Have you ever wondered where the real-time data comes from when you check the weather forecast on your phone app? Or how product information loads so quickly when you shop online? Behind these seemingly simple operations, there's a powerful "hero behind the scenes"—the API. Today, let's delve into the world of Python API development and see how to utilize this powerful tool to create amazing applications.
Unveiling the API
An API, or Application Programming Interface, is the bridge of communication between different software applications. Imagine if software is a city, then the API is the road connecting different buildings. Through these "roads," data can flow freely, enabling various applications to work together and create more powerful and useful functions.
For example, when you use a weather app, the app itself doesn't generate weather data. Instead, it gets the latest weather information by calling the API provided by meteorological services. This is the charm of APIs—it allows different systems to exchange information seamlessly.
So, what does a typical API request look like? Let's take a look:
- URL: This is the API's address, telling you where to find the information you need.
- HTTP Method: Like GET (to retrieve data) or POST (to send data), it's like ringing a doorbell or knocking on the door at the address.
- Request Parameters: The specific information you want, like telling the host your purpose for visiting.
- Response Data: The result returned by the API, usually in JSON format, like the response from the host.
Isn't it interesting? Next, let's see how to develop and use APIs in Python.
Flask: A Lightweight API Development Tool
When it comes to API development in Python, you can't miss the Flask framework. Flask is like a magical toolbox, containing various tools to help us quickly build APIs. Let's look at a simple example:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def hello():
return 'Hello, API World!'
@app.route('/v1/task', methods=['GET', 'POST'])
def do_task():
if request.method == "GET":
content = request.args.get("content")
res = int(content) + 10
elif request.method == "POST":
input_dict = request.get_json()
res = input_dict.get('content') + 12
return jsonify({"code": 0, "msg": "success", "data": res})
if __name__ == '__main__':
app.run("0.0.0.0", debug=True, port=6006)
Looks magical, right? Let me explain this code:
- We created two routes:
/hello
and/v1/task
. - The
/hello
route is simple, saying "Hello, API World!" when accessed. - The
/v1/task
route is more interesting. It can handle GET and POST requests and returns different results based on the request method.
See, with just a few lines of code, we created a fully functional API. Isn't Flask powerful?
The Art of Requesting
Now that we've created an API, how do we use it? This brings us to another magic tool in Python—the Requests library. Using Requests to send HTTP requests is as simple as shopping online. Let's take a look:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
data = response.json()
print(data)
url = 'http://127.0.0.1:6006/v1/task'
headers = {'Content-Type': 'application/json'}
data = json.dumps({'content': 1})
response = requests.post(url, data=data, headers=headers)
print(response.text)
See, it's that simple! We can easily send GET and POST requests, just like browsing and submitting forms online.
The Magic of Data
In real projects, we often need to store data obtained from APIs for later use. SQLite is a lightweight database, perfect for this scenario. Let's see how to store API data in an SQLite database:
import sqlite3
conn = sqlite3.connect('items.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS items
(id TEXT PRIMARY KEY, title TEXT, price REAL)''')
def save_item_to_db(item):
c.execute("INSERT OR REPLACE INTO items (id, title, price) VALUES (?, ?, ?)",
(item['numiid'], item['title'], item['price']))
conn.commit()
def get_item_details(item_id):
# Here should be the code to call the API to get item details
# For demonstration, we directly return fake data
return {'numiid': item_id, 'title': f'Item {item_id}', 'price': 99.9}
item_ids = ['652874751412', '520813250866']
for item_id in item_ids:
item = get_item_details(item_id)
save_item_to_db(item)
conn.close()
This code shows how to store data retrieved from an API into an SQLite database. We created a simple table to store item information and used a function to insert or update data in the database.
Advanced Techniques
In real development, we also need to pay attention to some details to improve code efficiency and robustness:
- Using list comprehensions can greatly simplify code. For example:
python
prices = [item['price'] for item in items]
This line of code can extract all prices from a list of items.
- Exception handling is key to ensuring code robustness. For example:
python
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as e:
print(f"HTTP Error: {e}")
This code can catch errors that may occur in HTTP requests and handle them appropriately.
Conclusion
Today, we learned the basics of Python API development, from the concept of APIs to creating APIs with Flask, sending requests using the Requests library, and finally exploring how to store data in an SQLite database. This knowledge opens a door to a broader programming world for you.
