Hello, Python enthusiasts! Today, let's talk about the hot topic of Python API development. As a Python developer, have you ever thought about developing your own API? Or maybe you're using someone else's API but aren't sure how to build one yourself. Don't worry, today I'm going to guide you through the mysteries of Python API development step by step.
Getting Started
First, we need to understand what an API actually is. An API, or Application Programming Interface, is essentially a set of predefined interfaces that allow different software components to communicate with each other. Imagine an API as a waiter in a restaurant; it takes your order (request), communicates your needs to the kitchen (server), and finally brings the delicious dish (data or functionality) to your table.
So why are APIs so important in modern software development? Simply put, APIs make software development modular and reusable. You don't need to write code from scratch every time; instead, you can call existing APIs to implement complex features. This greatly improves development efficiency and allows different systems to collaborate better.
Framework Selection
When it comes to Python API development, we have to mention two super popular frameworks: Flask and Django REST Framework. These two frameworks are like chefs with different styles, each with their own specialties.
Flask: The Lightweight and Flexible Chef
Flask is like a flexible little chef; it's lightweight and simple, yet can create all kinds of delicious dishes. For developers working on small projects or wanting to quickly prototype, Flask is an ideal choice.
Here's how to make a simple API with Flask:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def hello():
return 'Hello, API world!'
@app.route('/echo', methods=['POST'])
def echo():
data = request.get_json()
return jsonify({"You said": data['message']})
if __name__ == '__main__':
app.run(debug=True)
See? In just a few lines of code, we've created two API endpoints. A simple GET request and a POST request that echoes back. The charm of Flask lies in its simplicity and intuitiveness.
Django REST Framework: The All-Round Chef
In contrast, Django REST Framework is like an all-round chef; it provides a complete set of tools and features suitable for building complex, large-scale APIs. If your project needs to handle complex data relationships, user authentication, and permission control, Django REST Framework is your best choice.
Here's a simple example with Django REST Framework:
from rest_framework import serializers, viewsets
from myapp.models import Book
class BookSerializer(serializers.ModelSerializer):
class Meta:
model = Book
fields = ['id', 'title', 'author', 'published_date']
class BookViewSet(viewsets.ModelViewSet):
queryset = Book.objects.all()
serializer_class = BookSerializer
This code may look more complex than the Flask example, but it actually does more for us. It automatically creates a complete CRUD (Create, Read, Update, Delete) API for the Book model.
Hands-On Practice
Okay, we've covered the theoretical knowledge, now let's put it into practice! We'll create a simple book management API.
First, let's implement it with Flask:
from flask import Flask, request, jsonify
app = Flask(__name__)
books = [
{"id": 1, "title": "Python Crash Course", "author": "Eric Matthes"},
{"id": 2, "title": "Fluent Python", "author": "Luciano Ramalho"}
]
@app.route('/books', methods=['GET'])
def get_books():
return jsonify(books)
@app.route('/books/<int:book_id>', methods=['GET'])
def get_book(book_id):
book = next((book for book in books if book['id'] == book_id), None)
if book:
return jsonify(book)
return jsonify({"error": "Book not found"}), 404
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.get_json()
new_book['id'] = len(books) + 1
books.append(new_book)
return jsonify(new_book), 201
if __name__ == '__main__':
app.run(debug=True)
This API allows us to get all books, get details of a single book, and add new books. Isn't it intuitive?
Now, let's implement the same functionality with Django REST Framework:
from django.db import models
from rest_framework import serializers, viewsets
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=100)
class BookSerializer(serializers.ModelSerializer):
class Meta:
model = Book
fields = ['id', 'title', 'author']
class BookViewSet(viewsets.ModelViewSet):
queryset = Book.objects.all()
serializer_class = BookSerializer
This code looks simpler than the Flask version, but it provides more functionality. Django REST Framework automatically creates a full set of CRUD operations for us, including list views, detail views, creation, updating, and deletion.
Advanced Techniques
1. Error Handling
Proper error handling is very important in API development. Let's see how to gracefully handle errors in Flask:
from flask import Flask, jsonify
app = Flask(__name__)
class APIError(Exception):
status_code = 400
def __init__(self, message, status_code=None, payload=None):
super().__init__()
self.message = message
if status_code is not None:
self.status_code = status_code
self.payload = payload
def to_dict(self):
rv = dict(self.payload or ())
rv['message'] = self.message
return rv
@app.errorhandler(APIError)
def handle_api_error(error):
response = jsonify(error.to_dict())
response.status_code = error.status_code
return response
@app.route('/example')
def example():
raise APIError('This is an example error', status_code=418)
if __name__ == '__main__':
app.run(debug=True)
This example shows how to create a custom error class and globally handle these errors in Flask. This way, we can throw APIError
anywhere, and Flask will automatically convert it to an appropriate JSON response.
