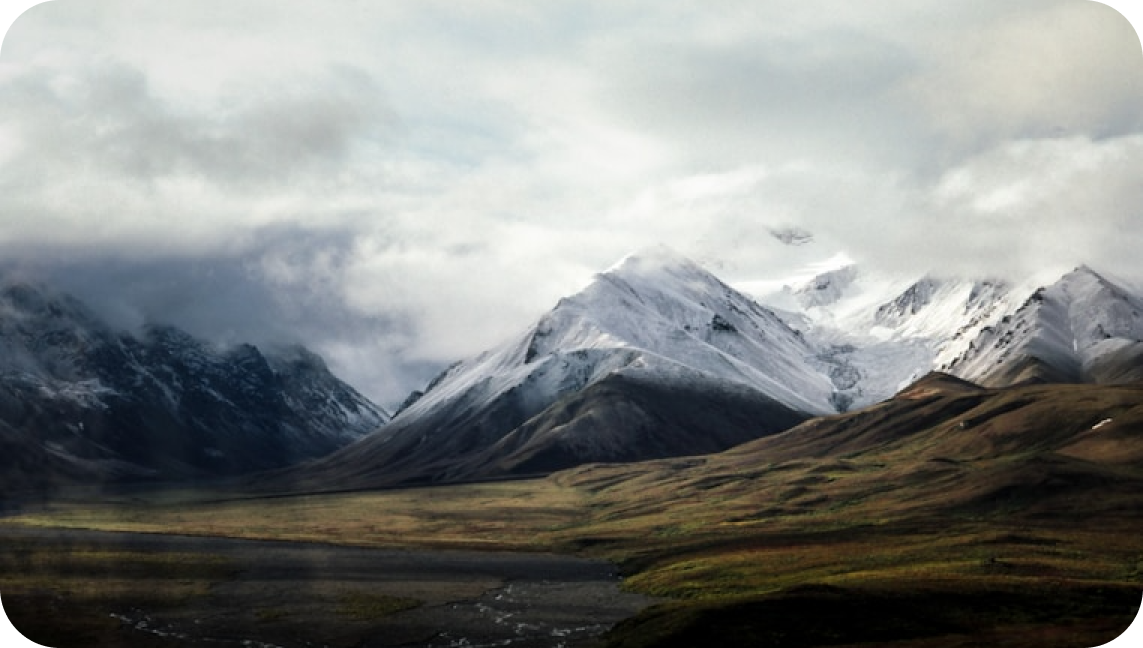
Introduction
Have you ever been confused by asynchronous programming in Python? Or puzzled by the concept of coroutines? Don't worry, today I'll explore Python coroutines and async programming with you in depth. Through years of teaching experience, I've found many students struggling with this topic. Let's unveil its mysteries step by step through some vivid examples.
Basic Concepts
Before diving deep, we need to understand several key concepts. Imagine you're at a restaurant, where waiters serve multiple tables without waiting for one table to finish before serving another - they serve other tables during waiting periods. This is the basic idea of asynchronicity.
In Python, coroutines are like these efficient waiters. They can switch to handling other tasks while waiting for one operation to complete. This mechanism is particularly suitable for I/O-intensive tasks, such as network requests and file operations.
Let's look at a simple example:
import asyncio
async def greet(name, delay):
await asyncio.sleep(delay)
print(f'Hello, {name}')
async def main():
await asyncio.gather(
greet('Alice', 1),
greet('Bob', 2),
greet('Charlie', 3)
)
asyncio.run(main())
Would you like me to explain or break down this code?
Deep Understanding
The working principle of coroutines is actually quite interesting. During teaching, many students ask: "Why do we use the keywords async and await?" That's a good question. async declares a function as asynchronous, while await indicates that we need to wait for an asynchronous operation to complete.
Let's understand through a more practical example. Suppose you're developing a network application that needs to handle multiple user requests simultaneously:
import asyncio
import aiohttp
import time
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.text()
async def process_urls(urls):
async with aiohttp.ClientSession() as session:
tasks = [fetch_data(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return results
async def main():
start_time = time.time()
urls = [
'http://api.example.com/data1',
'http://api.example.com/data2',
'http://api.example.com/data3'
]
results = await process_urls(urls)
end_time = time.time()
print(f'Total time: {end_time - start_time:.2f} seconds')
asyncio.run(main())
Would you like me to explain or break down this code?
