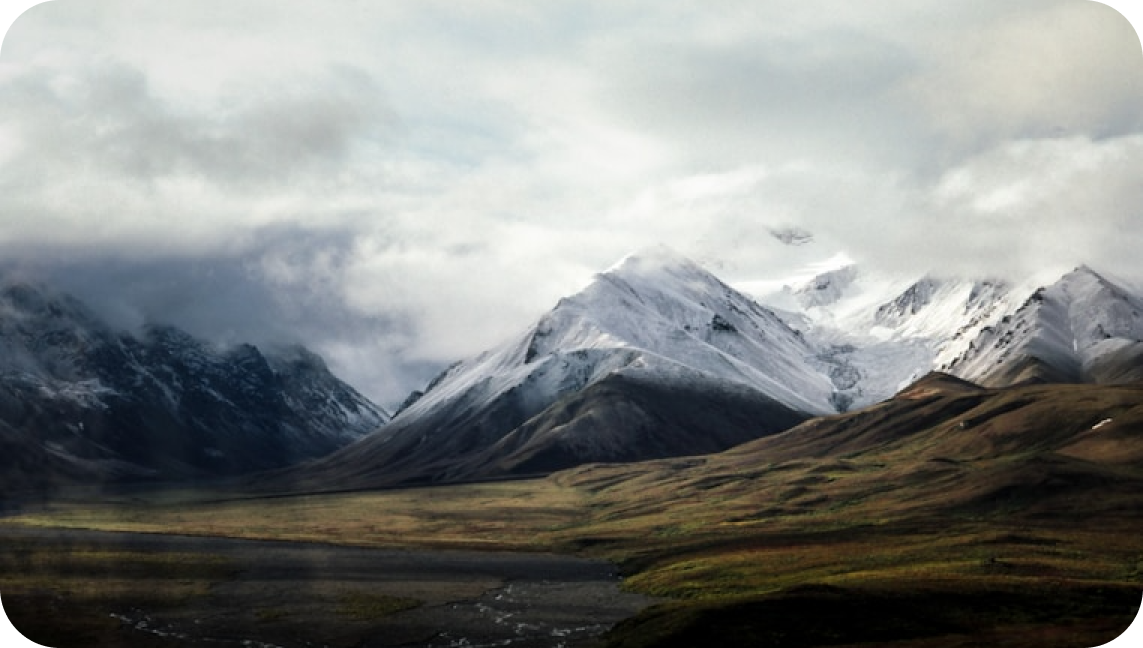
Choosing a Framework
While Flask is lightweight and flexible, it lacks strong support for large-scale API projects. Django REST framework is powerful but can be complex for beginners. FastAPI, as an emerging framework, combines the advantages of both and is a good choice.
FastAPI is based on ASGI (Asynchronous Server Gateway Interface) and achieves excellent performance through async/await syntax for asynchronous programming. It has native support for OpenAPI and JSON Schema, automatically generating interactive API documentation. It also provides advanced features like automatic data validation and request/response model definitions.
You can quickly install it with pip:
pip install fastapi uvicorn
uvicorn is a high-performance ASGI server used to host FastAPI applications.
Creating a minimal API only requires a few lines of code:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
Start the server:
uvicorn main:app --reload
Visit http://127.0.0.1:8000 and you'll see the JSON response. Simple, right?
Security Control
Any public-facing API needs authentication and authorization mechanisms to prevent unauthorized access. FastAPI can easily integrate third-party libraries to implement these features.
For example, using JWT (JSON Web Token), we can generate a token like this:
import jwt
from datetime import datetime, timedelta
SECRET_KEY = "09d25e094faa6ca2556c818166b7a9563b93f7099f6f0f4caa6cf63b88e8d3e7"
def create_token(data: dict, expires_delta: timedelta = None):
to_encode = data.copy()
if expires_delta:
expire = datetime.utcnow() + expires_delta
else:
expire = datetime.utcnow() + timedelta(minutes=15)
to_encode.update({"exp": expire})
encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm="HS256")
return encoded_jwt
The code to verify the token is as follows:
def verify_token(token: str):
try:
payload = jwt.decode(token, SECRET_KEY, algorithms=["HS256"])
return payload
except jwt.ExpiredSignatureError:
raise HTTPException(status_code=401, detail="Token expired")
except jwt.InvalidTokenError as e:
raise HTTPException(status_code=401, detail=str(e))
In the API view function, we can use HTTPBearer
to get the token from the request header and verify its validity:
from fastapi import Depends, HTTPException
import jwt
auth_scheme = HTTPBearer()
def verify_token(token: str = Depends(auth_scheme)):
try:
payload = jwt.decode(token.credentials, SECRET_KEY, algorithms=["HS256"])
return payload
except jwt.ExpiredSignatureError:
raise HTTPException(status_code=401, detail="Token expired")
except jwt.InvalidTokenError as e:
raise HTTPException(status_code=401, detail=str(e))
@app.get("/protected")
def protected_route(user=Depends(verify_token)):
return {"message": f"Hello {user['username']}!"}
This way, only authenticated requests can access protected routes. You can use other authentication schemes like OAuth2 as needed.
