Introduction
Hello, today I want to discuss an important topic in Python asynchronous programming - how to build a robust async API system. As a Python developer, I deeply feel that async programming is both challenging and fascinating. It's like a double-edged sword - when used properly, it can significantly improve system performance; when used improperly, it can make code difficult to maintain and debug.
Let's dive deep into this topic. I'll combine practical experience to help you understand the core concepts and best practices of async programming in the most straightforward way.
Basic Concepts
Before we begin, we need to understand some basic concepts. Do you know why we need async programming? Imagine you're developing a web application that needs to handle many concurrent requests. With synchronous programming, each request blocks the thread until processing is complete. It's like having a cashier who can only serve customers one at a time, which is very inefficient.
Async programming is like having multiple cashiers working simultaneously - when one cashier is waiting for a customer to find change, they can serve the next customer. In Python, we use async/await syntax to implement this kind of asynchronous operation.
Here's a simple example of an async function:
import asyncio
async def process_request(request_id):
print(f"Start processing request {request_id}")
await asyncio.sleep(1) # Simulate IO operation
print(f"Request {request_id} processing complete")
return f"Result {request_id}"
async def main():
tasks = [process_request(i) for i in range(3)]
results = await asyncio.gather(*tasks)
print(f"All requests processed: {results}")
asyncio.run(main())
Would you like me to explain what this code means in detail?
Exception Handling
When it comes to async programming, exception handling might be the most headache-inducing part. In synchronous code, we're used to using try-except blocks to catch exceptions. But in async code, things get much more complicated. Because async operations might execute at some future point, exceptions might only appear then.
I once encountered this issue: when handling many concurrent requests, one request failed, but the exception information was "swallowed," making it very difficult to troubleshoot. Later, I developed a more complete exception handling solution:
async def safe_request(url):
try:
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
if response.status == 200:
return await response.text()
else:
raise Exception(f"HTTP {response.status}")
except Exception as e:
print(f"Error occurred while processing request {url}: {str(e)}")
# Log detailed error information
import traceback
traceback.print_exc()
return None
async def process_multiple_requests(urls):
tasks = [safe_request(url) for url in urls]
results = await asyncio.gather(*tasks, return_exceptions=True)
# Process results
success_count = sum(1 for r in results if r is not None)
print(f"Successfully processed {success_count} requests, failed {len(results) - success_count}")
return results
Resource Management
Do you know what's the most problematic area in async programming? That's right, it's resource management. Resources like database connections, file handles, and network connections can easily cause resource leaks if not managed correctly.
Here's a database connection pool management solution I use in actual projects:
class DatabasePool:
def __init__(self):
self.pool = None
async def initialize(self):
self.pool = await aiomysql.create_pool(
host='localhost',
user='user',
password='password',
db='database',
minsize=5,
maxsize=20
)
async def close(self):
if self.pool:
self.pool.close()
await self.pool.wait_closed()
async def execute_query(self, query, params=None):
async with self.pool.acquire() as conn:
async with conn.cursor() as cur:
await cur.execute(query, params)
return await cur.fetchall()
class DatabasePoolManager:
def __init__(self):
self.pool = DatabasePool()
async def __aenter__(self):
await self.pool.initialize()
return self.pool
async def __aexit__(self, exc_type, exc_val, exc_tb):
await self.pool.close()
Performance Optimization
When talking about async programming, we must discuss performance optimization. Many developers focus only on the "async" concept when using async programming, but overlook the key points of performance optimization.
I've summarized several important performance optimization techniques:
- Properly control concurrency:
async def process_items(items, max_concurrency=10):
semaphore = asyncio.Semaphore(max_concurrency)
async def process_with_limit(item):
async with semaphore:
return await process_item(item)
tasks = [process_with_limit(item) for item in items]
return await asyncio.gather(*tasks)
- Use batch processing to reduce IO operations:
async def batch_process(items, batch_size=100):
for i in range(0, len(items), batch_size):
batch = items[i:i + batch_size]
async with self.pool.acquire() as conn:
async with conn.cursor() as cur:
# Build batch insert statement
query = "INSERT INTO table (field1, field2) VALUES %s"
values = [(item.field1, item.field2) for item in batch]
await cur.executemany(query, values)
Testing Strategy
Testing async code is another challenging topic. I've found that many developers often feel confused when writing tests for async code. Here's a practical testing framework I'd like to share:
import pytest
import asyncio
@pytest.mark.asyncio
async def test_async_function():
# Prepare test data
test_data = [1, 2, 3]
# Execute async operation
results = await process_items(test_data)
# Verify results
assert len(results) == len(test_data)
assert all(r is not None for r in results)
async def mock_async_operation():
await asyncio.sleep(0.1)
return "mock_result"
@pytest.mark.asyncio
async def test_with_mock(mocker):
# Use mock to replace real async operation
mocker.patch('your_module.real_async_operation',
side_effect=mock_async_operation)
result = await your_function()
assert result == "mock_result"
Monitoring and Logging
In actual production environments, monitoring and logging are crucial for maintaining async systems. I developed a simple but practical async logging decorator:
import functools
import logging
import time
from typing import Callable
def async_logger(func: Callable):
@functools.wraps(func)
async def wrapper(*args, **kwargs):
start_time = time.time()
try:
result = await func(*args, **kwargs)
elapsed_time = time.time() - start_time
logging.info(
f"Function: {func.__name__} "
f"Args: {args} {kwargs} "
f"Completed in {elapsed_time:.2f}s"
)
return result
except Exception as e:
elapsed_time = time.time() - start_time
logging.error(
f"Function: {func.__name__} "
f"Args: {args} {kwargs} "
f"Failed after {elapsed_time:.2f}s "
f"Error: {str(e)}"
)
raise
return wrapper
Practical Case
Let's connect all the concepts we discussed earlier through a practical case. Suppose we need to develop an async data processing system:
class AsyncDataProcessor:
def __init__(self):
self.db_pool = None
self.processing = False
self.processed_count = 0
async def initialize(self):
self.db_pool = await aiomysql.create_pool(
host='localhost',
user='user',
password='password',
db='database',
minsize=5,
maxsize=20
)
@async_logger
async def process_data(self, data_batch):
if not self.processing:
self.processing = True
try:
results = await self._process_batch(data_batch)
self.processed_count += len(results)
return results
finally:
self.processing = False
else:
raise RuntimeError("Processor is busy")
async def _process_batch(self, data_batch):
results = []
async with self.db_pool.acquire() as conn:
async with conn.cursor() as cur:
for item in data_batch:
try:
await cur.execute(
"INSERT INTO processed_data (data) VALUES (%s)",
(item,)
)
results.append(item)
except Exception as e:
logging.error(f"Error processing data {item}: {str(e)}")
return results
async def close(self):
if self.db_pool:
self.db_pool.close()
await self.db_pool.wait_closed()
Final Thoughts
Through this article, we've deeply explored the core concepts and best practices in Python async programming. From basic async/await syntax to exception handling, resource management, performance optimization, testing strategies, and monitoring logging, we've built a complete knowledge system.
Have you noticed that while async programming increases code complexity, the performance improvements and resource utilization it brings are worth it? In real projects, we need to weigh the scenarios for using async programming, as not all operations are suitable for async approaches.
Finally, I'd like to ask you a question: Have you encountered any async programming challenges in your projects? How did you solve them? Feel free to share your experiences and thoughts in the comments section.
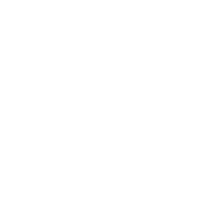