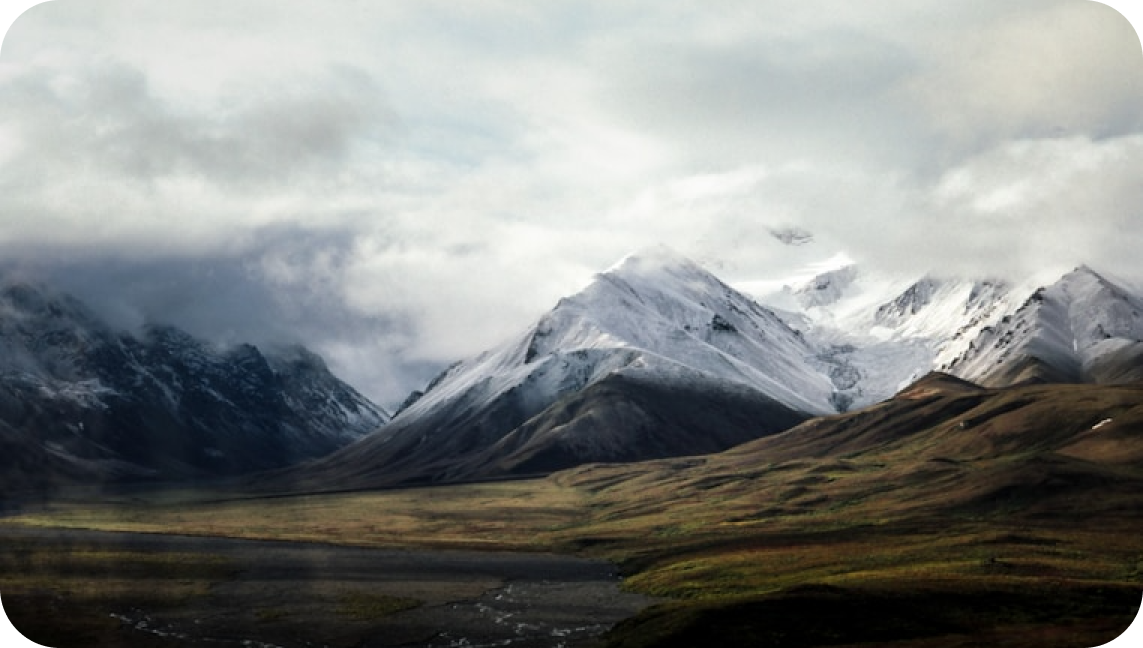
In modern web development, RESTful APIs play a crucial role. Using an excellent Python web framework can greatly simplify the development and deployment process of APIs. Next, let's compare the mainstream Python RESTful API frameworks and look at their respective features and pros and cons.
Flask: Simple and Easy to Start
Flask has long been one of the most popular web frameworks in the Python community. It is known as "small and beautiful", and its usage is extremely simple with a gentle learning curve. Of course, to build RESTful APIs, we still need to rely on some extension libraries.
Basic Functionality
As a "microframework", Flask itself only provides the most basic functionality such as routing and request handling. To build RESTful APIs, we first need to define routes to distinguish different HTTP methods (GET, POST, PUT, DELETE), and then handle the requests and return JSON-formatted data in the corresponding view functions.
Here's a code example:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/users', methods=['GET'])
def get_users():
users = [...] # Get user list from database
return jsonify(users)
@app.route('/api/users', methods=['POST'])
def create_user():
user_data = request.get_json()
# Process data and save to database
return jsonify(user_data), 201
if __name__ == '__main__':
app.run(debug=True)
Flask-RESTful Extension
The above approach works, but it's a bit cumbersome. The Flask-RESTful extension was created to simplify the development of RESTful APIs.
from flask import Flask
from flask_restful import Api, Resource
app = Flask(__name__)
api = Api(app)
class UserAPI(Resource):
def get(self):
users = [...] # Get user list from database
return users
def post(self):
user_data = request.get_json()
# Process data and save to database
return user_data, 201
api.add_resource(UserAPI, '/api/users')
You can see that after using Flask-RESTful, the code becomes more concise. We only need to define a resource class, with different HTTP methods corresponding to methods with the same name in the class. The framework will automatically dispatch requests and handle responses for us.
