowledge of Python asynchronous programming to help you break through performance bottlenecks.
Concepts
Before diving into details, let's understand several key concepts.
Synchronous programming is like queuing for bubble tea - everyone has to wait in line for the person in front to finish buying. Asynchronous programming is more like dining at a restaurant - you can find a seat first, and the server will bring the food to your table when it's ready.
Python's asynchronous programming is primarily implemented through coroutines. A coroutine can be understood as a "pausable function" - when encountering IO operations, it actively yields control to let other coroutines continue executing. This mechanism enables concurrency even in a single thread.
Basics
Let's understand the basic usage of asynchronous programming through a simple example:
import asyncio
async def hello(name):
print(f'Hello {name}...')
await asyncio.sleep(1) # Simulate IO operation
print(f'Goodbye {name}!')
async def main():
await asyncio.gather(
hello("Alice"),
hello("Bob"),
hello("Charlie")
)
asyncio.run(main())
Advanced
The power of asynchronous programming goes far beyond this. In practical applications, we often need to handle more complex scenarios. For example, when you need to process multiple network requests simultaneously:
import asyncio
import aiohttp
import time
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'http://example.com/api1',
'http://example.com/api2',
'http://example.com/api3'
]
async with aiohttp.ClientSession() as session:
tasks = [fetch_data(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return results
if __name__ == '__main__':
start = time.perf_counter()
asyncio.run(main())
print(f'Total time: {time.perf_counter() - start:.2f} seconds')
Practice
In my years of Python development experience, I've found that asynchronous programming is particularly suitable for the following scenarios:
- Network Application Development For example, when developing a high-concurrency web server, using an asynchronous framework can significantly improve performance. FastAPI is a good example:
from fastapi import FastAPI
import asyncio
app = FastAPI()
async def process_data():
await asyncio.sleep(1) # Simulate time-consuming operation
return {"status": "success"}
@app.get("/async")
async def async_endpoint():
result = await process_data()
return result
- Data Processing When dealing with large amounts of data, especially involving IO operations, asynchronous programming can greatly improve efficiency:
import asyncio
import aiofiles
async def process_file(filename):
async with aiofiles.open(filename, mode='r') as file:
content = await file.read()
# Process file content
return len(content)
async def main():
files = ['file1.txt', 'file2.txt', 'file3.txt']
tasks = [process_file(f) for f in files]
results = await asyncio.gather(*tasks)
return sum(results)
Optimization
In practical development, I've summarized some key techniques for improving asynchronous program performance:
-
Proper Task Grouping Not all tasks are suitable for concurrent execution. I've found that grouping tasks by characteristics can achieve better performance. For example, separating CPU-intensive and IO-intensive tasks.
-
Resource Management Asynchronous programs also need resource management. For example, using connection pools to reuse database connections:
import asyncpg
async def get_db_pool():
return await asyncpg.create_pool(
host='localhost',
database='mydb',
user='user',
password='password',
min_size=5,
max_size=20
)
async def query_data():
pool = await get_db_pool()
async with pool.acquire() as connection:
return await connection.fetch("SELECT * FROM users")
- Error Handling Error handling in asynchronous programs requires special attention. I recommend using try/except/finally structures to ensure proper resource release:
async def safe_operation():
try:
async with aiohttp.ClientSession() as session:
async with session.get('http://example.com') as response:
return await response.text()
except aiohttp.ClientError as e:
logging.error(f"Network error: {e}")
raise
finally:
# Clean up resources
pass
Reflection
In the process of learning and using Python asynchronous programming, I gradually realized several important issues:
-
Balance between Performance and Complexity While asynchronous programming can bring performance improvements, it also increases code complexity. We need to weigh the pros and cons in actual projects. From my experience, if an application is primarily IO-intensive, adopting asynchronous programming is worthwhile.
-
Debugging Challenges Debugging asynchronous programs is more challenging than synchronous ones. You need to master more debugging techniques, such as using asyncio's debugging tools:
import asyncio
import logging
async def debug_example():
loop = asyncio.get_running_loop()
loop.set_debug(True)
logging.basicConfig(level=logging.DEBUG)
await asyncio.sleep(1)
Looking Forward
Python's asynchronous programming ecosystem is rapidly developing. I believe more excellent asynchronous frameworks and tools will emerge in the coming years. As developers, we need to continue learning to keep up with technological developments.
