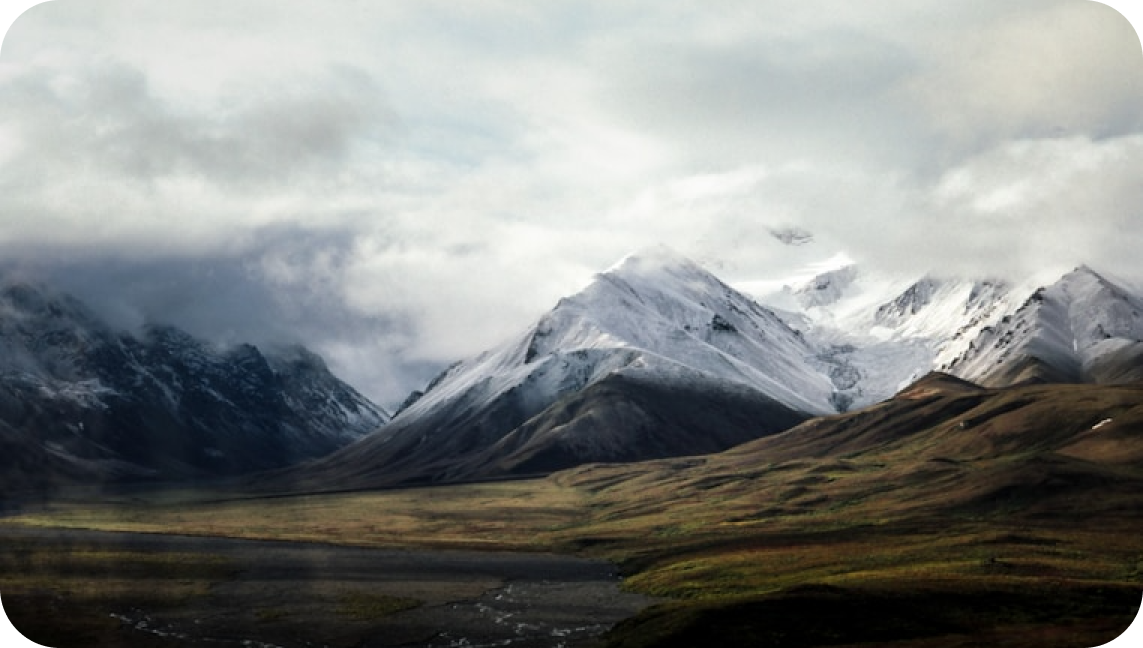
In Python API development, we often encounter IO-intensive tasks such as network requests and database queries. To improve program efficiency and response speed, asynchronous programming has emerged. Today, let's discuss how to elegantly use asynchronous programming in Python, as well as some common pitfalls and solutions.
Asynchronous Calls in Subthreads
In a multithreaded environment, you might encounter a scenario where you need to call an asynchronous function in a subthread and return the result to the main thread. Sounds a bit confusing? Don't worry, asyncio provides us with a very useful tool — asyncio.run_coroutine_threadsafe()
.
The purpose of this function is to safely call an asynchronous function in a subthread and return the result to the event loop of the main thread. You can use it like this:
import asyncio
async def my_async_func():
# Some asynchronous operations...
return result
def my_thread_func():
loop = asyncio.get_event_loop()
coro = my_async_func()
future = asyncio.run_coroutine_threadsafe(coro, loop)
result = future.result()
# Handle result in the main thread
Note that when using run_coroutine_threadsafe()
, you need to ensure that the main thread's event loop has started and pass it to this function. Otherwise, you might encounter some tricky errors.
To get the result of an asynchronous function, you can use the statement await asyncio.wrap_future(coro)
. It will wait for the asynchronous function to complete and return the result.
async def main():
coro = my_async_func()
wrapped = asyncio.wrap_future(coro)
result = await wrapped
# Handle result in the main thread
In this way, you can elegantly use asynchronous programming in a multithreaded environment. However, it's worth noting that too many threads and asynchronous operations may bring new problems, such as resource contention and deadlocks. So, it's still necessary to use these tools reasonably based on the actual situation.
