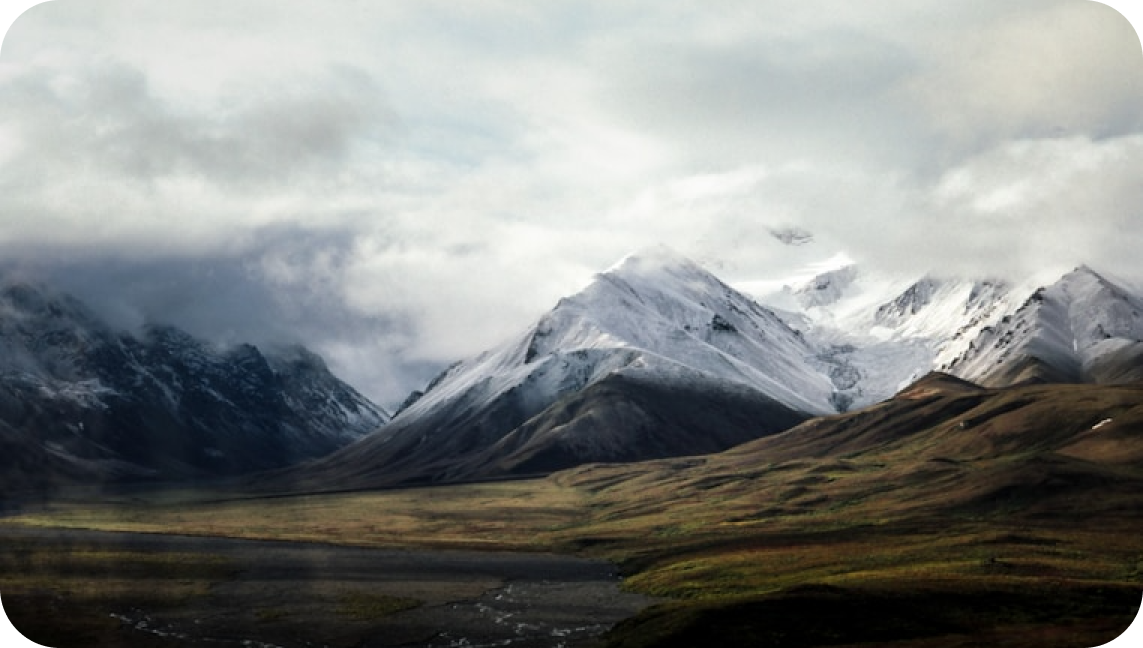
Hello everyone! Today we're going to talk about several commonly used frameworks for developing APIs in Python. As a Python blogger, I'm excited to share some practical technical knowledge with you all. Let's get started!
Quick Start with Flask
Flask is a lightweight Python Web framework, perfect for quickly building RESTful APIs. You only need a few simple lines of code to create a basic API. Let's see how:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
return jsonify({"message": "Hello, World!"})
if __name__ == '__main__':
app.run(debug=True)
Did you see that? We first created a Flask application, then defined a route handling function, using jsonify
to return JSON data. Run this code, visit http://127.0.0.1:5000/api/data
, and you'll see the JSON response returned by the API.
Isn't it super simple? However, if you want to build a more fully-featured API, Flask itself might be a bit underpowered. That's when you need to rely on some extensions.
Flask-RESTful Extension
Flask-RESTful is an extension for Flask that simplifies the process of creating APIs. With it, you can more conveniently define resources and routes, without having to write route functions one by one. Let's look at an example:
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
We first created a Resource
class, defining a get
method in it to handle GET requests. Then we used the Api
object to map this resource to the root path /
. Run the code, visit http://127.0.0.1:5000/
, and you'll see the JSON data returned by the API.
Personally, I find this approach with Flask-RESTful more intuitive and easier to manage. Especially for large projects, it can make your code more neat and orderly.