Error Handling
Graceful error handling is crucial for API usability. FastAPI provides built-in exception handling mechanisms, and we can customize exception responses.
First, we need to install a third-party library:
pip install python-http-client
Then create a custom exception handler:
from fastapi import FastAPI, Request, status
from fastapi.responses import JSONResponse
from pydantic import ValidationError
from http import HTTPStatus
app = FastAPI()
@app.exception_handler(ValidationError)
async def validation_exception_handler(request: Request, exc: ValidationError):
exc_str = f'{exc}'.replace('
', ' ').split(':
')[-1]
return JSONResponse(
status_code=status.HTTP_422_UNPROCESSABLE_ENTITY,
content={"detail": exc_str}
)
@app.exception_handler(HTTPException)
async def http_exception_handler(request, exc):
return JSONResponse(
status_code=exc.status_code,
content={"detail": exc.detail}
)
This handler catches ValidationError
and HTTPException
, and returns appropriate HTTP status codes and JSON error messages. You can add custom handlers for other exception types as well.
Additionally, logging exceptions is important for debugging and monitoring. FastAPI uses the logging
module by default, and we can configure log levels and handlers:
import logging
logging.basicConfig(level=logging.ERROR)
logging.getLogger().addHandler(logging.StreamHandler())
Through these mechanisms, we can provide friendly and consistent error responses for the API while ensuring exceptions are properly handled.
In conclusion, FastAPI combines simplicity, high performance, security, and maintainability, making it an excellent choice for building production-grade API services. Give it a try, and you'll surely be able to create beautiful APIs with it!
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
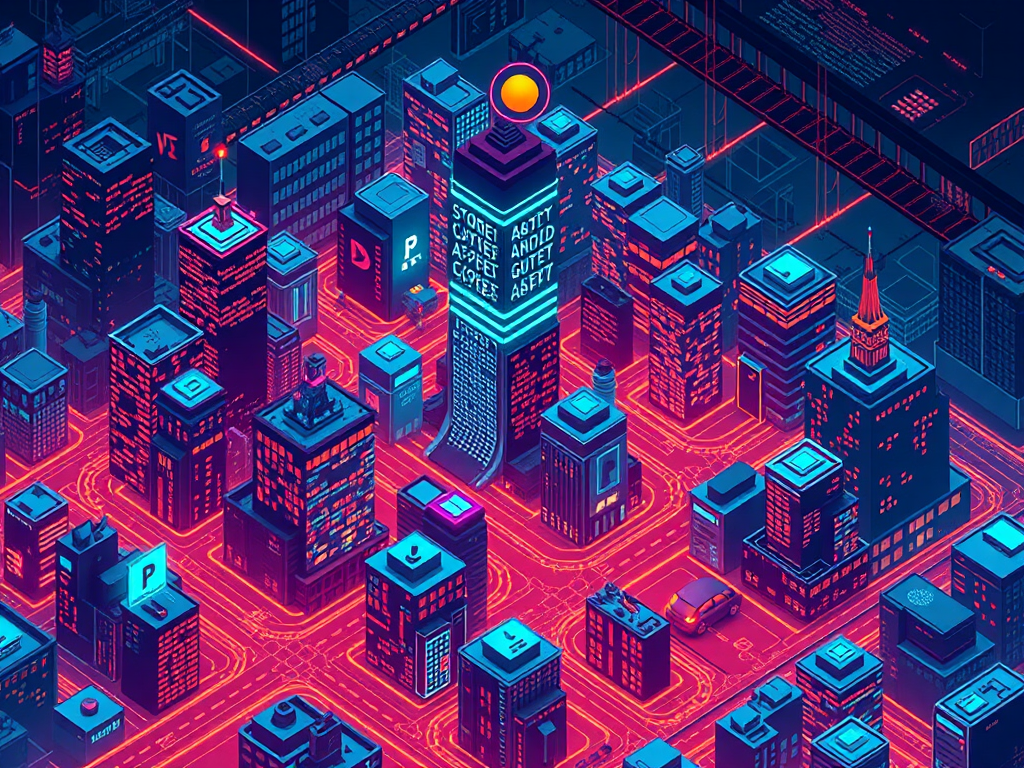
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
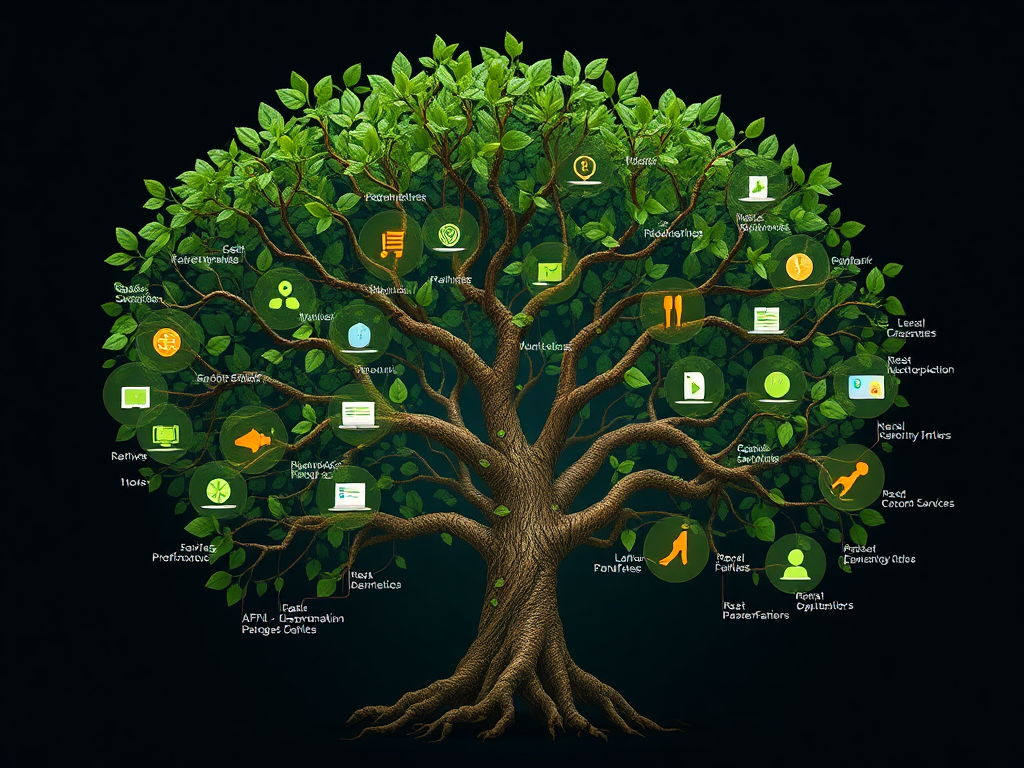
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
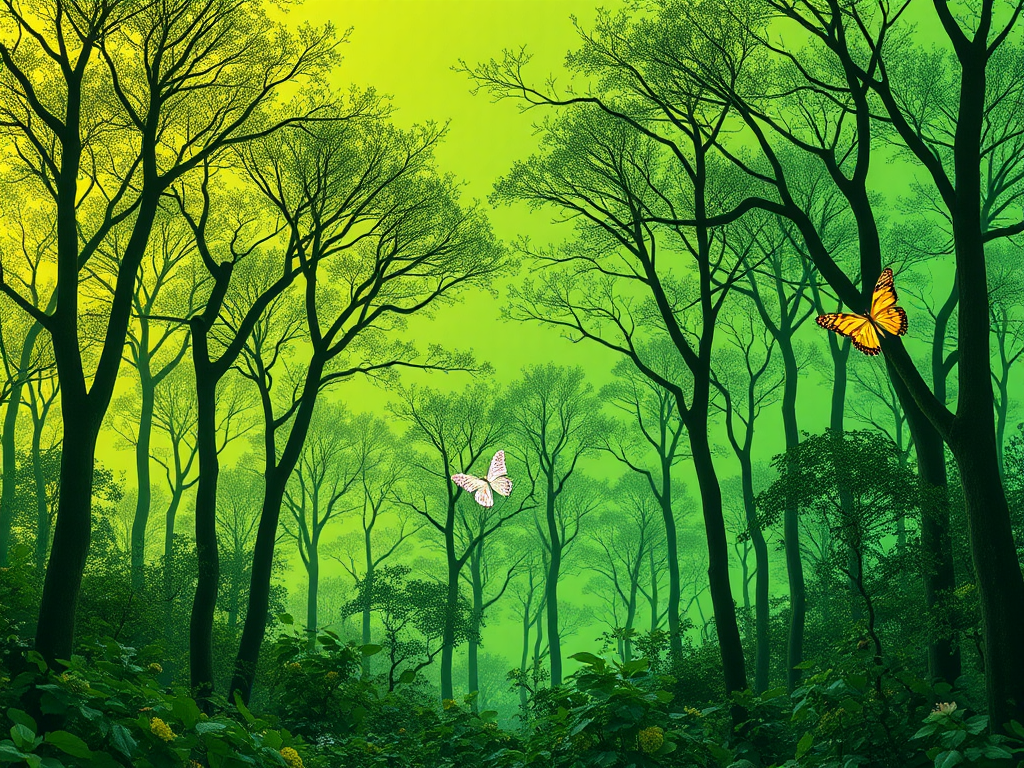
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services