Loading Large Models
Speaking of asynchronous programming, we have to mention a related topic — loading large models. When dealing with tasks like deep learning, we often need to load GB-level large models. If using traditional synchronous methods, it may cause the program to hang for a long time, affecting user experience.
Fortunately, ONNX Runtime provides us with a solution. It supports loading ONNX models larger than 2GB, but requires additional data to support the model size. You can use the onnxruntime.InferenceSession
class to load the model and pass the onnx_data
parameter to specify additional data.
import onnxruntime as rt
session = rt.InferenceSession("model.onnx", onnx_data="extra_data.bin")
inputs = {...}
outputs = session.run(None, inputs)
In this example, we loaded a model named model.onnx
using onnxruntime.InferenceSession
and passed extra_data.bin
as additional data. Note that the onnx_data
parameter must contain all the external data required by the model, otherwise it may cause loading failure or runtime errors.
In this way, we can load large models without affecting program response. Of course, if your model is relatively small, you can also consider using asynchronous loading to further improve efficiency.
New Ideas for Video Display
Processing video in Python is also a common task, such as displaying videos created by OpenCV in Google Colab. The traditional approach might be to save the video locally and then use some third-party libraries for display. But is there a more elegant way?
The answer is yes! In Colab, we can use the IPython.display.HTML()
function to display videos. First, we need to save the video locally, then use the open()
function to read the video file and convert it to HTML format. Finally, use the IPython.display.HTML()
function to display the video.
import cv2
from IPython.display import HTML
video = cv2.VideoWriter("output.mp4", ...)
video.release()
with open("output.mp4", "rb") as f:
video_data = f.read()
HTML(data="""
<video controls>
<source src="data:video/mp4;base64,{0}" type="video/mp4">
</video>
""".format(base64.b64encode(video_data).decode()))
In this way, we can directly display videos in Colab without relying on any third-party libraries. This method is not only concise and elegant but also avoids some potential compatibility issues.
Of course, if you need to display videos in a local environment, you can also consider using cv2.imshow()
or other third-party libraries like pygame
, matplotlib
, etc. The most important thing is to choose the right tool for different scenarios.
API Calls and Error Handling
When using third-party libraries and APIs, we often encounter various problems, such as version mismatches, API changes, etc. At this time, proper error handling becomes particularly important.
Taking the RAG model in the Hugging Face Transformers library as an example, if an error occurs, we can try the following methods:
- Check if the RAG model is correctly imported and ensure that the correct version is used.
- Ensure that the input data format and type are correct and meet the requirements of the model.
- If the problem still exists, try updating the Transformers library to the latest version, or check Hugging Face's documentation and sample code.
Similarly, when using the Roblox API, if you encounter API change issues, we can take the following steps:
- Check the Roblox developer documentation to understand the latest friend request API requirements and usage methods.
- Update the code according to the new API requirements and test to ensure it works properly.
- If it still can't be resolved, try seeking help on the Roblox developer forum.
Overall, when using third-party libraries and APIs, we need to pay attention to version updates and API changes at all times, and adjust the code promptly. At the same time, we should also pay attention to error handling, try to catch and handle potential exceptions as much as possible to ensure the stability and reliability of the program.
Summary
In this article, we discussed several common scenarios in Python API development, including asynchronous calls in subthreads, loading large models, video display, and API calls and error handling. Through these examples, we can see that asynchronous programming not only improves program efficiency and response speed but also helps us elegantly solve some tricky problems.
Of course, asynchronous programming is not omnipotent; it also has some pitfalls and limitations. For example, too many asynchronous operations may lead to resource contention and deadlock issues. Therefore, we need to choose synchronous or asynchronous programming modes reasonably according to specific scenarios, and pay attention to related best practices and pitfalls.
Finally, I want to emphasize one point: whether it's synchronous or asynchronous programming, good code style and error handling are very important. We should develop good coding habits, write code with good readability and maintainability, and focus on exception handling to ensure the stability and reliability of the program.
