Introduction
Have you ever encountered a situation where you wrote some file handling code, only to have it mysteriously crash at runtime, leaving you baffled? Or perhaps the code seems fine but lacks a bit of safety and robustness. Today, I'll share with you how to gracefully handle various exceptions in file operations using Python.
Common Pitfalls
In my years of teaching Python, I've found that beginners often fall into a few common traps when handling files:
- Forgetting to close a file after opening it
- Not considering the possibility of a file not existing
- Failing to handle read/write permission issues
- Garbled text due to encoding format issues
Let's first look at a common example of erroneous code:
def read_file(filename):
file = open(filename, 'r')
content = file.read()
file.close()
return content
data = read_file('my_file.txt')
print(data)
This code seems fine but actually hides many risks. What if the file doesn't exist? Will the file close properly if an exception occurs during reading?
Best Practices
Let's improve this code step by step. First, use the with
statement to ensure the file is closed properly:
def read_file_better(filename):
try:
with open(filename, 'r', encoding='utf-8') as file:
content = file.read()
return content
except FileNotFoundError:
print(f"The file {filename} does not exist")
return None
except PermissionError:
print(f"No permission to read the file {filename}")
return None
except UnicodeDecodeError:
print(f"Incorrect encoding format for file {filename}")
return None
Practical Tips
In real-world projects, we often need to handle large files, which requires considering memory usage. I find many beginners like to read the entire file at once, which can lead to memory overflow when dealing with large files. Let's see how to improve this:
def process_large_file(filename):
try:
with open(filename, 'r', encoding='utf-8') as file:
for line in file:
# Process line by line to avoid loading the entire file at once
yield line.strip()
except OSError as e:
print(f"Error processing the file {filename}: {e}")
yield None
for line in process_large_file('large_file.txt'):
if line is not None:
# Process each line of data
process_line(line)
Advanced Applications
Did you know? Python also offers some cool ways to handle files. For instance, we can use the pathlib
module to handle file paths more elegantly:
from pathlib import Path
def smart_file_handling(filename):
file_path = Path(filename)
if not file_path.exists():
print(f"The file {filename} does not exist")
return
if not file_path.is_file():
print(f"{filename} is not a file")
return
try:
with file_path.open('r', encoding='utf-8') as file:
return file.read()
except Exception as e:
print(f"Error reading the file: {e}")
return None
Useful Advice
From my development experience, here are some tips for handling files:
- Always use the
with
statement to automatically handle file closing - Explicitly specify file encoding (usually use utf-8)
- Use generators to process large files line by line
- Use exception handling wisely to make your code more robust
- Consider using the
pathlib
module to handle file paths
Conclusion and Outlook
File operations are a fundamental skill in Python programming, and mastering exception handling can make your code more robust. Do you find these tips useful? Feel free to share your experiences and thoughts in the comments section.
Next time, we can delve into Python's context managers, which are not only useful for file operations but also applicable in many other scenarios. If you're interested in this topic, don't forget to follow my blog.
Have you ever encountered tricky file handling issues in real projects? Let's discuss and learn together.
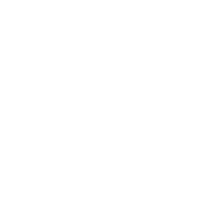
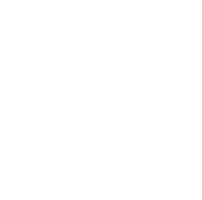