In addition to this, Flask-RESTful also provides advanced features such as request parameter parsing, data format support, authentication, and more, which can greatly improve development efficiency.
Database Integration and Authentication
To build production-level APIs, we usually need to integrate databases and add authentication features. The Flask community has a wealth of extension libraries that can meet these needs.
For example, Flask-SQLAlchemy can easily integrate SQLAlchemy ORM to interact with mainstream databases. Flask-JWT provides a JWT-based authentication solution.
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
from flask_jwt import JWT
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///app.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
def authenticate(username, password):
user = User.query.filter_by(username=username).first()
if user and user.verify_password(password):
return user
def identity(payload):
user_id = payload['identity']
return User.query.get(user_id)
jwt = JWT(app, authenticate, identity)
With the above configuration, we can use databases and JWT authentication in the Flask application. However, the code complexity also increases accordingly.
Deployment Options
Flask applications can run using the built-in development server, but in production environments, we need to use more powerful WSGI servers like Gunicorn. Deploying Flask applications to cloud platforms (such as Heroku, AWS) is also a good choice.
If the application has a large amount of traffic, we can also consider using Nginx as a reverse proxy to improve concurrency. Or use Docker containerization for better portability and scalability.
Overall, the Flask ecosystem is very comprehensive, and by combining various extension libraries, it can meet various needs for building RESTful APIs. Its advantages are simplicity, lightweightness, and suitability for small projects for quick start-up. The downside is that it requires integrating various components, resulting in higher code complexity.
Django REST Framework: Comprehensive Features
Django, the "heavyweight" web framework, can also be used to build RESTful APIs. In fact, Django officially provides a dedicated extension, Django REST Framework (DRF), which offers comprehensive support for building APIs.
DRF Basics
The core concepts of DRF are Serializer and View. Serializers are used to convert between Django model instances and JSON data. Views define the processing logic corresponding to different HTTP methods.
from rest_framework import serializers, views, response
class UserSerializer(serializers.ModelSerializer):
class Meta:
model = User
fields = ('id', 'username', 'email')
class UserViewSet(views.ViewSet):
def list(self, request):
users = User.objects.all()
serializer = UserSerializer(users, many=True)
return response.Response(serializer.data)
def create(self, request):
serializer = UserSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
return response.Response(serializer.data, status=201)
return response.Response(serializer.errors, status=400)
router = routers.DefaultRouter()
router.register(r'users', UserViewSet)
In the above example, we first define the UserSerializer to describe the fields of the User model. Then in the UserViewSet, we use the serializer to complete data serialization and deserialization, and implement the logic for getting the user list and creating a new user, respectively.
Finally, we use the router provided by DRF to register the view to the URL routing. This approach can automatically generate standard RESTful-style URLs for us.
Advanced Features
In addition to basic functionality, DRF also provides many powerful features:
- Authentication and permissions: Built-in multiple authentication methods (such as Token, Session, JWT), and object-level permission control.
- ViewSets and routers: ViewSets and Routers can simplify view and URL mapping definitions.
- Parsing and rendering: Supports multiple request/response data formats, such as JSON, XML, YAML, etc.
- Pagination and filtering: Built-in pagination and data filtering functionality, suitable for building large-scale APIs.
- Documentation generation: Can automatically generate interactive API documentation.
These features make DRF highly suitable for building large-scale, highly complex RESTful API systems. However, compared to Flask, its learning curve is steeper.
Deployment Options
Django applications can be deployed to common WSGI servers such as Gunicorn, uWSGI, etc. Nginx can also be used as a reverse proxy to improve concurrency.
Similar to Flask, we can also choose to deploy Django applications to cloud platforms or use Docker containerization. Overall, there are plenty of deployment options.