Advanced Features of Django REST
When it comes to Python Web frameworks, we certainly can't ignore Django. Django REST framework is a powerful tool for building APIs in the Django ecosystem. Compared to Flask, it provides more advanced features, such as powerful serialization functionality and authentication mechanisms.
Serializing Data
Django REST framework's serialization functionality is very powerful, easily converting complex data types (such as querysets and model instances) to JSON. Let's look at an example:
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
We defined a serializer class that inherits from ModelSerializer
. In the Meta
class, we specified the model and fields to be serialized. Then, we can use this serializer in the view:
from rest_framework.views import APIView
from rest_framework.response import Response
class MyModelView(APIView):
def get(self, request):
items = MyModel.objects.all()
serializer = MyModelSerializer(items, many=True)
return Response(serializer.data)
Look, we only need a few lines of code to convert a queryset to JSON data. Django REST framework handles all the details for us, greatly simplifying the development process.
JWT Authentication
In addition to serialization, Django REST framework also provides powerful authentication and permission control features. For example, we can use JWT (JSON Web Token) to implement stateless authentication.
First, we need to install the djangorestframework-simplejwt
library:
pip install djangorestframework-simplejwt
Then, enable JWT authentication in Django settings:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': (
'rest_framework_simplejwt.authentication.JWTAuthentication',
),
}
Finally, we create views for obtaining and refreshing JWT:
from rest_framework_simplejwt.views import TokenObtainPairView, TokenRefreshView
urlpatterns = [
path('api/token/', TokenObtainPairView.as_view(), name='token_obtain_pair'),
path('api/token/refresh/', TokenRefreshView.as_view(), name='token_refresh'),
]
With these settings, our API can now use JWT for authentication. Clients can obtain JWT by sending a username and password, and then carry JWT in subsequent requests for authentication.
Personally, I find these advanced features of Django REST framework very useful, as they can greatly improve our development efficiency. Of course, if your project is relatively simple, using Flask might also be a good choice.
FastAPI: The High-Performance Newcomer
Finally, I want to introduce an emerging Python Web framework - FastAPI. Compared to Flask and Django, FastAPI has better performance, and it also provides features like automatic documentation generation and better code completion.
Let's look at a simple example:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
After starting the service, visit http://127.0.0.1:8000/docs
, and you'll see the interactive API documentation automatically generated by FastAPI. Isn't that cool?
In addition to automatic documentation generation, FastAPI has another great feature - it leverages Python's type hints to provide better code completion and error checking. For large projects, this can greatly improve development efficiency and code quality.
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
price: float
@app.post("/items/")
def create_item(item: Item):
return item
In the code above, we defined an Item
model, using the Pydantic library to validate request data. FastAPI will automatically check if the request body conforms to the model definition, and throw an exception if it doesn't. This static type checking can detect errors at the coding stage, thus avoiding unexpected behavior at runtime.
Finally, FastAPI is based on Starlette, a high-performance asynchronous web server, so its performance is also top-notch. According to official benchmarks, FastAPI's performance is 300% to 500% higher than Flask, and 700% to 3000% higher than Django. This is undoubtedly a huge advantage for scenarios that need to handle a large number of requests.
Summing Up
Alright, that's all for today. We've looked at Flask, Flask-RESTful, Django REST framework, and FastAPI, each with its own characteristics and suitable for different scenarios.
If you just want to quickly build a small API, then Flask is definitely a good choice. It's simple to use and quick to get started with. If your project is larger and needs more advanced features, then Django REST framework would be a better choice, as it provides powerful serialization, authentication, and permission control features.
And if you're pursuing ultimate performance and want features like automatic documentation generation and better code completion, then FastAPI is definitely the trend of the future. It incorporates many excellent features of modern Python, and the development experience is also excellent.
No matter which framework you choose, I suggest you practice a lot. Theory is important, but it's only by encountering various problems in practice and solving them that your skills can truly improve. So, start coding right away!
If you encounter any problems during your learning process, feel free to ask me anytime. As an experienced Python blogger, I'll do my best to answer your questions. Let's go further together on the path of Python!
