Getting to Know Python
Hello, dear readers! Today we're going to discuss the basics of Python programming. Python is an elegant, concise, and highly practical programming language that's worth spending time learning and mastering, whether you're a programming novice or an experienced coder.
As a Python programming enthusiast, I often receive questions from friends or readers like: "What are the data types in Python?", "How do you define and call functions?", "How do you handle exceptions?", and so on. Today, let's address these questions one by one and open the door to Python programming for you.
Many Types of Data
Python has several data types, with numbers and strings being the most commonly used. Numeric types include integers (int) and floating-point numbers (float), such as 1 and 3.14. Strings are sequences of characters enclosed in single or double quotes, like 'hello' or "world".
Of course, besides individual numbers and strings, Python also supports data structures like lists, tuples, and dictionaries. For example, a list is an ordered collection that can store elements of different types. You can create a list like this:
my_list = [1, 2, 'three', 4.0]
This list contains integers, a string, and a float. You can access elements in the list using indices, for instance, my_list[0]
will return 1. Lists support various operations like adding, removing, and sorting, making them quite convenient to use.
Input and Output
After learning about data types, let's look at how to interact with programs. In Python, you can get user input using the input()
function and output content to the user using the print()
function.
Here's a small example:
name = input("Please enter your name: ")
print(f"Hello, {name}!")
Here, we first use input()
to get the user's name and store it in the variable name
. Then we use the print()
function, utilizing a formatted string, to print a greeting to the user.
Don't you find this example interesting? With just a few lines of code, we've achieved interaction with the program. This is the charm of Python - simple to learn yet powerful.
Conditions and Loops
In programming, we often need to execute different operations based on different conditions, or repeat certain code. In Python, this is where conditional statements and loop statements come in.
First, let's look at conditional statements. Python uses the if
, elif
, and else
keywords to implement these, with the following syntax:
if condition1:
code_block1
elif condition2:
code_block2
else:
code_block3
If condition1 is true, code_block1 is executed; otherwise, condition2 is checked, and if true, code_block2 is executed; if all conditions are not met, code_block3 is executed.
Now let's look at loop statements. Python has two types of loops: for
loops and while
loops. for
loops are typically used to iterate over sequences (like lists):
fruits = ['apple', 'banana', 'orange']
for fruit in fruits:
print(f"I like to eat {fruit}")
While while
loops continue to execute the loop body based on a condition:
count = 0
while count < 5:
print(f"Current count: {count}")
count += 1
By flexibly using conditional statements and loop statements, you can write more intelligent and powerful programs.
Functions in Action
Functions play a central role in programming. In Python, you define functions using the def
keyword, and call functions simply by using the function name.
Let's look at a simple function example:
def greet(name):
"""
Print a greeting
"""
print(f"Hello, {name}!")
greet('Python') # Output: Hello, Python!
Here we've defined a function called greet
that takes a name
parameter. The function body uses a print
statement to output a greeting including that name. Finally, we call the greet
function, passing the string 'Python'
as an argument, resulting in the output.
You see, by encapsulating code into functions, we can organize code logically, improving its readability and maintainability. Moreover, functions can return values, making the code even more flexible.
Handling Exceptions Gracefully
In the process of programming, we inevitably encounter various exceptional situations, such as users entering illegal characters, files failing to open, and so on. If not handled, the program would crash and exit directly.
Fortunately, Python provides us with the try-except
structure to catch and handle exceptions, allowing the program to continue running gracefully. For example:
try:
num = int(input("Please enter an integer: "))
result = 100 / num
print(f"The result is: {result}")
except ValueError:
print("You didn't enter a valid integer!")
except ZeroDivisionError:
print("Cannot divide by zero!")
In this example, we first use the code in the try
block to get user input and calculate the result. If the user enters a non-integer, it will raise a ValueError
exception, and the program will execute the code in the except ValueError
block. If the user enters 0, it will raise a ZeroDivisionError
exception, and the corresponding except
block will be executed.
By skillfully using the try-except
structure, you can write more robust Python programs. It's worth mentioning that Python has many other exception types, which you can learn and master gradually in practice.
Time is Money
Finally, let's look at a practical example: how to handle time and dates in Python. Python provides the datetime
module, which makes operations like time and date calculations and formatting very simple.
For instance, if we have two 24-hour military times and want to calculate the time difference between them, we can do it like this:
from datetime import datetime
first = input("Please enter the first time (HHMM): ")
second = input("Please enter the second time (HHMM): ")
first_time = datetime.strptime(first, "%H%M")
second_time = datetime.strptime(second, "%H%M")
difference = second_time - first_time
hours = difference.seconds // 3600
minutes = (difference.seconds % 3600) // 60
print(f"The time difference is {hours} hours and {minutes} minutes")
This code first gets two military time format strings from the user. Then it uses the strptime
function to convert them into datetime
objects. Next, we calculate the difference between the two time objects and convert the total seconds into hours and minutes, finally printing the time difference.
You see, by mastering the datetime
module, we can easily handle many programming tasks related to time and date. So, time is money, and by mastering the correct time handling techniques, you can avoid many detours in your programming journey.
Summary and Outlook
Today, we've learned the basics of Python programming, including data types, input and output, conditions and loops, functions, exception handling, and time handling. I believe that through this content, you've gained an initial understanding of Python.
Of course, there's much more to Python than this. In the future, we can learn about Python's object-oriented programming, file operations, database interactions, network programming, and other advanced topics. I will continue to share more Python tips and applications on my blog, so stay tuned!
Finally, I want to emphasize again the joy of learning programming. Programming not only exercises our logical thinking abilities but also allows us to experience the happiness of creation. So, let's start practicing together! As long as you maintain enthusiasm and patience, I believe you'll soon be able to master Python and embark on your programming journey.
The Python journey is just beginning, looking forward to seeing you again!
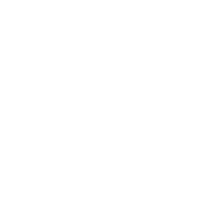
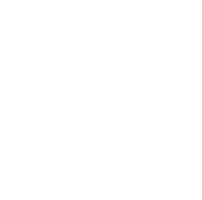