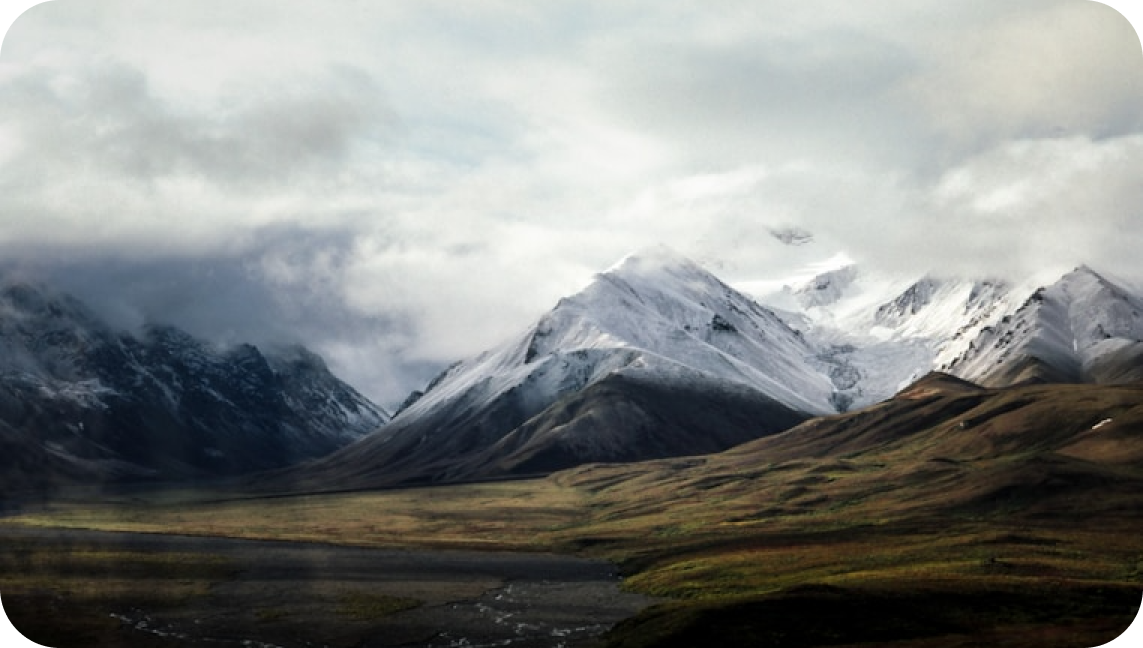
Introduction
Hello everyone! Today we're going to discuss some tips and experiences for getting started with Python programming. As an experienced Python blogger, I'm very passionate about conveying dry programming knowledge to you all in a lively and interesting way. So let's get started!
Introduction to Python
Python is an elegant and simple programming language with very concise and easy-to-understand syntax rules and high readability. This makes Python very suitable for beginners in programming. Even complete novices without any programming background won't find it too difficult to learn. However, while Python is simple to get started with, it is very powerful and widely used in website development, data analysis, artificial intelligence, and many other fields. So learning Python is definitely a good investment that can open the door to the world of programming for you.
You might ask, "How simple is Python exactly?" Let me give you an example. The following code can print numbers from 1 to 10:
for i in range(1, 11):
print(i)
Isn't it super simple? With just these few lines of code, you can already make some interesting little programs. Compared to other languages, getting started with Python is really much easier!
Why Choose Python
Simple and Easy to Learn
The main reason why Python is so popular is its simplicity and ease of learning. Let's look at some features of Python that make it easy for beginners to get started:
-
Concise syntax. As shown in the previous example, Python's syntax rules are very simple and intuitive, making them easy to understand at a glance.
-
High readability. Python code is almost like describing what to do, with excellent readability, facilitating collaboration and maintenance.
-
Interactive programming. You can execute code line by line in the Python interpreter, learning and practicing simultaneously, achieving twice the result with half the effort.
-
Rich libraries. Python has powerful standard libraries and third-party libraries that can quickly implement various functions, achieving twice the result with half the effort.