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
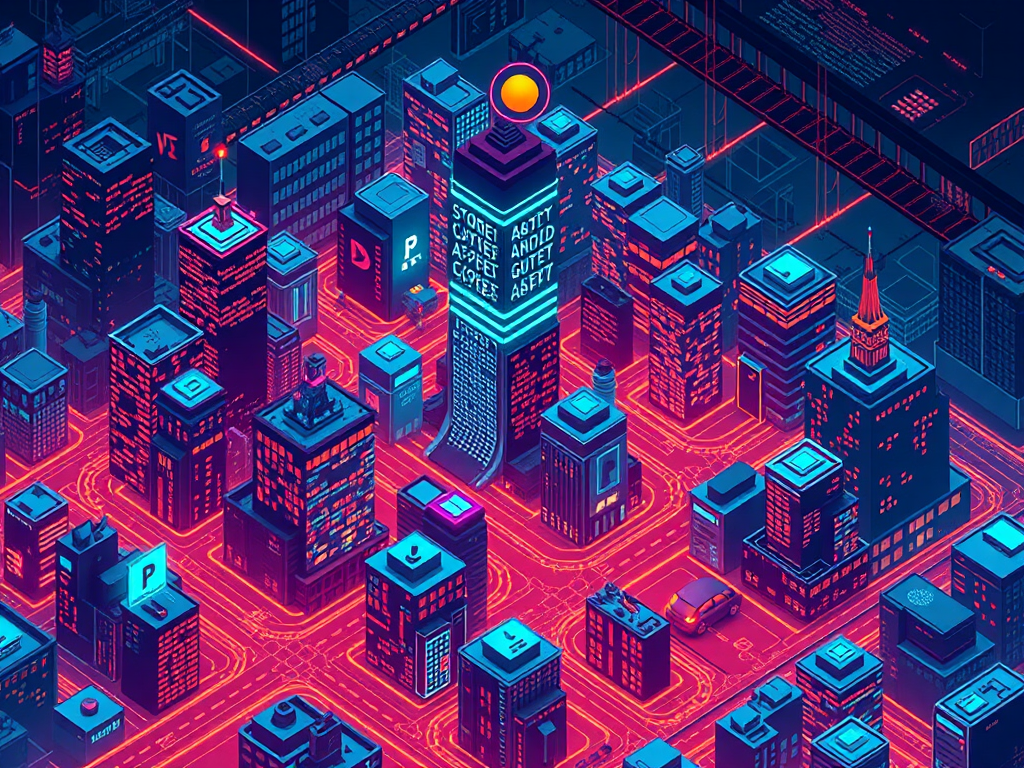
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
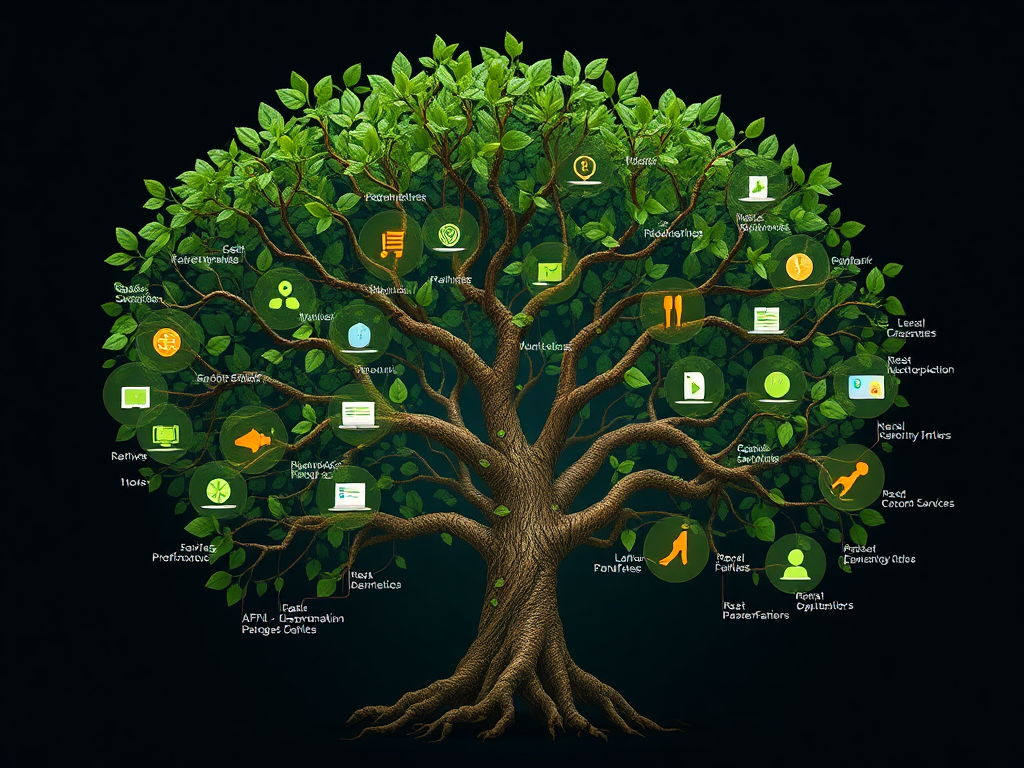
