Origins
Have you often encountered such troubles? Django is too heavy, while Flask seems too lightweight. Sometimes we just need a web framework that is both simple and powerful, capable of quickly building high-performance APIs. Today I want to share with you the FastAPI framework, which makes Python API development unprecedentedly simple and efficient.
Features
I remember being amazed by its performance data when I first encountered FastAPI. Its speed can rival NodeJS and Go language projects, making it unique among Python web frameworks. Specifically, FastAPI is about 25% faster than Flask and about 40% faster than Django. This performance improvement is particularly noticeable when handling high concurrency requests.
FastAPI achieves such performance mainly due to its asynchronous features. It's built on Python 3.6+'s type hints and fully utilizes modern Python's new features. Did you know? FastAPI's founder Sebastián Ramírez developed FastAPI specifically to address the performance bottlenecks of traditional Python web frameworks.
Practice
Let's experience FastAPI's charm through a specific example. Suppose we want to develop a simple book management API:
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List, Optional
app = FastAPI()
class Book(BaseModel):
title: str
author: str
year: int
price: float
is_available: bool = True
books_db = []
@app.post("/books/", response_model=Book)
async def create_book(book: Book):
books_db.append(book)
return book
@app.get("/books/", response_model=List[Book])
async def read_books(skip: int = 0, limit: int = 10):
return books_db[skip : skip + limit]
@app.get("/books/{book_id}", response_model=Book)
async def read_book(book_id: int):
if book_id < 0 or book_id >= len(books_db):
raise HTTPException(status_code=404, detail="Book not found")
return books_db[book_id]
Would you like me to explain or break down this code?
Deep Dive
This code looks simple, but it demonstrates several important features of FastAPI. First, did you notice the type hints in the code? Like the response_model=Book
declaration. This not only provides better code hints but also automatically generates API documentation. I personally think this is one of FastAPI's most thoughtful features.
Looking at error handling, FastAPI provides a very elegant approach. Through HTTPException
, we can easily return appropriate HTTP status codes and error messages. This is much more concise than traditional try-except blocks.
Extension
FastAPI has a wide range of applications. In projects I've been involved with, some used it to build microservice architectures, some to develop machine learning APIs, and even some to build real-time data analysis platforms. Let me share a more complex example showing how to use FastAPI to build an API with database operations:
from fastapi import FastAPI, Depends, HTTPException
from sqlalchemy import create_engine, Column, Integer, String, Float, Boolean
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker, Session
from typing import List
from pydantic import BaseModel
SQLALCHEMY_DATABASE_URL = "sqlite:///./books.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
class BookDB(Base):
__tablename__ = "books"
id = Column(Integer, primary_key=True, index=True)
title = Column(String, index=True)
author = Column(String, index=True)
year = Column(Integer)
price = Column(Float)
is_available = Column(Boolean, default=True)
Base.metadata.create_all(bind=engine)
class BookSchema(BaseModel):
title: str
author: str
year: int
price: float
is_available: bool = True
class Config:
orm_mode = True
app = FastAPI()
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
@app.post("/books/", response_model=BookSchema)
async def create_book(book: BookSchema, db: Session = Depends(get_db)):
db_book = BookDB(**book.dict())
db.add(db_book)
db.commit()
db.refresh(db_book)
return db_book
@app.get("/books/", response_model=List[BookSchema])
async def read_books(skip: int = 0, limit: int = 10, db: Session = Depends(get_db)):
books = db.query(BookDB).offset(skip).limit(limit).all()
return books
Would you like me to explain or break down this code?
Advantages
Speaking of FastAPI's advantages, I think the most noteworthy is its automatic documentation generation feature. You just need to visit the /docs
path to see beautiful Swagger UI documentation, which is very helpful for API debugging and team collaboration. I remember in one project, the product manager was thrilled to see this documentation because he could test API functionality directly in the browser.
Additionally, FastAPI's dependency injection system is very powerful. Through Depends
, we can easily implement authentication, database session management, and other features. This design makes the code easier to test and maintain.
Ecosystem
FastAPI's ecosystem is rapidly developing. According to Python Package Index (PyPI) statistics, FastAPI's monthly downloads have exceeded 5 million, and this number continues to grow. More and more companies are starting to use FastAPI in production environments, including tech giants like Microsoft and Uber.
Practical Application
In actual development, I suggest you pay special attention to the following points:
-
Use asynchronous features appropriately. Not all operations need to be asynchronous; decide based on the actual scenario.
-
Pay attention to API version control. This can be implemented through path prefixes, for example:
from fastapi import APIRouter
router_v1 = APIRouter(prefix="/v1")
router_v2 = APIRouter(prefix="/v2")
@router_v1.get("/users")
async def get_users_v1():
return {"version": "1.0", "data": "users"}
@router_v2.get("/users")
async def get_users_v2():
return {"version": "2.0", "data": "users"}
Would you like me to explain or break down this code?
Future
Looking ahead, I believe FastAPI will continue to strengthen in several areas:
First is enhanced WebSocket support. Currently, FastAPI's WebSocket functionality is already powerful, but there's room for improvement. Particularly in real-time data transmission and bidirectional communication, there will likely be more optimizations.
Second is integration with other modern technologies. For example, deep integration with GraphQL will provide more possibilities for API development. According to discussions on GitHub, FastAPI's next major version might bring these improvements.
Recommendations
Finally, I want to give some advice to those planning to use FastAPI:
-
Value type hints. They not only provide a better development experience but also help you avoid many potential errors.
-
Organize project structure properly. As project scale increases, good structure becomes increasingly important. I suggest referring to this directory structure:
project/
├── app/
│ ├── __init__.py
│ ├── main.py
│ ├── dependencies.py
│ ├── routers/
│ │ ├── __init__.py
│ │ ├── users.py
│ │ └── items.py
│ └── models/
│ ├── __init__.py
│ └── user.py
├── tests/
│ ├── __init__.py
│ ├── test_main.py
│ └── test_users.py
└── requirements.txt
- Make good use of FastAPI's built-in features. Such as parameter validation, dependency injection, etc., these are well-designed and can greatly improve development efficiency.
Conclusion
FastAPI is indeed a game-changing framework. It maintains Python's concise elegance while bringing the high performance and type safety needed for modern web development. What do you think? Feel free to share your experiences and thoughts using FastAPI in the comments section.
If you want to learn FastAPI in depth, I suggest starting with the official documentation and then gradually trying more complex projects. Remember, the best way to learn is through hands-on practice. Let's explore more possibilities together in the world of FastAPI.
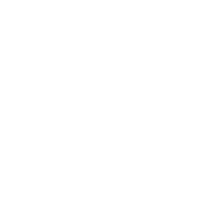
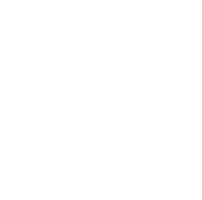