-
Cross-platform. Python programs can run on Windows, macOS, and Linux systems without modification.
So if you're a programming novice or want to learn a new programming language, Python is definitely a great choice!
Wide Application
In addition to being simple and easy to learn, Python has another huge advantage: its application areas are very wide. Whether you want to do website development, data analysis, or machine learning and artificial intelligence, Python is an invaluable tool.
-
Web scraping. Python's powerful network data collection capabilities make it the go-to choice for scraper development.
-
Scientific computing. Libraries like NumPy and SciPy provide Python with high-performance scientific computing capabilities.
-
Machine learning. Popular frameworks like TensorFlow and PyTorch all provide Python interfaces.
-
Automated operations. Python scripts can automatically complete system management, network programming, and other operational tasks.
-
Game development. Third-party libraries like Pygame can be used to create small games.
-
Visualization development. Plotting libraries like Matplotlib and Bokeh help you easily generate various visualizations.
So you see, Python has broad application prospects in various fields. Mastering Python is equivalent to adding a brand new professional skill for yourself. Isn't that very attractive?
Python Basics
Alright, through the introduction above, I believe you already have a preliminary understanding of Python. So let's officially start learning the basics of Python programming! At this stage, you need to master core concepts such as variables, data types, control flow, and functions.
Variables and Data Types
Variables can be said to be the cornerstone of programming. Defining and using variables in Python is very simple, you just need to assign a value to the variable, for example:
x = 5 # Integer
y = 3.14 # Float
name = "Alice" # String
Python will automatically infer the data type of the variable based on the assignment. For beginners, it's important to focus on mastering the usage of common data types such as integers, floats, strings, and booleans.
In addition, Python also supports compound data types, such as lists and dictionaries. They are also simple to use, you can create them like this:
fruits = ["apple", "banana", "orange"] # List
info = {"name": "Bob", "age": 25} # Dictionary
Through lists and dictionaries, you can conveniently store and manipulate multiple data. Just get a basic understanding of the usage of these data types for now, you'll gradually master them as you practice later.
Control Program Execution Flow
Every program needs flow control, otherwise the code would just keep executing downwards. Python controls the execution flow of the program through conditional statements and loop statements.
Conditional statements decide which piece of code to execute by checking whether a condition is met. Python's if...elif...else
statement is similar to that in other languages, you can use it like this:
x = 10
if x > 0:
print("x is positive")
elif x < 0:
print("x is negative")
else:
print("x equals 0")
Loop statements allow code to be executed repeatedly, where for
loops are commonly used to iterate over sequences, while while
loops continue to execute when a condition is met. For example:
for i in range(5):
print(i) # Outputs 0 1 2 3 4
count = 0
while count < 5:
print(count)
count += 1
By flexibly using conditional statements and loop statements, you can write various logics. However, be aware that overly complex logic will affect the readability of the code, so you should exercise moderation when writing code.
The Power of Functions
Functions are the cornerstone of code reuse in programming and can greatly improve the maintainability of code. Defining functions in Python is very simple:
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Outputs Hello, Alice!
As you can see, the def
keyword is used to define a function, followed by parentheses with specified parameters after the function name. Write the code logic within the function body, and you can achieve reuse.
Functions in Python also support return values, just use the return
statement within the function:
def square(x):
return x * x
result = square(5)
print(result) # Outputs 25
Through return values, functions can pass calculation results to the caller, achieving decoupling.
In addition to custom functions, Python itself has a large number of built-in useful functions, such as len()
, max()
, sorted()
, etc., which can greatly improve programming efficiency, so be sure to master them proficiently.
Programming Practice
Now that you've learned the theoretical knowledge, it's time to start hands-on practice! We'll start with some simple examples and gradually transition to practical projects to help you solidly master Python programming.
Time Processing
The first example is a small program for processing time. We'll design a function that takes two 24-hour format times as input and calculates and displays the time difference.