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
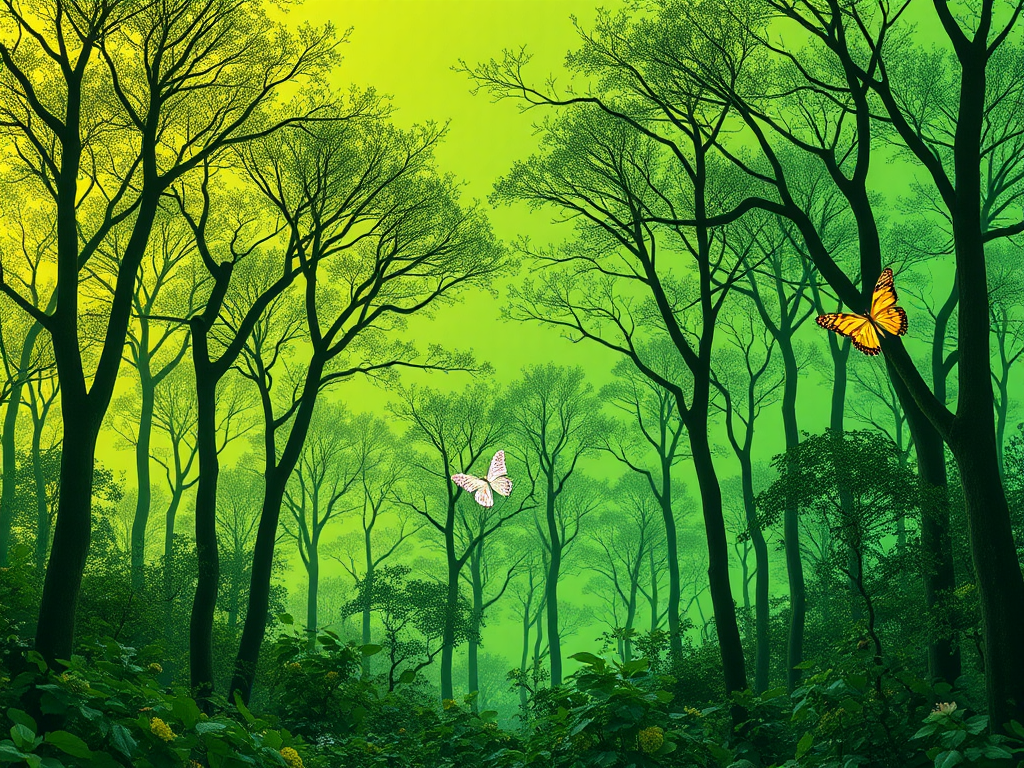
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services