Hey, Python enthusiasts! Today let's talk about the hot topic of Python API development. As a Python developer, have you ever been troubled by various issues in API development? Don't worry, today I'll guide you step by step to unveil the mysteries of Python API development, allowing you to easily master this essential skill.
Getting Started
First, let's start with the basics. Did you know that API development isn't as complicated as many people imagine? Once you master the right methods and tools, you can easily handle it.
So, where should we begin? That's right, with one of the most popular Web frameworks - Flask.
Flask
Flask is a lightweight Python Web application framework. It's designed to be extensible and easy to get started with. You might ask, why choose Flask instead of Django? Good question!
Flask's advantage lies in its simplicity and flexibility. For API development, we usually don't need the comprehensive features of Django. Flask allows us to quickly set up a basic API structure and then gradually add features as needed.
Let's look at how to start a Flask project:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
Looks simple, right? But when you start running this application, you might encounter a common error:
Error: failed to find flask application or factory in module 'app'
This error is usually caused by incorrect environment variable settings. Don't panic, I'll teach you how to solve it.
Environment Variables
In Flask development, setting environment variables correctly is very important. You need to set two key environment variables: FLASK_APP
and FLASK_ENV
.
Run the following commands in the terminal:
export FLASK_APP=app.py
export FLASK_ENV=development
Here, app.py
is the name of your main application file. Setting FLASK_ENV
to development
enables debug mode, which is very useful during development.
If you're using a Windows system, you need to use the set
command instead of export
:
set FLASK_APP=app.py
set FLASK_ENV=development
After setting the environment variables, you can use the flask run
command to start your application.
Application Structure
As your API becomes more complex, a good application structure becomes particularly important. I personally like to use the following structure:
myapp/
├── app/
│ ├── __init__.py
│ ├── models/
│ ├── routes/
│ ├── services/
│ └── utils/
├── config.py
├── requirements.txt
└── run.py
This structure separates different functional modules, making the code easier to maintain and expand. What do you think?
In the __init__.py
file, we can initialize the Flask application and other extensions:
from flask import Flask
from config import Config
def create_app():
app = Flask(__name__)
app.config.from_object(Config)
# Register blueprints and initialize extensions here
return app
This method is called the application factory pattern, which allows us to easily create multiple application instances, which is particularly useful in testing.
Testing
Speaking of testing, did you know? Testing is one of the most important parts of API development. Good tests can help you catch potential errors, improve code quality, and enhance your confidence in the code.
In Flask, we can use pytest
for testing. Here's a simple test example:
import pytest
from app import create_app
@pytest.fixture
def client():
app = create_app()
app.config['TESTING'] = True
with app.test_client() as client:
yield client
def test_hello_world(client):
response = client.get('/')
assert response.status_code == 200
assert b'Hello, World!' in response.data
This test ensures that our "Hello, World!" route works properly.
However, when running Django tests in PyCharm, you might encounter some issues. One of the most common problems is that DJANGO_SETTINGS_MODULE
is not set correctly.
Django Testing
Although we're mainly discussing Flask, Django is also a very popular Web framework, especially for large projects. When conducting Django tests in PyCharm, setting DJANGO_SETTINGS_MODULE
correctly is key.
You can set this environment variable in PyCharm's run configuration:
- Open the "Run/Debug Configurations" dialog
- Select your Django test configuration
- In the "Environment variables" section, add
DJANGO_SETTINGS_MODULE=myproject.settings
Here, myproject.settings
should be replaced with your actual settings module path.
After setting this up, you should be able to run Django tests smoothly. Remember, whether using Flask or Django, good test coverage is key to ensuring API quality.
Specific Scenarios
Now, let's look at some specific API development scenarios. These scenarios might seem special, but they actually represent many common problems.
OSC Communication
First, let's see how to send an OSC (Open Sound Control) bundle using Python. OSC is a communication protocol used for audio processing and other multimedia applications.
