Hello everyone, today we're going to delve into two concepts in Python that may seem advanced but are actually quite commonly used: generators and metaclasses. Through this article, I hope to give you a deeper understanding of them, enabling you to use them with ease in your programming practice.
Generators, Not Your Simple Iterators
I'm sure you're all familiar with the concept of Python iterators. An iterator is an object that implements the iterator protocol, allowing it to be traversed by a for
loop or to continuously retrieve the next value through the next()
function.
Generators are actually a special kind of iterator. However, unlike iterators implemented through classes, generators are implemented through functions. You're probably very familiar with this type of function:
def count_up_to(n):
i = 0
while i < n:
yield i
i += 1
See that? This function uses the yield
keyword, which turns a function into a generator. Whenever yield
is encountered, the function pauses and returns a result, and the next time it continues executing, it resumes from where it last paused.
What's the benefit of generators? The biggest advantage is laziness. A list containing a large number of elements needs to be constructed all at once in memory, while a generator can produce elements one by one, thus saving memory. Imagine, if you want to iterate over the squares of all natural numbers, which is better, a list or a generator?
squares = [x**2 for x in range(10**8)] # Memory explosion
square_generator = (x**2 for x in range(10**8))
for square in square_generator:
print(square)
Generators are not only lazy, but they can also produce elements infinitely. This is very useful for some special iteration scenarios, such as the Fibonacci sequence:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci()
print(next(fib)) # 0
print(next(fib)) # 1
print(next(fib)) # 1
Look, we easily implemented an infinite Fibonacci sequence generator using a generator. This technique also has important applications in certain algorithms and data structures, such as generating infinitely long pseudo-random number sequences.
Generator functions can not only use the yield
statement, but also use the send
method to receive external data. This gives generators a certain "coroutine" capability, allowing bidirectional data transfer between the generator and the caller. This topic is too advanced, so we won't expand on it in this article.
In short, generators, as a feature of the Python language, make the use of iterators more concise and efficient. When you're dealing with large amounts of data or need infinite sequences, generators are definitely a tool worth mastering.
Metaclasses, The Classes Behind Classes
We all know that in Python, everything is an object, including classes themselves. So, what creates classes? The answer is metaclasses.
The concept of metaclasses is actually very simple, it's "the class that creates classes". When you define a class, the Python interpreter actually creates this class object by calling a metaclass. For example:
class MyClass:
pass
obj = MyClass()
This code actually runs like this:
- When Python encounters
class MyClass:
, it uses the built-intype
metaclass to create a new class objectMyClass
. - After creation, you can instantiate this class through
MyClass()
to create an instance objectobj
.
So, type
is actually the metaclass that Python uses behind the scenes to create all classes. You can manually call type
to create a class:
MyClass = type('MyClass', (), {})
obj = MyClass()
print(type(obj)) # <class '__main__.MyClass'>
The last line of this code output indicates that we indeed created a new class using the type
metaclass.
You might ask, why learn about metaclasses? Metaclasses provide an extensible mechanism that allows you to inject custom behavior into the class creation process. This is very useful when implementing some advanced techniques, such as:
- Automatically logging class attributes
- Automatically implementing thread safety
- Automatically binding method dispatchers
- Implementing feature-based object programming paradigms
- ...
Let's look at a simple example of implementing the singleton pattern through a custom metaclass:
class Singleton(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super(Singleton, cls).__call__(*args, **kwargs)
return cls._instances[cls]
class MyClass(metaclass=Singleton):
pass
obj1 = MyClass()
obj2 = MyClass()
print(obj1 is obj2) # True
As you can see, we customized a Singleton
metaclass and overrode its __call__
method. This way, no matter how many times we instantiate MyClass
, it always returns the same instance object.
Through the above example, you should have a basic understanding of metaclasses. Metaclass programming is a very useful but high-threshold topic. If you're interested in it, you can continue to study it in depth. However, understanding the basic concepts and functions of metaclasses is already sufficient for writing Pythonic code.
Summary
This article introduced two seemingly advanced concepts in Python: generators and metaclasses. Generators provide a way of lazy iteration that can save memory and implement infinite sequences. Metaclasses, on the other hand, are classes that create classes, and by customizing metaclasses, you can inject special behavior into the class creation process.
Although the theoretical foundations of these two concepts are relatively abstract, they have many application scenarios in actual programming. By mastering them, you can write more Pythonic code. If there's anything you don't understand, feel free to continue the discussion in the comments section. Learning has no limits, so let's move forward together on the path of Python!
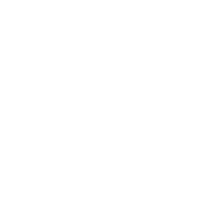
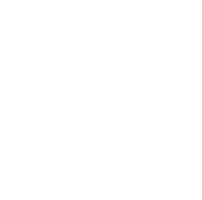