Introduction
Have you often heard the term Object-Oriented Programming (OOP)? As a Python programmer, I was also confused when I first encountered OOP. What are classes? What are objects? What do concepts like inheritance, polymorphism, and encapsulation mean? Today, let's explore object-oriented programming in Python together.
Basics
Object-oriented programming is a programming paradigm that organizes data and methods for processing data together into an organic whole. This might sound abstract, so let's understand it with a concrete example.
Imagine you're developing a book management system. Using traditional procedural programming, you might write:
book_name = "Python Programming"
book_author = "Zhang San"
book_price = 59.9
book_isbn = "9787111111111"
def print_book_info(name, author, price, isbn):
print(f"Title:{name}")
print(f"Author:{author}")
print(f"Price:{price}")
print(f"ISBN:{isbn}")
But using object-oriented programming, we can organize code like this:
class Book:
def __init__(self, name, author, price, isbn):
self.name = name
self.author = author
self.price = price
self.isbn = isbn
def print_info(self):
print(f"Title:{self.name}")
print(f"Author:{self.author}")
print(f"Price:{self.price}")
print(f"ISBN:{self.isbn}")
my_book = Book("Python Programming", "Zhang San", 59.9, "9787111111111")
my_book.print_info()
Want to know the difference between these two approaches? The object-oriented approach encapsulates data (title, author, price, ISBN) and methods that operate on this data (print_info) in a structure called a "class". This has many benefits: code is easier to maintain, easier to reuse, and more aligned with human thinking patterns.
Intermediate
When discussing object-oriented programming, we must mention inheritance, an important feature. Inheritance allows us to create new classes based on existing ones, where new classes can inherit all features of the original class and add their own features.
For example, in our book management system to distinguish between ebooks and physical books:
class EBook(Book):
def __init__(self, name, author, price, isbn, format_type):
super().__init__(name, author, price, isbn)
self.format_type = format_type
def print_info(self):
super().print_info()
print(f"Format:{self.format_type}")
my_ebook = EBook("Python Programming", "Zhang San", 29.9, "9787111111111", "PDF")
my_ebook.print_info()
In this example, the EBook class inherits all features of the Book class and adds the format_type attribute specific to ebooks. This is the power of inheritance.
Advanced
Another important concept in object-oriented programming is polymorphism. Polymorphism means different classes can have different implementations of the same method. Here's an example:
class AudioBook(Book):
def __init__(self, name, author, price, isbn, duration):
super().__init__(name, author, price, isbn)
self.duration = duration
def print_info(self):
super().print_info()
print(f"Duration:{self.duration} minutes")
def display_book_info(book):
book.print_info()
paper_book = Book("Python Basics", "Li Si", 69.9, "9787111222222")
ebook = EBook("Python Advanced", "Wang Wu", 39.9, "9787111333333", "EPUB")
audio_book = AudioBook("Python Expert", "Zhao Liu", 89.9, "9787111444444", 360)
display_book_info(paper_book)
display_book_info(ebook)
display_book_info(audio_book)
See? The same print_info method call displays different information for different types of books. This is the charm of polymorphism.
Practical Application
Let's look at a more complex example, a simple book management system:
class BookManager:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
print(f"Successfully added book '{book.name}'")
def remove_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
self.books.remove(book)
print(f"Successfully removed book '{book.name}'")
return
print("Book not found")
def search_book(self, keyword):
found_books = []
for book in self.books:
if keyword.lower() in book.name.lower() or \
keyword.lower() in book.author.lower():
found_books.append(book)
return found_books
manager = BookManager()
manager.add_book(Book("Python Basic Tutorial", "Zhang San", 69.9, "9787111222222"))
manager.add_book(EBook("Python Network Programming", "Li Si", 39.9, "9787111333333", "PDF"))
manager.add_book(AudioBook("Python Data Analysis", "Wang Wu", 89.9, "9787111444444", 480))
search_results = manager.search_book("python")
for book in search_results:
book.print_info()
print("-" * 20)
This example demonstrates how to apply object-oriented concepts in a real project. The BookManager class encapsulates core book management functionality, including adding, removing, and searching for books. Through inheritance and polymorphism, we can easily handle different types of books.
Insights
Through these examples, I think you now have a deeper understanding of Python's object-oriented programming. Let me share some personal insights:
-
Class design should follow the single responsibility principle. A class should be responsible for only one thing, making code easier to maintain and extend.
-
Use inheritance relationships cautiously. Overusing inheritance can make code difficult to understand and maintain. Sometimes composition is more appropriate than inheritance.
-
Encapsulation is important. Using private attributes and methods (starting with double underscores in Python), we can better control class interfaces.
-
Polymorphism makes code more flexible. Through polymorphism, we can write more generic code to handle different types of objects.
Looking Forward
Object-oriented programming is a powerful tool, but it's not omnipotent. In real development, we need to choose appropriate programming paradigms based on specific situations. Sometimes procedural programming is simpler and more direct, sometimes functional programming is more suitable. The key is understanding the advantages and disadvantages of each paradigm and using them flexibly.
What do you think about this article? Feel free to share your thoughts and experiences in the comments. If you have any questions, feel free to discuss them. Let's learn and progress together.
There are many more interesting features of Python's object-oriented programming waiting for us to explore. Such as metaclasses, descriptors, property decorators (@property), etc. Which features are you interested in? Perhaps we can discuss them in detail in the next article.
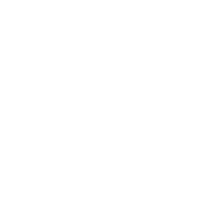