Practical Applications
In real development, async programming has wide applications. I encountered such a situation in a project: needing to handle thousands of WebSocket connections simultaneously. Using synchronous methods would quickly exhaust server resources. But with coroutines, even a regular personal computer could easily handle tens of thousands of connections.
Let's look at an example of a WebSocket server:
import asyncio
import websockets
connected = set()
async def handle_connection(websocket, path):
connected.add(websocket)
try:
async for message in websocket:
for conn in connected:
if conn != websocket:
await conn.send(f"User said: {message}")
finally:
connected.remove(websocket)
async def main():
server = await websockets.serve(
handle_connection,
"localhost",
8765
)
await server.wait_closed()
asyncio.run(main())
Would you like me to explain or break down this code?
Performance Optimization
Speaking of async programming performance, there's an interesting phenomenon. I've found many developers marking all functions as async when first using coroutines. This is actually a misconception. Async programming is mainly for I/O-intensive tasks, not CPU-intensive tasks.
Let's look at a performance comparison example:
import asyncio
import time
from concurrent.futures import ProcessPoolExecutor
def cpu_bound_task(n):
return sum(i * i for i in range(n))
async def io_bound_task(n):
await asyncio.sleep(n) # Simulate I/O operation
return n
async def compare_performance():
# I/O-intensive tasks using coroutines
start = time.time()
tasks = [io_bound_task(i) for i in range(5)]
await asyncio.gather(*tasks)
io_time = time.time() - start
# CPU-intensive tasks using process pool
start = time.time()
with ProcessPoolExecutor() as executor:
numbers = [10**6] * 5
loop = asyncio.get_event_loop()
results = await loop.run_in_executor(
executor,
lambda: list(map(cpu_bound_task, numbers))
)
cpu_time = time.time() - start
print(f'I/O tasks time: {io_time:.2f} seconds')
print(f'CPU tasks time: {cpu_time:.2f} seconds')
asyncio.run(compare_performance())
Would you like me to explain or break down this code?
Best Practices
In practical development, I've summarized some best practices for async programming. These experiences were accumulated while handling real projects:
- Pay special attention to exception handling. Unhandled exceptions in async code can crash the entire application. Let's look at a more robust error handling example:
import asyncio
from typing import Optional
class AsyncRetry:
def __init__(self, retries: int = 3, delay: float = 1.0):
self.retries = retries
self.delay = delay
async def __call__(self, coro):
last_exception: Optional[Exception] = None
for attempt in range(self.retries):
try:
return await coro
except Exception as e:
last_exception = e
if attempt < self.retries - 1:
await asyncio.sleep(self.delay * (attempt + 1))
continue
raise last_exception
async def main():
retry = AsyncRetry()
@retry
async def unreliable_operation():
# Simulate potentially failing operation
import random
if random.random() < 0.7:
raise ConnectionError("Network error")
return "Success"
try:
result = await unreliable_operation
print(f"Operation result: {result}")
except Exception as e:
print(f"Final failure: {e}")
asyncio.run(main())
Would you like me to explain or break down this code?
Summary
Through this article, we've deeply explored coroutines and async programming in Python. From basic concepts to practical applications, from performance optimization to best practices, I believe you now have a deeper understanding of this topic.
Async programming is indeed a deep subject, and what we've discussed today might just be the tip of the iceberg. What part did you find most difficult to understand? Feel free to share your thoughts and experiences in the comments. If you want to learn more details, we can continue exploring in future articles.
Remember, choosing async programming isn't about following trends, but about making decisions based on actual scenarios. In I/O-intensive tasks, async programming can bring significant performance improvements; but for CPU-intensive tasks, multiprocessing might be a better choice.
Let's continue exploring and growing in the world of Python together. Do you have any thoughts to share?