What are your thoughts and experiences with Python asynchronous programming? Feel free to share your insights in the comments. If you found this article helpful, please share it with other Python developers.
Let's explore more possibilities in the world of asynchronous programming together. Remember, programming is not just a technology, but also an art. While pursuing performance, we should also pay attention to code elegance and maintainability.
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
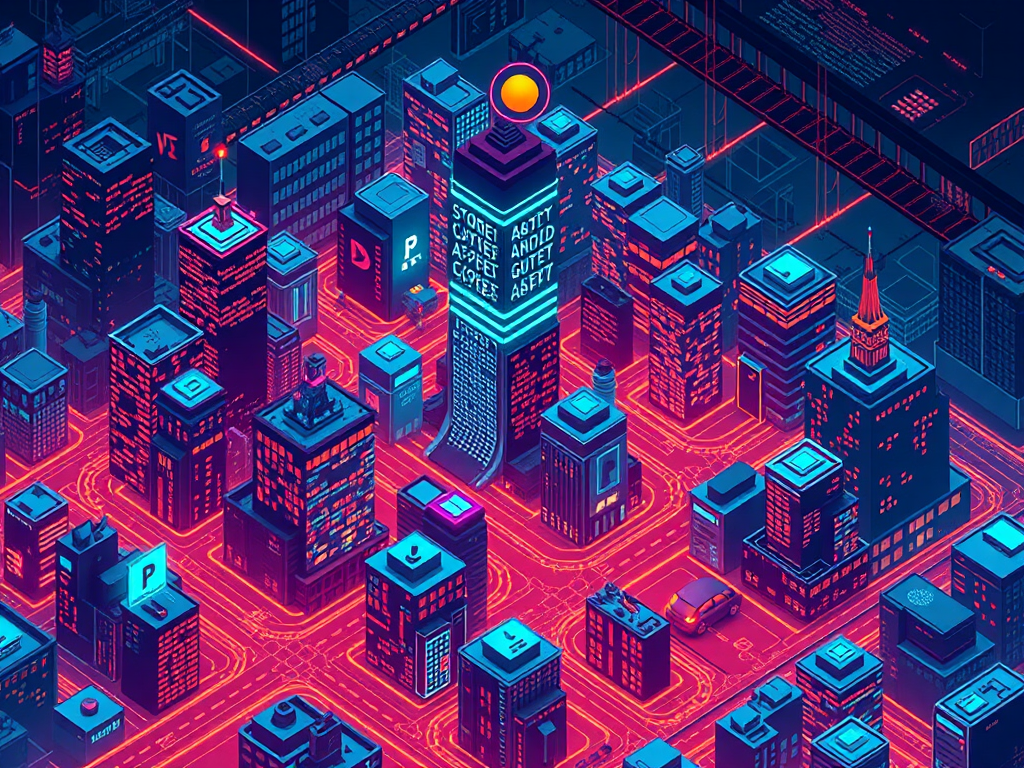
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
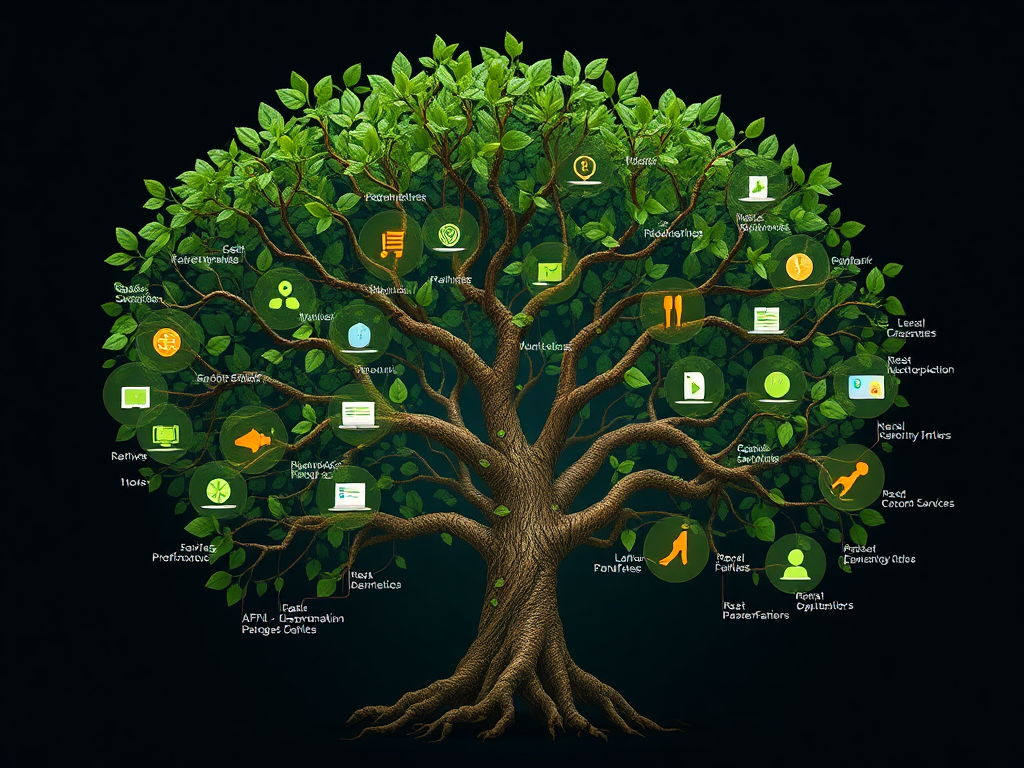
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
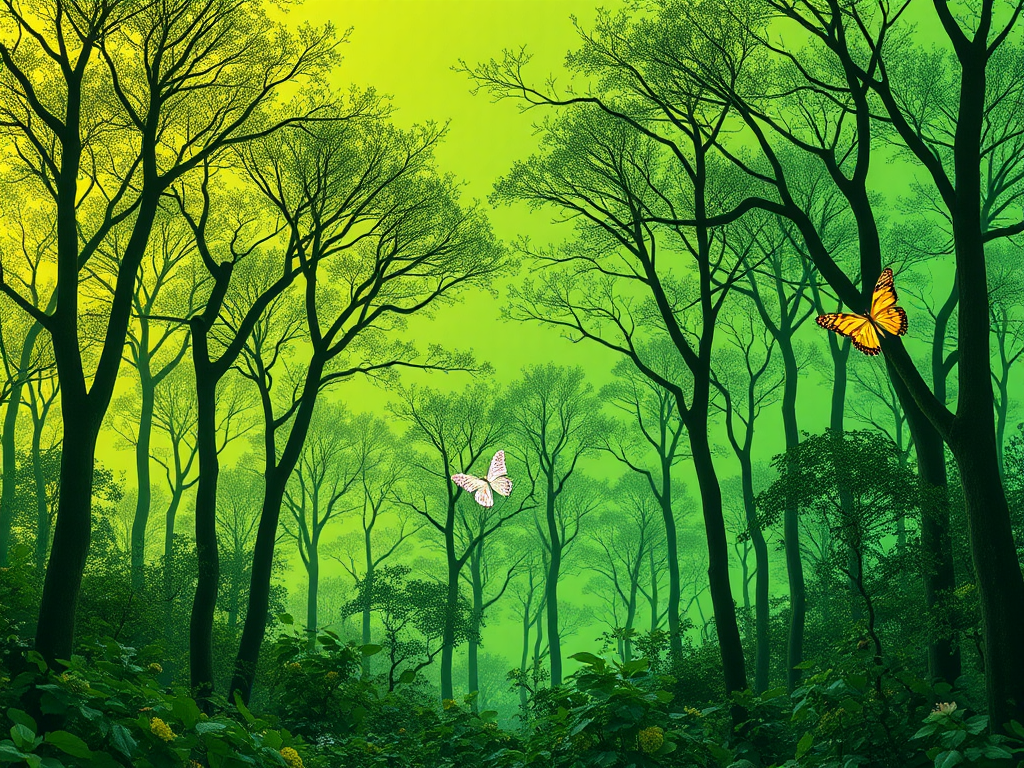
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services