Django REST Framework is comprehensive in features and highly extensible, but this also means higher complexity and overhead. If your project is large-scale with high API requirements, DRF can be a good choice. If you only need to build a small API service, Flask might be more suitable.
FastAPI: High-Performance Newcomer
FastAPI is a Python web framework that has emerged in recent years, touting "high performance while maintaining a low learning curve". It is designed specifically for building RESTful APIs and has been highly praised for its exceptional performance and development efficiency.
Quick Start
FastAPI's design philosophy is "high performance, efficient coding, easy to learn, robust, and production-ready". Its biggest highlight is providing an extremely concise way to define API routes.
from fastapi import FastAPI
app = FastAPI()
@app.get('/users')
def get_users():
users = [...] # Get user list from database
return users
@app.post('/users')
def create_user(user: User):
# Process data and save to database
return user
You can see that FastAPI's route definitions are very concise and intuitive. We only need to use decorators to specify the request method and path, and handle the request and return data in the corresponding function.
FastAPI will automatically infer the data format from the function parameters and return annotations, and perform automatic serialization/deserialization. If needed, we can also explicitly define the request body and response models.
More Features
In addition to being simple and easy to use, FastAPI also has many powerful features:
- Data models and validation: You can use the Pydantic library to define request/response data models and perform automatic data validation.
- Automatic interactive documentation: FastAPI will automatically generate OpenAPI documentation based on the code and provide an interactive API documentation interface.
- Asynchronous support: FastAPI is based on ASGI and supports async/await syntax for asynchronous programming, delivering excellent performance.
- Database support: Can be integrated with mainstream Python ORM libraries like SQLAlchemy and Tortoise ORM.
- Dependency injection system: Improves code maintainability through dependency injection and decoupling.
Additionally, FastAPI also provides comprehensive security support, such as API keys, OAuth2, and JWT authentication.
Deployment Options
FastAPI applications can use Uvicorn or Hypercorn as the ASGI server to run. Traditional WSGI servers like Gunicorn can also be used for deployment.
Similar to other frameworks, we can also choose to deploy FastAPI applications to cloud platforms or Docker containers. Overall, FastAPI offers very flexible deployment options.
FastAPI combines simplicity, high performance, and modern features, making it an excellent choice for building RESTful APIs. If you plan to develop a new API service, FastAPI is definitely worth considering. However, its ecosystem is relatively young, and support for some features or tools may not be as mature yet.
Falcon: Minimalist and High-Performance
Falcon is another high-performance Python web framework, with a design philosophy of "minimalism". Falcon itself only provides the most basic functionality, and other features need to be integrated with third-party libraries. This is similar to Flask in some ways.
Basic Usage
Falcon's API design is very concise, requiring only the definition of resource classes and request handlers.
import falcon
class UserResource:
def on_get(self, req, resp):
users = [...] # Get user list from database
resp.media = users
def on_post(self, req, resp):
user_data = req.media
# Process data and save to database
resp.status = falcon.HTTP_201
app = falcon.App()
users = UserResource()
app.add_route('/users', users)
You can see that Falcon's code structure is very concise. We only need to define a resource class and implement the handler methods corresponding to different HTTP methods within it. Falcon will automatically dispatch requests to the corresponding handlers based on the request method.
Similar to Flask, Falcon itself only provides basic functionality like routing and middleware, and other features need to be integrated separately.
Middleware and Hooks
Falcon's middleware mechanism is very powerful, allowing you to execute custom logic at various stages of the request/response lifecycle. This makes it easy to add various features like authentication, caching, rate limiting, and more.
In addition to middleware, Falcon also provides a Hook mechanism that allows you to inject custom logic into the resource lifecycle, enabling more fine-grained control.
