Hey, Python enthusiasts! Today we're going to explore an exciting and challenging topic - Python API development. Are you eager to delve deep into this field? Then let's start this wonderful journey!
First Exploration
First, we need to understand what an API is. API stands for Application Programming Interface, and it's like a bridge for communication between different software. Imagine if there were no APIs, wouldn't software be like strangers who don't know each other? With APIs, they can happily communicate, collaborate, and complete various complex tasks together.
When it comes to Python API development, you might think of famous frameworks like Flask or Django. But today, I want to talk about a relatively new but very powerful framework - FastAPI.
One Step Ahead
FastAPI is really "fast" just like its name. It's not only fast in runtime but also in development efficiency. When I first used FastAPI, I was amazed by its simplicity and efficiency. Did you know that FastAPI claims its performance is comparable to NodeJS and Go? That's no small achievement!
However, like all technologies, you may encounter some challenges when developing APIs with FastAPI. Let's look at some common problems and how to solve them.
Pitfall Guide
Routing Maze
Sometimes, you might find that your carefully designed API always returns a 404 error. It's like you've drawn a treasure map but can't find the treasure yourself. Isn't that frustrating?
This problem is usually because the routes are not correctly registered. I remember once I spent an entire afternoon debugging, only to find out that I forgot to add the route in the FastAPI application. So, when you encounter this situation, first check if your routes are correctly registered.
For example, your code might look like this:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"message": "Hello World"}
Looks fine, right? But if your API still returns 404, it might be because you forgot to import this application in your run file. Make sure your main run file (like main.py
) has code similar to this:
from your_module import app
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
Database Connection Myth
If you encounter problems when using ORM tools like SQLModel, don't worry, you're not alone. Database connection issues are a nightmare for many developers, but they're actually not complicated to solve.
First, make sure your database URL is correct. A common mistake is forgetting to add sqlite:///
before the SQLite database URL. Second, check if you've correctly created the database session. A typical setup might look like this:
from sqlmodel import SQLModel, create_engine, Session
DATABASE_URL = "sqlite:///./test.db"
engine = create_engine(DATABASE_URL, echo=True)
def get_session():
with Session(engine) as session:
yield session
@app.on_event("startup")
def on_startup():
SQLModel.metadata.create_all(engine)
This code creates a database engine, defines a function to get a session, and creates all tables when the application starts.
Authentication Fog
OAuth2 authentication can be confusing, especially when you're trying to integrate third-party APIs. I remember the first time I tried to implement OAuth2, it felt like going in circles in a maze.
The key is to understand the OAuth2 process. Usually, it includes the following steps: 1. User clicks the "Login" button 2. User is redirected to a third-party service for authentication 3. User authorizes your application to access their data 4. The third-party service redirects the user back to your application and provides an authorization code 5. Your application uses this authorization code to obtain an access token 6. Your application uses the access token to call the API
In FastAPI, you can use the fastapi.security
module to simplify OAuth2 implementation. For example:
from fastapi.security import OAuth2AuthorizationCodeBearer
from fastapi import Depends, HTTPException, status
oauth2_scheme = OAuth2AuthorizationCodeBearer(
authorizationUrl="https://example.com/oauth/authorize",
tokenUrl="https://example.com/oauth/token",
)
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
user = get_current_user(token)
return user
This code sets up an OAuth2 authorization code flow and creates a dependency that can be used in routes that require authentication.
Open World
Speaking of APIs, we can't ignore those open APIs that have changed the world. For example, OpenAI's API allows us to easily integrate artificial intelligence into our applications. Or YouTube's API, which allows us to programmatically access the world's largest video platform.
AI Magic
Using OpenAI's API is like having an AI assistant. But be careful not to expose your API key! I once accidentally submitted my API key to GitHub and received a huge unexpected bill. Since then, I've learned to use environment variables to store sensitive information.
Here's a simple example of using the OpenAI API:
import os
import openai
openai.api_key = os.getenv("OPENAI_API_KEY")
response = openai.Completion.create(
engine="davinci",
prompt="Translate the following English text to French: '{}'",
max_tokens=60
)
print(response.choices[0].text.strip())
Remember, before using this code, you need to set the OPENAI_API_KEY
environment variable.
World of Video
The YouTube API opens up a world full of videos. But remember, when using this API, the search results may not always be consistent. This is because YouTube's search algorithm considers many factors, including the user's location, search history, etc.