Remember, API development is not just about writing code; it's an art of connecting different systems and creating new value. Once you master these skills, you can build more powerful and useful applications.
So, are you ready to start your API development journey? Let's explore this world full of endless possibilities together!
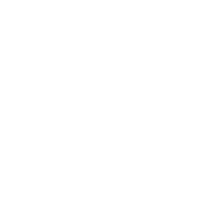
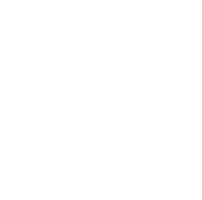
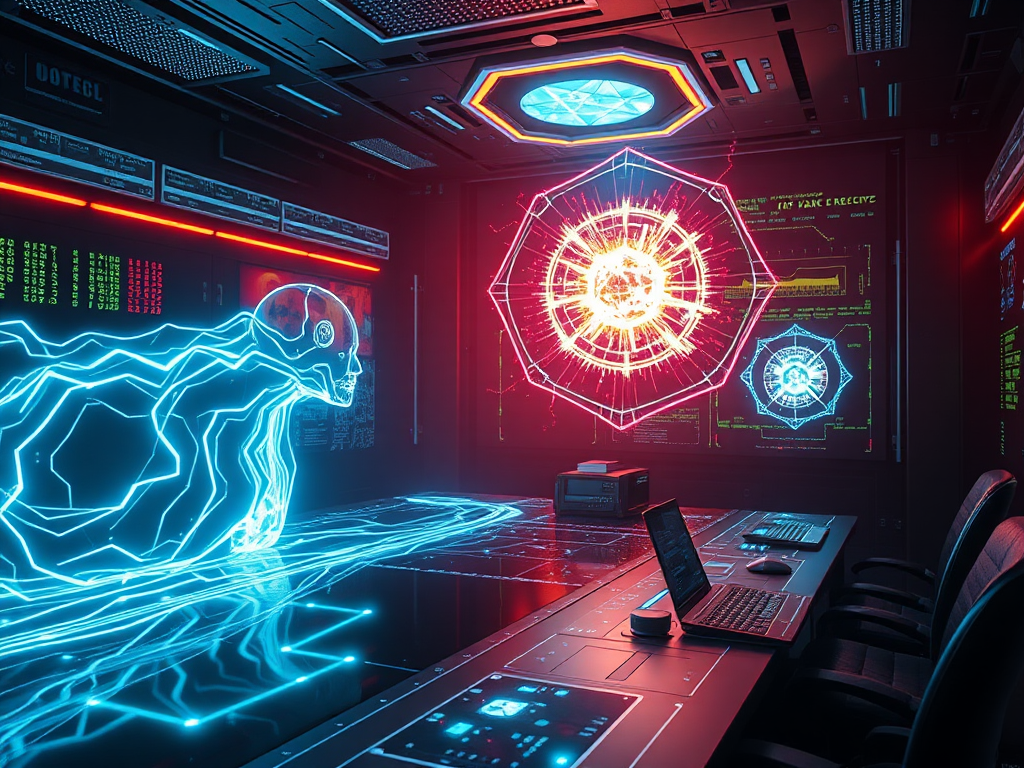
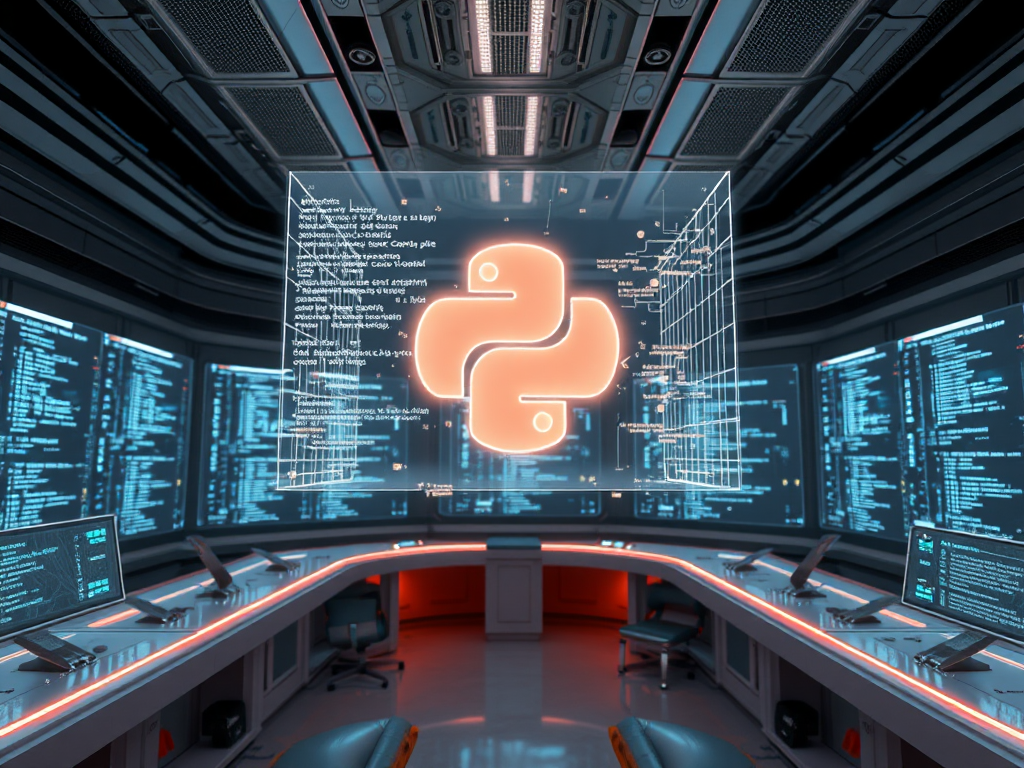
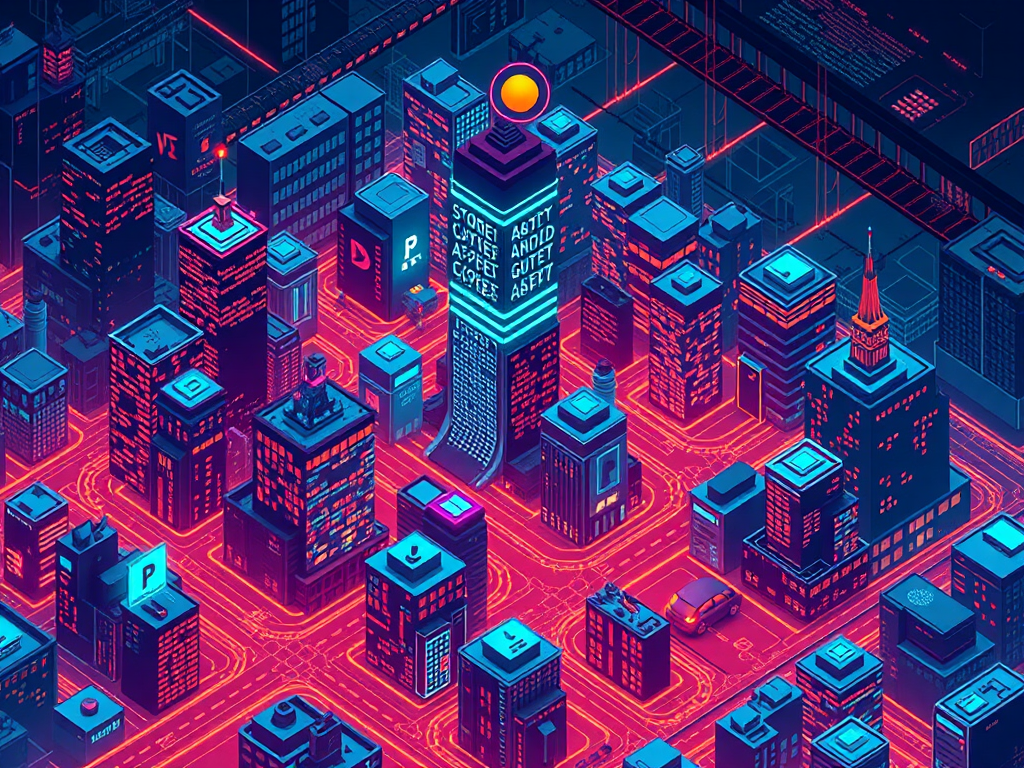