Next
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services
Next
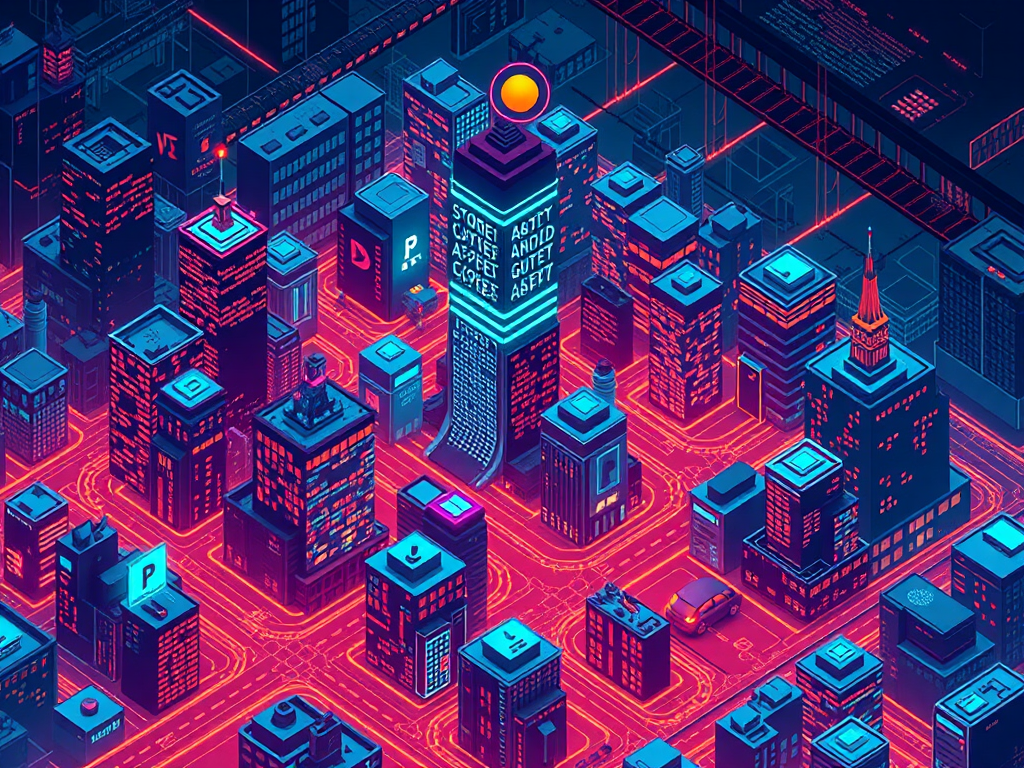
Advanced Python Data Structures: A Performance Optimization Journey from List Comprehension to Generator Expression
Comprehensive guide exploring Python programming features and its applications in web development, software development, and data science, combined with API development processes, best practices, and implementation guidelines, detailing the advantages and practical applications of Python in API development