Let's first look at how to get the user's input time:
first_time = input("Please enter the first time (e.g. 0900):")
second_time = input("Please enter the second time:")
Python's input()
function can get the user's keyboard input. Now we need to convert the string form of time into a time object that's convenient for calculation. This requires the use of the datetime
module:
import datetime
first = datetime.datetime.strptime(first_time, "%H%M").time()
second = datetime.datetime.strptime(second_time, "%H%M").time()
The strptime()
method can parse a string into a datetime
object according to the given format string. Then we extract the time()
part to get a time object of type time
.
Next is a simple time calculation:
hours = second.hour - first.hour
mins = second.minute - first.minute
if mins < 0:
hours -= 1
mins += 60
print(f"The time difference is {hours} hours and {mins} minutes")
We calculate the difference in hours and minutes separately. Note that if the minute difference is negative, we need to borrow from the hour count.
Just like that, a simple time processing program is complete! You can write it yourself and then run it to test. Once you've mastered these basics, you'll be able to handle more challenging programs.
Data Processing
Data processing is a major important application area for Python. Here we'll briefly introduce the use of the Pandas library.
Pandas can efficiently process tabular data and provides a large number of data analysis and visualization functions. Let's first use Pandas to read a CSV file:
import pandas as pd
data = pd.read_csv("sales.csv")
print(data.head()) # Print the first 5 rows
The read_csv()
function can read a CSV file as a DataFrame object. We printed the first 5 rows of data, so we can roughly understand the structure of the data.
Next, let's sum up the sales by region:
sales_by_region = data.groupby("region")["sales"].sum()
print(sales_by_region)
The groupby()
method groups the data by the specified column, and then we call the sum()
method on the sales
column to get the total sales for each region.
Finally, let's generate a simple bar chart to visualize this data:
sales_by_region.plot(kind="bar")
You see, through a few simple lines of code, we've completed the reading, aggregation, and visualization of the data! Pandas has given wings to Python's data processing capabilities, making Python shine in the field of data analysis.
GUI Programming
For the last example, let's look at how to create a graphical user interface (GUI) program using Python. This requires the use of Tkinter, a built-in GUI library.
Let's first create a root window:
import tkinter as tk
root = tk.Tk()
root.title("My First GUI Program")
Then add some widgets, such as labels and buttons:
label = tk.Label(root, text="Hello World!")
label.pack()
def show_message():
print("Hello, Python!")
button = tk.Button(root, text="Click Me", command=show_message)
button.pack()
Finally, start the main event loop:
root.mainloop()
Just that simple, you've created a window program with labels and buttons! When you click the button, the show_message()
function will be called, printing "Hello, Python!" to the console.
Doesn't the GUI program look interesting? You can definitely build on this foundation, add more widgets and logic, and develop powerful desktop applications. Although Python's Tkinter is somewhat rudimentary, it's sufficient for beginners.
Recommended Learning Resources
Through the learning above, I believe you already have a preliminary understanding and practice of Python. However, learning shouldn't stop here. To truly master this language, you need continuous learning and practice.
I recommend some good Python learning resources for everyone:
- Official documentation and tutorials. The Python official website provides complete documentation and beginner tutorials, with very detailed introductions to syntax, modules, etc.
- Online courses. There are many high-quality Python online courses on the internet, such as MIT courses on Coursera and edX.
- Practical projects. Exercising programming skills through practical projects is very effective, and there are many project source codes available online for reference.
- Programming practice websites. Websites like LeetCode and HackerRank have a large number of programming questions where you can practice solving problems.
My suggestion is to first systematically learn Python basic syntax, and then immediately start writing small programs to deepen your understanding. Once you have a good foundation, you can try learning some libraries and frameworks and do some practical projects. Only through more practice can your programming skills continuously improve.
Finally, Python is a vast ecosystem. In addition to programming basics, you can also learn specialized knowledge such as data science, machine learning, and web development. Choose the direction you're interested in for in-depth study based on your interests and hobbies, and you'll definitely gain a lot!
Conclusion
Alright everyone, that's all for today! Through my explanation, I believe you now have a comprehensive understanding of Python. Although Python is easy to get started with, mastering it is not something that can be achieved overnight. Let's persist in learning and continuous practice together, start our programming journey with Python, and we'll definitely be able to create amazing works!