Data Validation and Serialization
Due to Falcon's "minimalist" philosophy, it does not provide data validation and serialization functionality out of the box. However, we can use third-party libraries like Marshmallow to implement these features.
from marshmallow import Schema, fields
class UserSchema(Schema):
id = fields.Int()
username = fields.Str(required=True)
email = fields.Email()
schema = UserSchema()
def on_get(self, req, resp):
users = [...] # Get user list from database
resp.media = schema.dump(users, many=True)
def on_post(self, req, resp):
user_data = schema.load(req.media)
# Process data and save to database
With the above code, we can implement request data validation and response data serialization in the Falcon application.
Asynchronous Support and Deployment
Falcon supports asynchronous programming and can be integrated with ASGI servers like Gunicorn for high-performance asynchronous I/O operations.
Similar to other frameworks, we can also choose to deploy Falcon applications to cloud platforms or Docker containers.
Overall, Falcon is a very minimalist and high-performance web framework, highly suitable for building RESTful APIs. Its advantages are concise code and excellent performance, while its downside is the need to integrate various features separately, resulting in lower extensibility. If your project has very high performance requirements and does not need many additional features, Falcon can be a good choice.
Pyramid: Flexible and Extensible
Pyramid is a long-standing Python web framework known for its flexibility and extensibility. Although not particularly well-suited for building RESTful APIs out of the box, we can use it to develop API services with the help of some extensions.
Basic Usage
Pyramid's core concepts are Views and Routes. We need to define view functions to handle different HTTP requests and map them to corresponding routes.
from pyramid.view import view_config
@view_config(route_name='users', request_method='GET', renderer='json')
def get_users(request):
users = [...] # Get user list from database
return users
@view_config(route_name='users', request_method='POST', renderer='json')
def create_user(request):
user_data = request.json_body
# Process data and save to database
return {'status': 'success'}
config.add_route('users', '/users')
You can see that Pyramid's view functions need to be configured with decorators, specifying the request method, route name, etc. This approach is a bit more verbose compared to other frameworks.
However, Pyramid also provides some extensions to simplify the development of RESTful APIs.
Pyramid Extensions
The Pyramid community provides several extensions that can enhance the framework's API development capabilities.
Among them, the pyramid_jsonapi extension allows us to quickly generate RESTful APIs that conform to the JSONAPI specification, while pyramid_swagger can automatically generate OpenAPI documentation.
from pyramid_jsonapi import PyramidJSONAPI
api = PyramidJSONAPI(metadata, noop_models)
@api.view_config(collection_path='/users', context=User)
def user_list(context, request):
...
@api.view_config(collection_path='/users', request_method='POST',
context=User, permission='create')
def user_create(context, request):
...
You can see that after using the pyramid_jsonapi extension, the code structure becomes more concise and RESTful-style.
In addition to the above extensions, Pyramid has many other extensions, such as authentication and authorization (pyramid_jwt), database integration (pyramid_tm), and more, which can meet various needs for building production-level APIs.
Deployment Options
Pyramid applications can be deployed using WSGI servers like Gunicorn, uWSGI, etc. You can also choose to deploy the application to cloud platforms or Docker containers.
Overall, Pyramid is a highly flexible and extensible framework. Although its default design is not particularly well-suited for building RESTful APIs, we can make up for this shortcoming with the help of various extensions. If you need a highly customizable framework and don't mind introducing some third-party dependencies, Pyramid is a good choice.
Summary
We have briefly compared the features of Flask, Django REST Framework, FastAPI, Falcon, and Pyramid, popular Python web frameworks for building RESTful APIs.
Each framework has its own pros and cons, suitable for different scenarios. If you need to quickly develop a small API service, Flask and FastAPI are both good choices. For larger projects with more extensive API requirements, Django REST Framework might be more suitable. If you have extremely high performance requirements, Falcon is worth considering. And if you need a highly customizable and extensible solution, Pyramid is also a good choice.
Of course, in addition to the framework's features, you also need to consider factors such as the framework's ecosystem, community support, and personal preferences. Regardless, Python offers a wealth of choices in the web development domain, and you should be able to find the framework that best suits your needs.