Using the python-osc
library, we can easily create and send OSC bundles:
from pythonosc import osc_bundle_builder
from pythonosc import osc_message_builder
from pythonosc import udp_client
client = udp_client.SimpleUDPClient("127.0.0.1", 12345)
bundle = osc_bundle_builder.OscBundleBuilder(
osc_bundle_builder.OscBundleTimestamp.IMMEDIATE)
msg = osc_message_builder.OscMessageBuilder(address="/example")
msg.add_arg("Hello, World!")
bundle.add_content(msg.build())
client.send(bundle.build())
This code creates an OSC bundle, adds a simple message, and then sends it to port 12345 on localhost.
You might ask, why use a bundle instead of sending messages directly? Good question! Bundles allow us to combine multiple messages, ensuring they arrive at the destination simultaneously. This is very useful in scenarios where multiple operations need to be synchronized.
PayPal Webhook
Another common API development scenario is integrating third-party services, such as PayPal. When using PayPal's webhook, verifying the signature is a key step.
Here's an example of how to verify webhook signatures using PayPal's Python SDK:
from paypalrestsdk import WebhookEvent
import paypalrestsdk
paypalrestsdk.configure({
"mode": "sandbox", # Use "live" for production environment
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET"
})
def verify_webhook_signature(transmission_id, timestamp, webhook_id, event_body):
try:
webhook_event = WebhookEvent.verify(
transmission_id=transmission_id,
timestamp=timestamp,
webhook_id=webhook_id,
event_body=event_body
)
return True
except Exception as e:
print(f"Webhook verification failed: {str(e)}")
return False
transmission_id = "your_transmission_id"
timestamp = "your_timestamp"
webhook_id = "your_webhook_id"
event_body = "your_event_body"
if verify_webhook_signature(transmission_id, timestamp, webhook_id, event_body):
print("Webhook verified successfully!")
else:
print("Webhook verification failed.")
This code demonstrates how to use PayPal's SDK to verify webhook signatures. Remember, when actually using it, you need to replace YOUR_CLIENT_ID
and YOUR_CLIENT_SECRET
with your actual PayPal API credentials.
Verifying webhook signatures is an important step to ensure that the webhook notifications you receive actually come from PayPal. This can prevent malicious attackers from forging webhook notifications.
Data Visualization
Finally, let's talk about data visualization. In API development, we often need to process and present large amounts of data. Matplotlib is one of the most popular data visualization libraries in Python.
Suppose you're developing an API that needs to generate a time series chart. However, you find that there are some empty dates on the x-axis, which affects the aesthetics of the chart. How to solve this problem?
import matplotlib.pyplot as plt
import pandas as pd
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
values = [i if i % 7 != 0 else None for i in range(len(dates))]
df = pd.DataFrame({'date': dates, 'value': values})
df_clean = df.dropna()
plt.figure(figsize=(12, 6))
plt.plot(df_clean['date'], df_clean['value'])
plt.xticks(rotation=45)
plt.title('Time Series Data')
plt.xlabel('Date')
plt.ylabel('Value')
plt.tight_layout()
plt.show()
This code first creates a time series data with some empty values. Then, we use the dropna()
method to remove all rows containing empty values. This way, our chart will only show dates with actual data.
You might ask, why not just skip empty values when plotting? That's a good question! In fact, Matplotlib automatically handles None
values when plotting, but by preprocessing the data, we can better control the appearance of the chart and easily perform further analysis on the cleaned data if needed.
Summary
Alright, we've covered a lot! From Flask's basic configuration, to Django's test setup, to specific API development scenarios like OSC communication and PayPal webhook integration, and finally discussing data visualization. These are all common challenges and solutions in Python API development.
Remember, API development is a process of continuous learning and improvement. Each project may bring new challenges, but also new learning opportunities. Keep your curiosity, constantly try new technologies and methods, and you'll find that API development is actually a very interesting and rewarding thing.
So, what interesting challenges have you encountered in API development? Do you have any unique solutions? Feel free to share your experiences and thoughts in the comments!
Finally, I want to say, don't be afraid of making mistakes. Every error is an opportunity to learn. Stay patient, stay passionate, and you will definitely become an excellent API developer. Keep going!
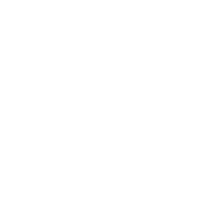
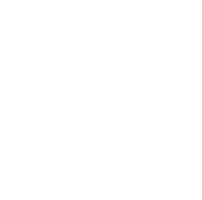