If you have any questions, feel free to ask me anytime. Let's communicate and progress together! The charm of Python is endless, let's open up new programming horizons together!
Next
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.
Next
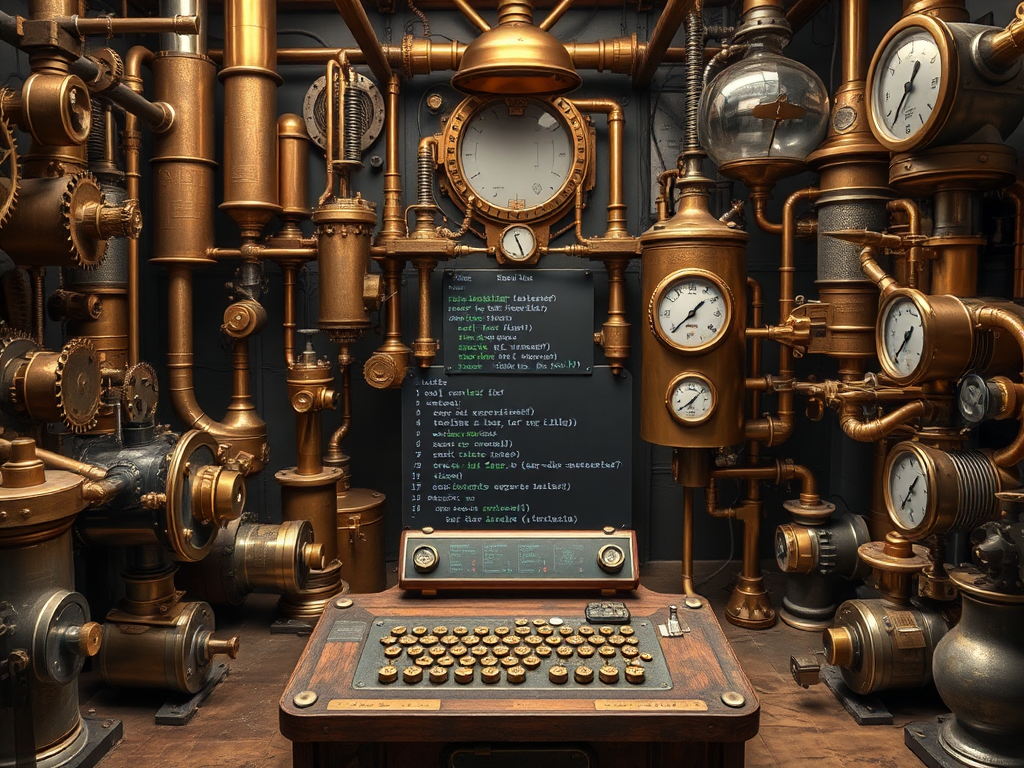