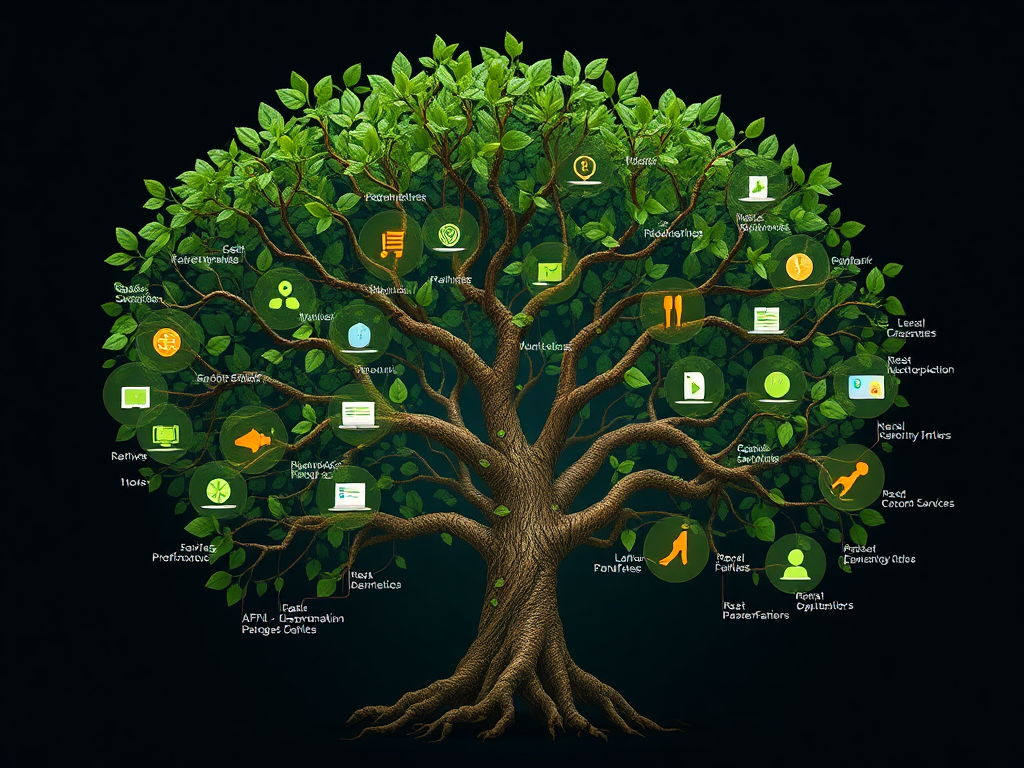
Unveiling Python API Development Frameworks
This article introduces several commonly used Python API development frameworks, including Flask, Flask-RESTful, Django REST framework, and FastAPI. It compares
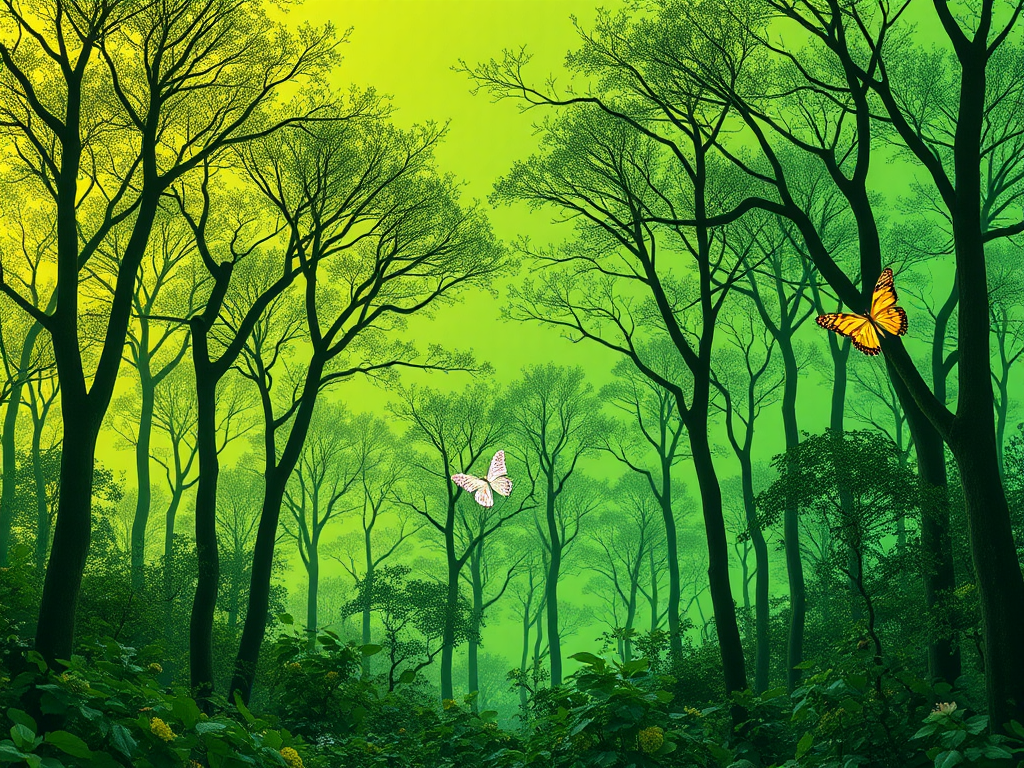
Essential Python API Development: Building Highly Available Interface Services in 6 Dimensions
A comprehensive guide to Python API development, covering RESTful frameworks, security mechanisms, quality assurance systems, and documentation testing to help developers build robust API services