2. Authentication and Authorization
Security is always an important topic in API development. Django REST Framework provides strong authentication and permission control mechanisms. Let's look at an example:
from rest_framework import viewsets, permissions
from rest_framework.authentication import TokenAuthentication
class BookViewSet(viewsets.ModelViewSet):
queryset = Book.objects.all()
serializer_class = BookSerializer
authentication_classes = [TokenAuthentication]
permission_classes = [permissions.IsAuthenticated]
def perform_create(self, serializer):
serializer.save(owner=self.request.user)
This code adds token authentication and permission control to our BookViewSet. Only authenticated users can access this API, and when creating a new book, we automatically set the current user as the owner of the book.
3. API Versioning
As your API evolves, versioning becomes increasingly important. Here's an example of using URLs for versioning in Flask:
from flask import Flask, Blueprint
app = Flask(__name__)
api_v1 = Blueprint('api_v1', __name__, url_prefix='/api/v1')
api_v2 = Blueprint('api_v2', __name__, url_prefix='/api/v2')
@api_v1.route('/hello')
def hello_v1():
return 'Hello from API v1!'
@api_v2.route('/hello')
def hello_v2():
return 'Hello from API v2!'
app.register_blueprint(api_v1)
app.register_blueprint(api_v2)
if __name__ == '__main__':
app.run(debug=True)
This example shows how to use Flask's Blueprint feature to implement API versioning. This way, we can maintain multiple versions of the API simultaneously without interfering with each other.
Performance Optimization
When your API starts receiving a large number of requests, performance optimization becomes crucial. Here are a few tips to help you improve your API's performance:
-
Use Caching: For data that is frequently requested but not often changed, use caching systems like Redis or Memcached.
-
Asynchronous Processing: For time-consuming operations, consider using asynchronous processing. Python's asyncio library or Celery task queue are good choices.
-
Database Optimization: Use appropriate indexes, optimize query statements, and consider using database connection pools.
-
Load Balancing: When a single server cannot handle all requests, use a load balancer to distribute requests to multiple servers.
-
Compression: Use compression algorithms like gzip to reduce the amount of data transmitted.
Here's an example of using Flask-Caching for caching:
from flask import Flask
from flask_caching import Cache
app = Flask(__name__)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/expensive-operation')
@cache.cached(timeout=60) # Cache the result for 60 seconds
def expensive_operation():
# Assume this is a time-consuming operation
import time
time.sleep(5)
return 'Operation completed'
if __name__ == '__main__':
app.run(debug=True)
This example shows how to use Flask-Caching to cache the result of a time-consuming operation. This way, during the cache validity period, subsequent requests can directly return the cached result, greatly improving response speed.
Documentation and Testing
Last but not least, don't forget to write documentation and tests for your API. Good documentation can make it easier for other developers to understand and use your API, while comprehensive testing ensures your API works as expected.
For documentation, consider using tools like Swagger/OpenAPI. They not only generate beautiful API documentation but also provide interactive API testing interfaces.
For testing, Python's unittest module or the pytest framework are good choices. Here's an example of using unittest to test a Flask API:
import unittest
import json
from app import app
class APITestCase(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
def test_get_books(self):
response = self.app.get('/books')
self.assertEqual(response.status_code, 200)
data = json.loads(response.data)
self.assertIsInstance(data, list)
def test_add_book(self):
new_book = {"title": "Test Book", "author": "Test Author"}
response = self.app.post('/books', json=new_book)
self.assertEqual(response.status_code, 201)
data = json.loads(response.data)
self.assertEqual(data['title'], new_book['title'])
if __name__ == '__main__':
unittest.main()
This test case checks whether our API can correctly fetch the list of books and add new books. By running these tests, we can ensure that the basic functions of the API work properly.
Conclusion
Well, our journey into Python API development ends here. We started with the basic concept of APIs, explored the popular frameworks Flask and Django REST Framework, and then delved into some advanced techniques and best practices in API development.
Remember, developing a good API is not just about writing code. It also involves reasonable design, thoughtful error handling, appropriate security measures, good performance optimization, clear documentation, and comprehensive testing.
As a Python developer, you're fortunate to have such a rich set of tools and resources to build powerful APIs. Whether you choose the lightweight Flask or the all-round Django REST Framework, the important thing is to make informed choices based on the needs of your project.
Finally, I want to say that API development is a process of continuous learning and improvement. Technology is constantly evolving, and new best practices are continually emerging. Keep the passion for learning, be willing to try new things, and I believe you'll become an outstanding API developer!
So, are you ready to start your API development journey? If you have any ideas or questions, feel free to leave a comment for discussion!
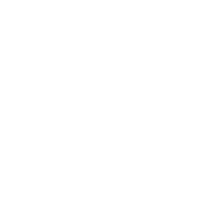
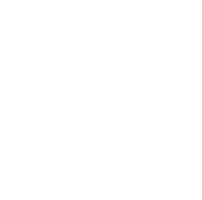