Hi, friends! Have you been learning Python API development lately? As a seasoned Python programmer, I've stumbled along this path too. Today, let's explore some advanced techniques in API development and grow together!
Choosing the Right Web Framework
First, we need to choose a suitable web framework. For Python, there are many excellent options, such as Flask, Django, and FastAPI. Each framework has its strengths and is suitable for different scenarios.
Flask is lightweight and simple, perfect for rapid development of small APIs; Django is comprehensive, aimed at large projects; while FastAPI is a dark horse, with outstanding performance and efficient asynchronous capabilities. You can choose flexibly based on project requirements and personal preferences.
However, I personally prefer FastAPI. It's highly efficient for development, and the code is concise and readable. More importantly, FastAPI comes with built-in OpenAPI documentation support, which is very friendly for front-end and back-end separation projects.
Building RESTful Style APIs
Regardless of which framework we choose, we should strive to build APIs that conform to the RESTful style. As a mature software architecture style, RESTful helps us design standardized, unified, and easy-to-use interfaces.
For example, use standard HTTP methods to perform different operations on resources: GET to retrieve resources, POST to create new resources, PUT to update resources, DELETE to delete resources. Also, pay attention to URL naming, following RESTful conventions.
Here's an example code snippet of a RESTful API built using FastAPI:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
class Task(BaseModel):
title: str
done: bool = False
tasks = []
@app.get("/tasks")
def get_tasks():
return tasks
@app.post("/tasks")
def create_task(task: Task):
tasks.append(task.dict())
return task
@app.put("/tasks/{task_id}")
def update_task(task_id: int, task: Task):
if task_id >= len(tasks):
raise HTTPException(404, "Task not found")
tasks[task_id] = task.dict()
return task
@app.delete("/tasks/{task_id}")
def delete_task(task_id: int):
if task_id >= len(tasks):
raise HTTPException(404, "Task not found")
tasks.pop(task_id)
return {"message": "Task deleted"}
You see, through simple route declarations, we can easily implement CRUD operations on task resources. FastAPI has built-in request body parsing, exception handling, and other features, allowing us to focus on writing business logic.
Implementing API Authentication
When building production-grade APIs, we usually need to implement authentication functionality to ensure the interfaces are secure and reliable. Common authentication methods include Session authentication, Basic authentication, Token authentication, etc.
Among these, using JWT (JSON Web Token) for Token authentication is currently a popular solution. JWT is an open standard that enables stateless authentication, suitable for distributed systems and mobile scenarios.
We can quickly integrate JWT authentication using libraries like Flask-JWT-Extended. Here's a simple example:
from flask import Flask, jsonify, request
from flask_jwt_extended import JWTManager, jwt_required, create_access_token
app = Flask(__name__)
app.config['JWT_SECRET_KEY'] = 'super-secret'
jwt = JWTManager(app)
users = [
{"id": 1, "username": "alice", "password": "alice123"},
{"id": 2, "username": "bob", "password": "bob456"}
]
@app.route('/login', methods=['POST'])
def login():
username = request.json.get('username')
password = request.json.get('password')
user = next((user for user in users if user["username"] == username and user["password"] == password), None)
if user:
access_token = create_access_token(identity=user["username"])
return jsonify(access_token=access_token)
return jsonify({"msg": "Bad username or password"}), 401
@app.route('/protected', methods=['GET'])
@jwt_required()
def protected():
return jsonify({"msg": "This is a protected route!"})
With the @jwt_required()
decorator, we can require clients to provide a valid JWT when accessing protected interfaces. This way, even if the interface is maliciously accessed, sensitive data won't be leaked.
Of course, besides JWT, we can also consider using the OAuth 2.0 protocol to implement a more robust authentication and authorization mechanism.
Implementing API Version Control
As business requirements change and technology evolves, we may need to upgrade our APIs. This requires us to consider version control from the beginning of our design.
Common version control methods include: including version numbers in URL paths, specifying version numbers in HTTP headers, etc. The former is more intuitive and simple, but the latter is more in line with RESTful style.
Here's an example using FastAPI to include version numbers in URLs:
from fastapi import FastAPI
app = FastAPI()
@app.get("/v1/tasks")
def get_v1_tasks():
return [{"id": 1, "title": "Buy groceries"}]
@app.get("/v2/tasks")
def get_v2_tasks():
return [
{"id": 1, "title": "Buy groceries", "done": False},
{"id": 2, "title": "Walk the dog", "done": True}
]
Through this approach, we can smoothly transition to new versions of the API while ensuring backward compatibility. However, maintaining multiple versions of code can be costly in the long run, so we should also consider deprecating old versions at appropriate times.
Writing Test Cases
Reliable software requires comprehensive testing. When developing APIs, we should also write unit tests and integration test cases for key functionalities.
Unit tests can help us verify the correctness of individual functions or methods, while integration tests are used to verify the correctness of multiple modules working together. There are many choices for testing frameworks, such as unittest, pytest, robot framework, etc.
Here's a simple test case written using pytest for the Task API above:
import pytest
from fastapi.testclient import TestClient
from main import app
client = TestClient(app)
def test_get_tasks():
response = client.get("/tasks")
assert response.status_code == 200
assert response.json() == []
def test_create_task():
task = {"title": "Buy milk"}
response = client.post("/tasks", json=task)
assert response.status_code == 200
assert response.json() == task
def test_update_task():
# First create a task
task = {"title": "Buy bread"}
client.post("/tasks", json=task)
# Update the task
updated_task = {"title": "Buy eggs", "done": True}
response = client.put("/tasks/0", json=updated_task)
assert response.status_code == 200
assert response.json() == updated_task
Through test-driven development, we can not only improve code quality but also enhance our understanding of business requirements. It's an important means to ensure API correctness and maintainability.
Summary
Alright, that's all for sharing advanced techniques in Python API development. Let's review the key points:
- Choose the right web framework, weighing pros and cons based on project requirements
- Build API designs that conform to RESTful style
- Implement authentication mechanisms like JWT or OAuth 2.0
- Reserve design for version control to ensure backward compatibility
- Write unit tests and integration tests to ensure code quality
Of course, there are many other topics worth discussing in API development, such as performance optimization, cache application, monitoring and logging, etc. Let's continue to move forward together on this path and become better Python programmers!
Looking forward to sharing more exciting content with you in the next article!
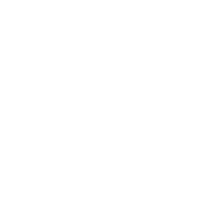
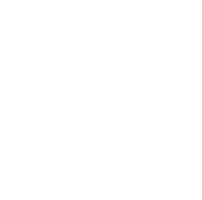