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
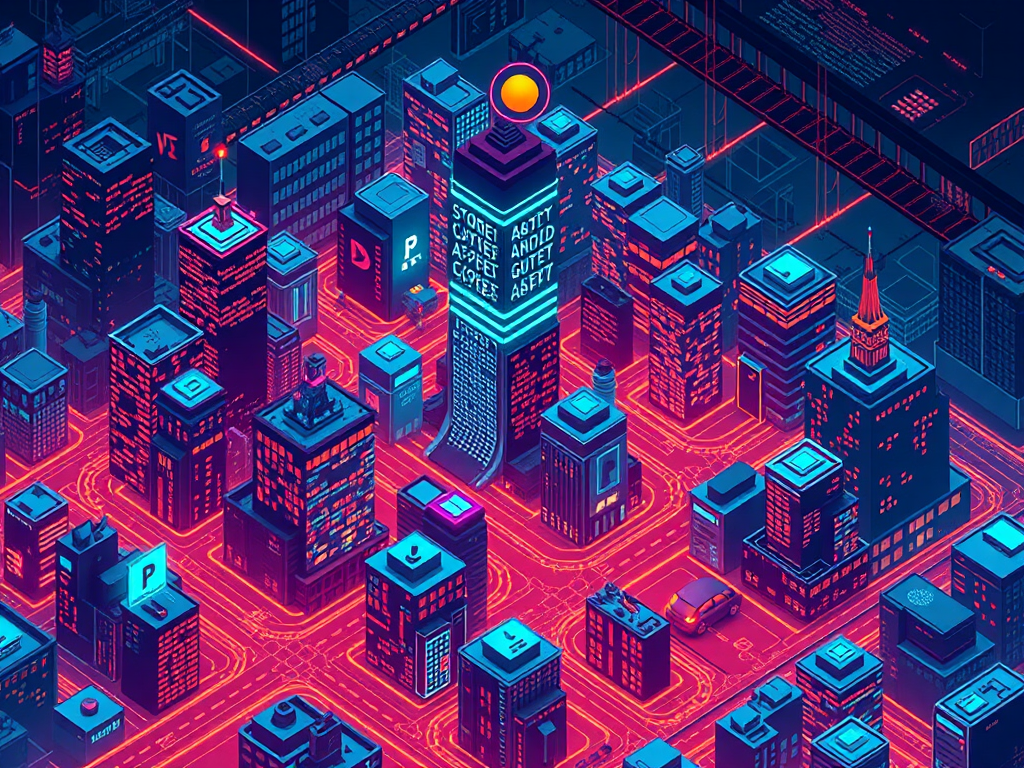
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
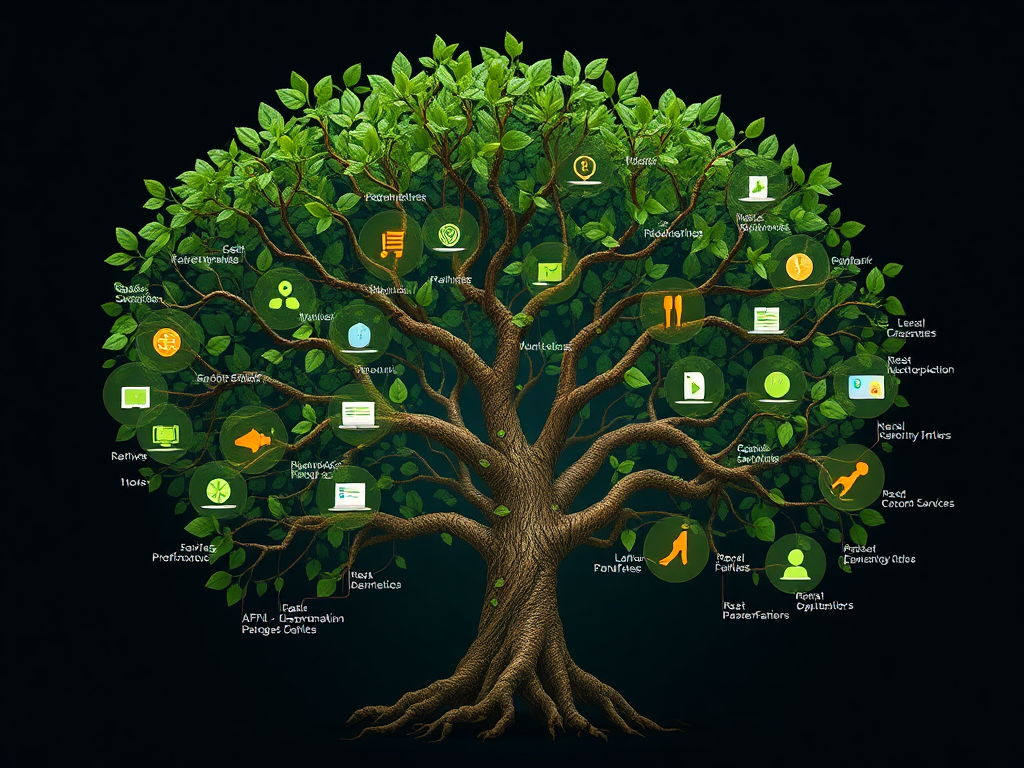