Here's an example of searching for videos using the YouTube API:
from googleapiclient.discovery import build
youtube = build('youtube', 'v3', developerKey=API_KEY)
request = youtube.search().list(
part="snippet",
q="Python programming",
type="video",
maxResults=10
)
response = request.execute()
for item in response['items']:
print(item['snippet']['title'])
In this example, we searched for videos related to "Python programming" and printed the titles of the top 10 results.
Looking to the Future
API development is a constantly evolving field. With the rise of microservice architecture, the importance of APIs will only increase. As Python developers, we are fortunate to be at the forefront of this wave.
So, what should we learn next? I think GraphQL is a direction worth paying attention to. It provides a more flexible way to query and manipulate data. Or, you might want to delve into API security, learning how to protect your API from various attacks.
No matter which direction you choose, remember: learning never ends. Keep your curiosity and be brave to try new things, and you'll go far on the path of API development.
So, are you ready to take on the challenges of API development? Let's explore together in this world full of possibilities!
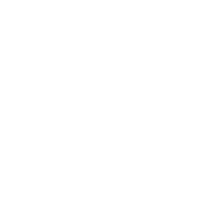
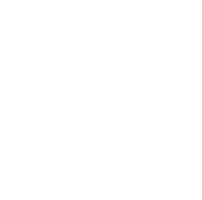
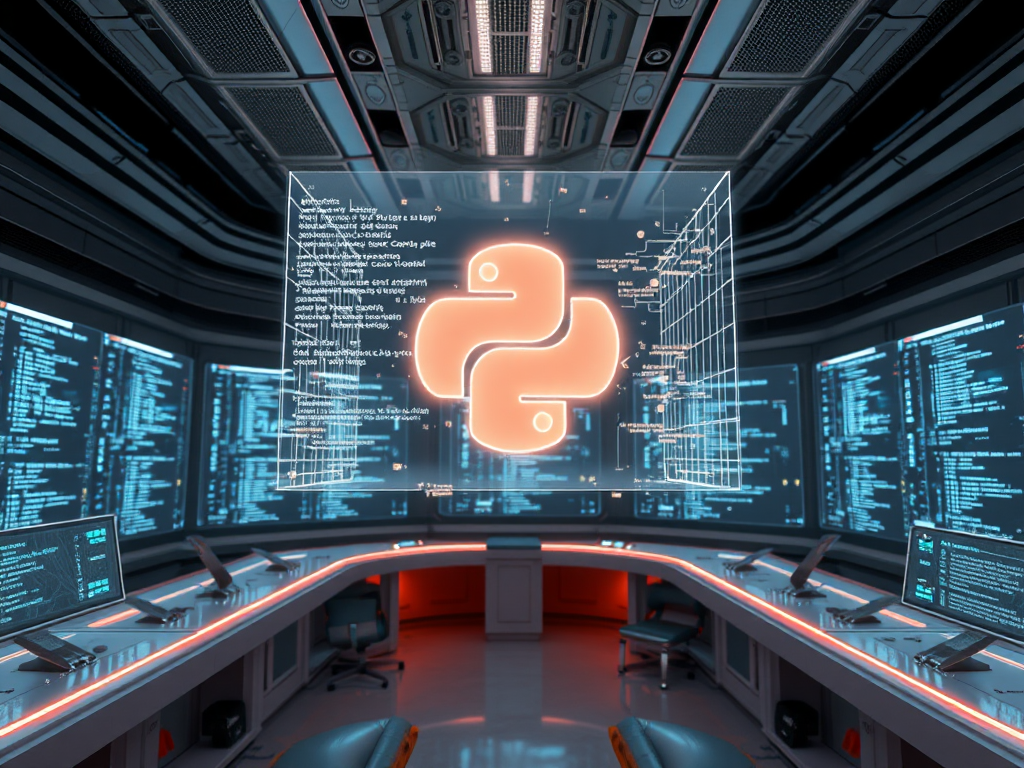
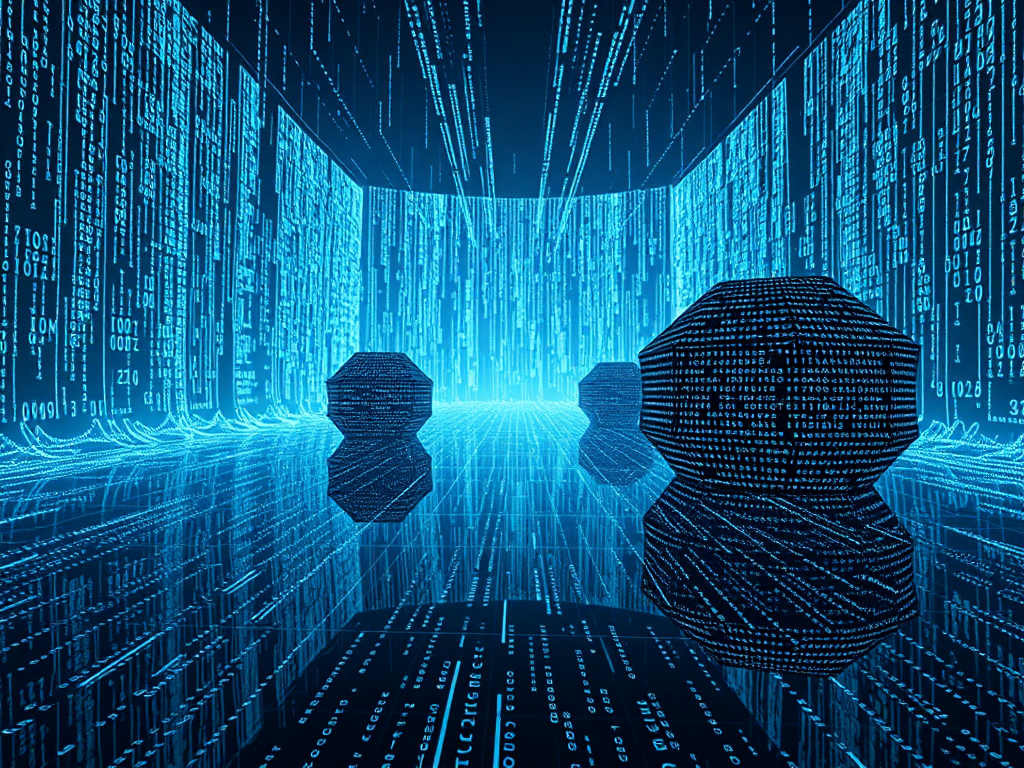
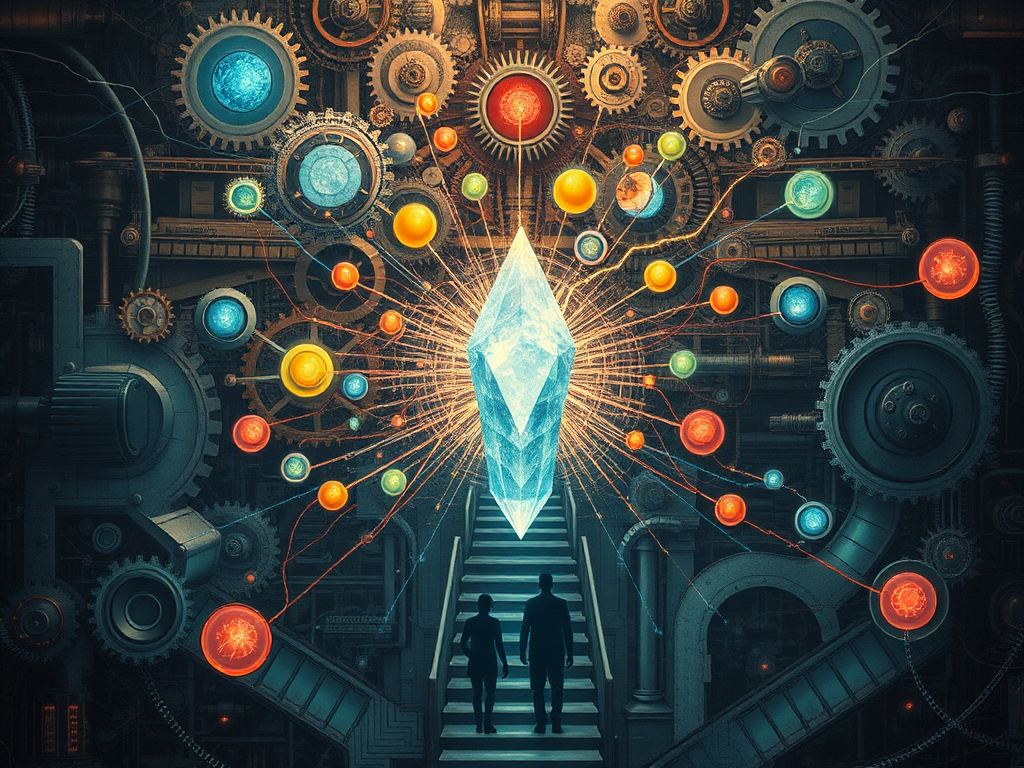