Alright, that's all I'll share about asynchronous programming in Python API development. If you have any questions or thoughts, feel free to discuss with me anytime. The road of programming is long and arduous, let's work hard together and continuously improve our skills and level!
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
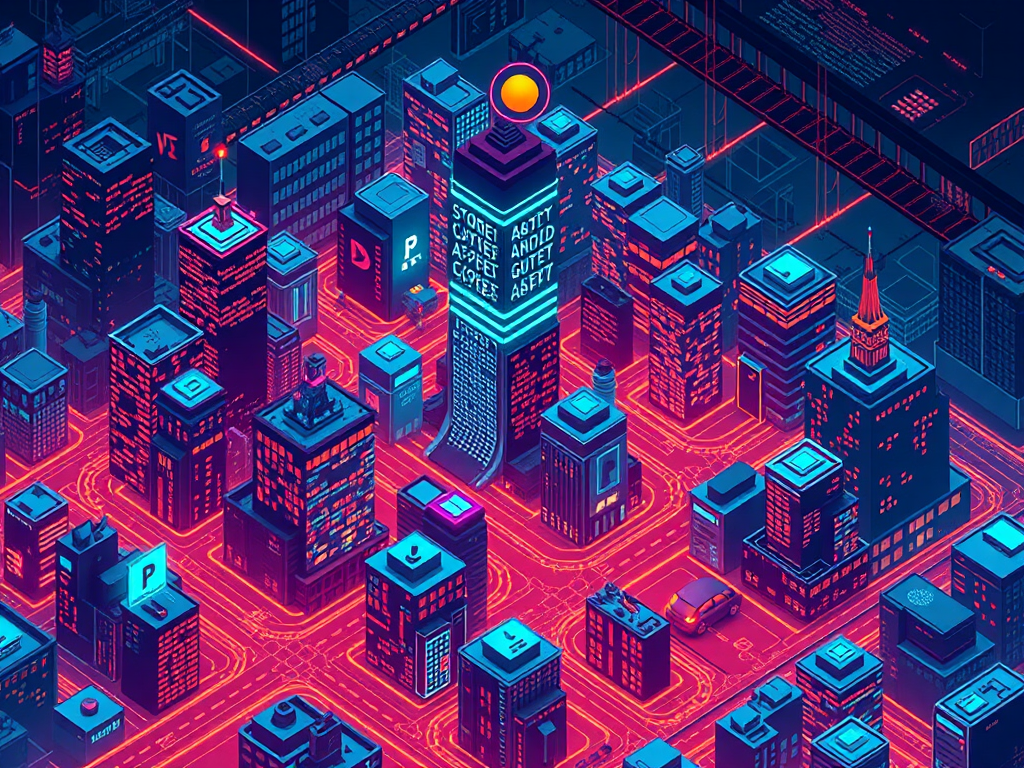
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
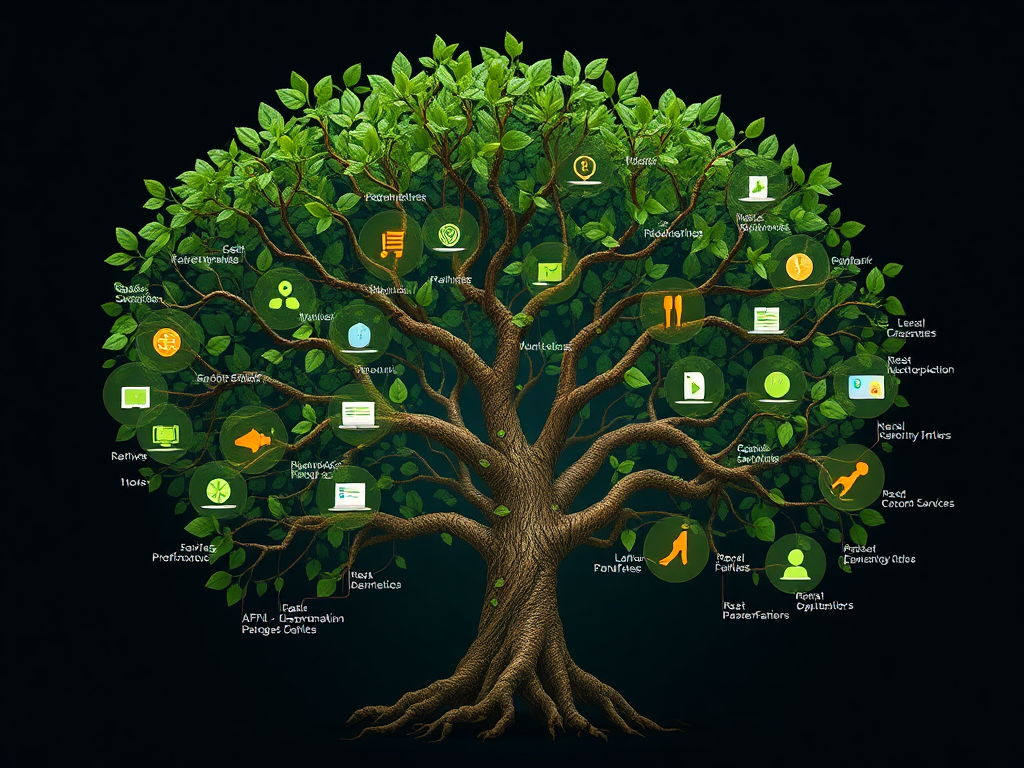
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
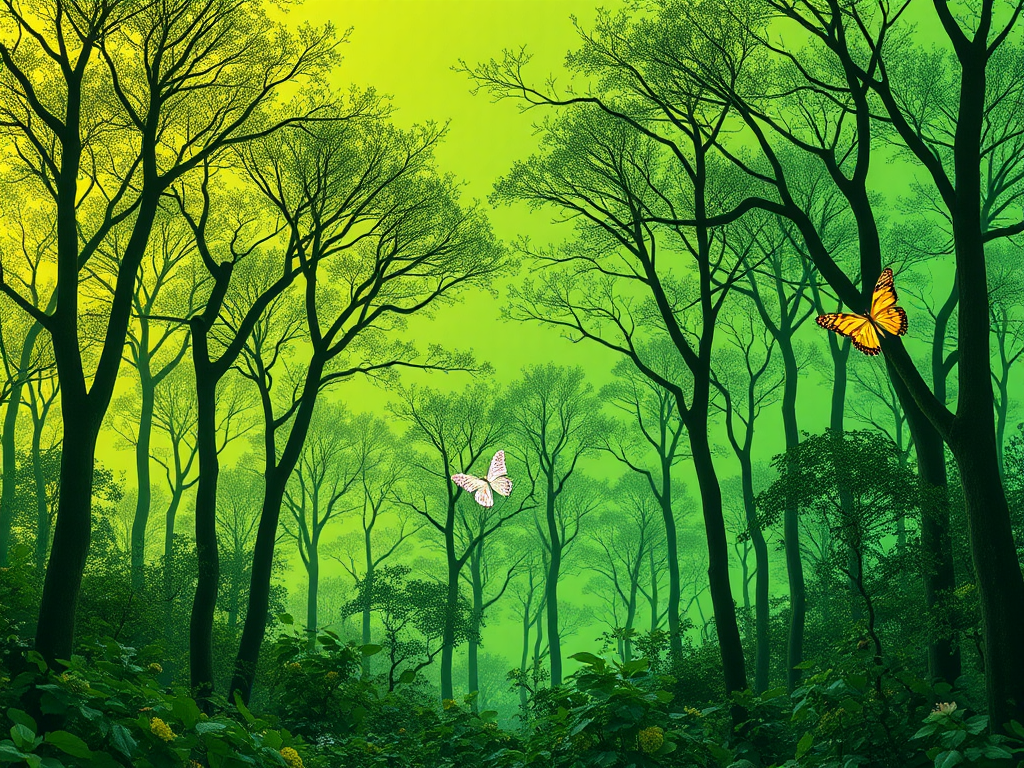
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services