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
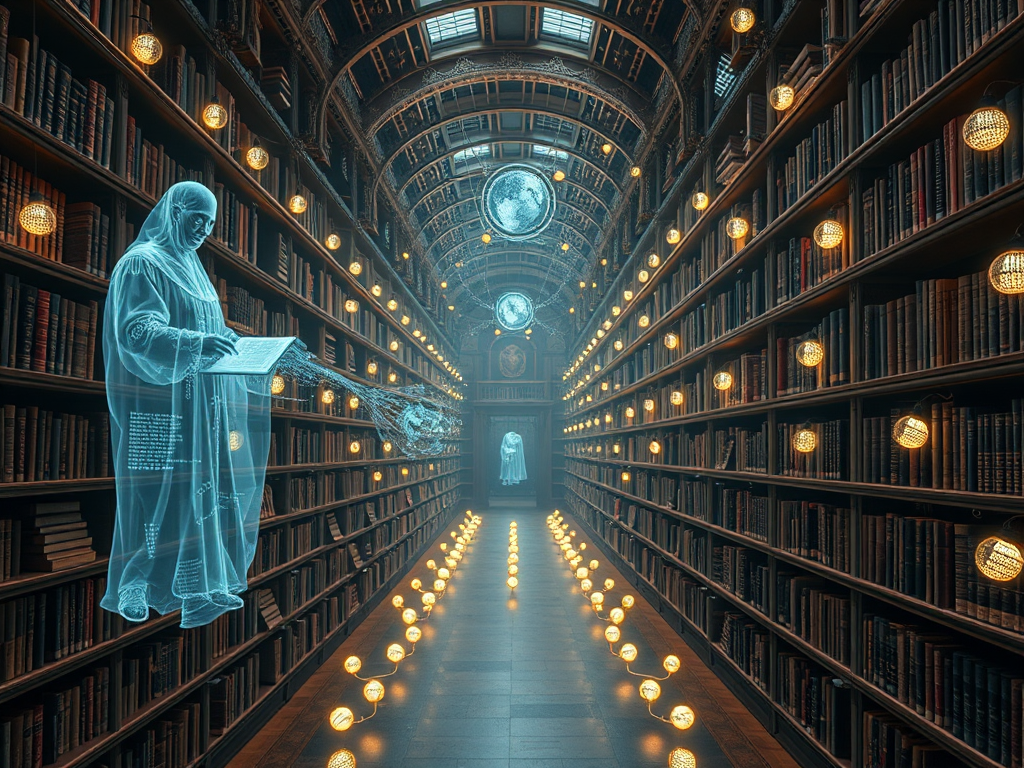
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
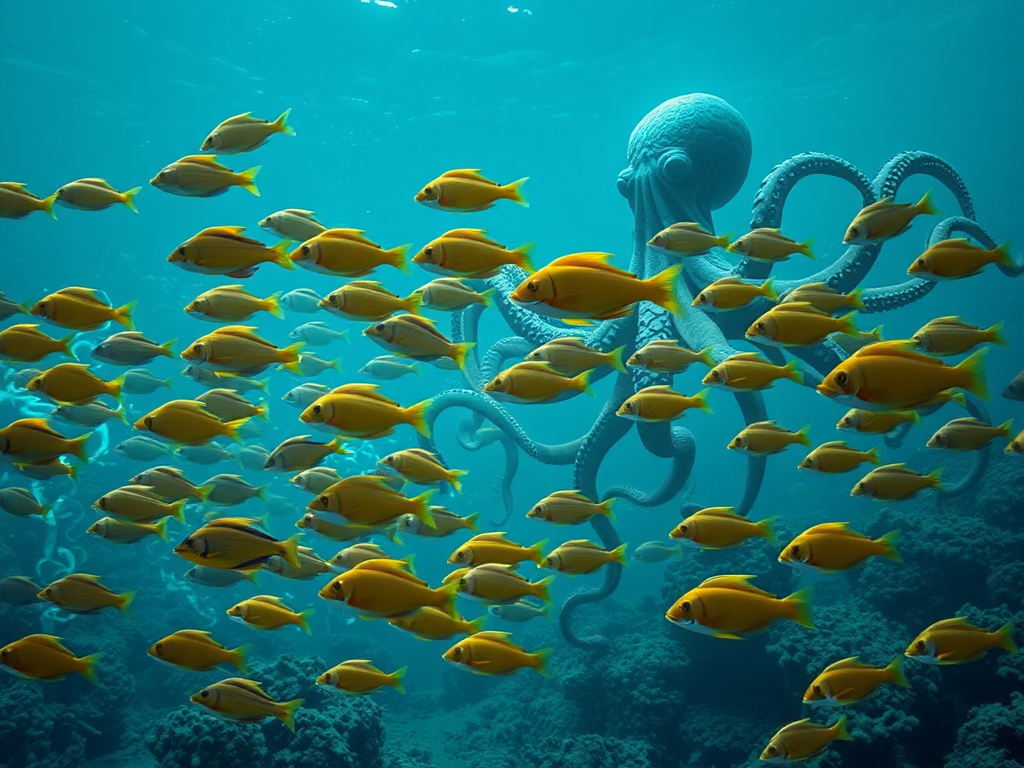
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.