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
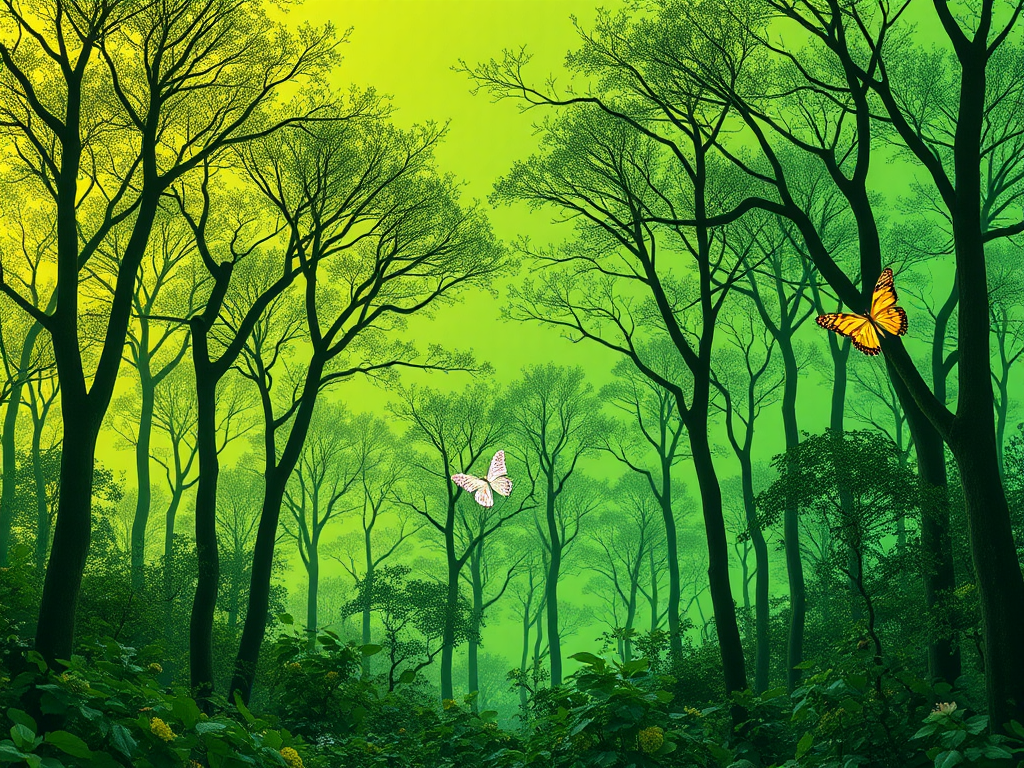
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services