In the previous section, we explored the basics and advanced techniques of Python API development. Today, let's go further and see how to use APIs to make data truly flow, creating more powerful and useful applications.
The Magic of Data
Have you ever wondered why today's applications can be so intelligent and convenient? The answer lies in the flow of data. Through APIs, we can enable free exchange of data between different systems and services, as if building a massive data ecosystem.
Imagine you're developing a travel application. You need weather information, hotel bookings, flight queries, and various other data. Without APIs, you might need to manually collect and update all this information. But with APIs, you can obtain the latest data in real-time, providing users with the most accurate information.
The Art of RESTful APIs
When it comes to APIs, we must mention RESTful APIs. REST (Representational State Transfer) is a design style that makes APIs more intuitive and easy to use. Let's see how to create a simple RESTful API using Python:
from flask import Flask, jsonify, request
app = Flask(__name__)
books = [
{"id": 1, "title": "Python Programming", "author": "Zhang San"},
{"id": 2, "title": "API Development Guide", "author": "Li Si"}
]
@app.route('/books', methods=['GET'])
def get_books():
return jsonify({"books": books})
@app.route('/books/<int:book_id>', methods=['GET'])
def get_book(book_id):
book = next((book for book in books if book["id"] == book_id), None)
if book:
return jsonify({"book": book})
return jsonify({"error": "Book not found"}), 404
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.json
new_book["id"] = max(book["id"] for book in books) + 1
books.append(new_book)
return jsonify({"book": new_book}), 201
if __name__ == '__main__':
app.run(debug=True)
This simple API allows us to get all books, get a specific book, and add new books. Don't you feel like you've mastered the essence of RESTful APIs?
The Importance of Data Validation
Data validation becomes particularly important when receiving user input. We can easily implement data validation using the marshmallow
library:
from marshmallow import Schema, fields, ValidationError
class BookSchema(Schema):
id = fields.Int(dump_only=True)
title = fields.Str(required=True)
author = fields.Str(required=True)
book_schema = BookSchema()
@app.route('/books', methods=['POST'])
def add_book():
try:
new_book = book_schema.load(request.json)
except ValidationError as err:
return jsonify(err.messages), 400
new_book["id"] = max(book["id"] for book in books) + 1
books.append(new_book)
return jsonify({"book": book_schema.dump(new_book)}), 201
By using marshmallow
, we can ensure that received data meets our expected format, improving the API's robustness.
The Wisdom of Pagination
When dealing with large amounts of data, pagination becomes very important. Let's see how to implement pagination:
@app.route('/books', methods=['GET'])
def get_books():
page = request.args.get('page', 1, type=int)
per_page = request.args.get('per_page', 10, type=int)
start = (page - 1) * per_page
end = start + per_page
paginated_books = books[start:end]
return jsonify({
"books": paginated_books,
"total": len(books),
"page": page,
"per_page": per_page
})
By implementing pagination, we can handle large amounts of data more effectively, improving API performance and user experience.
The Art of Version Control
As APIs evolve, version control becomes increasingly important. We can include version information in URLs:
@app.route('/v1/books', methods=['GET'])
def get_books_v1():
# V1 version implementation
@app.route('/v2/books', methods=['GET'])
def get_books_v2():
# V2 version implementation
Through version control, we can gradually introduce new features or changes without affecting existing users.
The Magic of Caching
To improve API performance, we can use caching. The Flask-Caching extension makes caching simple:
from flask_caching import Cache
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/books/<int:book_id>', methods=['GET'])
@cache.cached(timeout=60) # Cache for 60 seconds
def get_book(book_id):
book = next((book for book in books if book["id"] == book_id), None)
if book:
return jsonify({"book": book})
return jsonify({"error": "Book not found"}), 404
By using caching, we can greatly reduce database queries and improve API response speed.
The Art of Authentication
For API endpoints that need protection, we can implement JWT (JSON Web Token) authentication using Flask-JWT-Extended:
from flask_jwt_extended import JWTManager, jwt_required, create_access_token
app.config['JWT_SECRET_KEY'] = 'your-secret-key' # Should use environment variables in production
jwt = JWTManager(app)
@app.route('/login', methods=['POST'])
def login():
username = request.json.get('username', None)
password = request.json.get('password', None)
if username == 'admin' and password == 'password':
access_token = create_access_token(identity=username)
return jsonify(access_token=access_token), 200
return jsonify({"msg": "Bad username or password"}), 401
@app.route('/protected', methods=['GET'])
@jwt_required
def protected():
return jsonify({"msg": "This is a protected endpoint!"})
By implementing JWT authentication, we can ensure that only authorized users can access protected API endpoints.
The Magic of Documentation
Good documentation can greatly improve API usability. We can use Swagger UI to generate interactive documentation for our API:
from flask_swagger_ui import get_swaggerui_blueprint
SWAGGER_URL = '/api/docs' # URL for exposing Swagger UI (without trailing '/')
API_URL = '/static/swagger.json' # Our API url (can of course be a local resource)
swaggerui_blueprint = get_swaggerui_blueprint(
SWAGGER_URL, # Swagger UI static files will be mapped to '{SWAGGER_URL}/dist/'
API_URL,
config={ # Swagger UI config overrides
'app_name': "Test application"
},
)
app.register_blueprint(swaggerui_blueprint)
Through Swagger UI, other developers can easily understand and test your API.
The Wisdom of Error Handling
Elegant error handling can greatly improve API user experience. We can create custom error handlers:
@app.errorhandler(404)
def not_found(error):
return jsonify({"error": "Not found"}), 404
@app.errorhandler(500)
def internal_error(error):
return jsonify({"error": "Internal server error"}), 500
Through custom error handling, we can provide more meaningful error messages to API users.
The Art of Performance Monitoring
To ensure API performance, we need monitoring. We can implement this using Flask-MonitoringDashboard:
import flask_monitoringdashboard as dashboard
dashboard.bind(app)
By using monitoring tools, we can identify and resolve performance issues promptly.
Summary
We've deeply explored various aspects of Python API development, from basic RESTful design to advanced authentication, caching, and monitoring. By mastering these techniques, you can create powerful, efficient, and secure APIs that truly make data flow!
Remember, API development is a continuous process of learning and improvement. As technology evolves, new best practices and tools continue to emerge. Maintain your enthusiasm for learning, keep practicing and innovating, and you can become an excellent API developer.
So, are you ready to let data flow freely in your applications? Start your API development journey, and let's create a more intelligent and convenient digital world together!
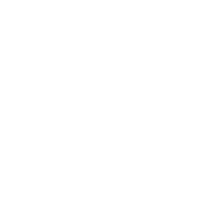
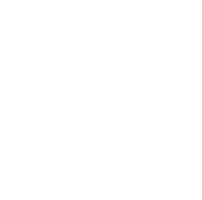