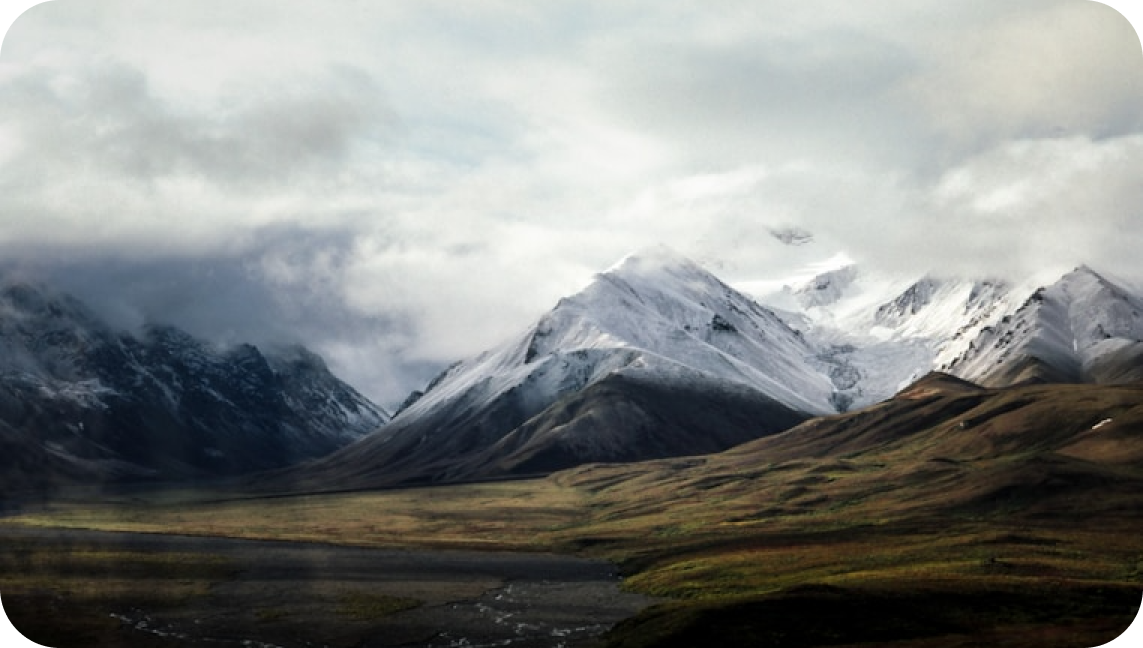
What are Generators?
Have you ever encountered a situation where you need to process a huge dataset, but have limited memory resources and can't load all the data at once? For example, reading an extremely large file, or obtaining data from a network stream? This is where generators come in handy.
Generators are a special type of function in Python that can pause execution and resume later, allowing the function to generate a series of values instead of returning all values at once. This enables generators to handle large amounts of data without exhausting memory.
How Generators Work
The working principle of generator functions is quite ingenious. When you call a generator function, it doesn't immediately execute the function body, but instead returns a generator object. Each time you call the next()
method on this generator object, the generator function executes until it encounters a yield
statement, then pauses execution and returns the value after yield
. The next time you call the next()
method, the function continues executing from where it last paused.
This mechanism allows generator functions to generate one value at a time, rather than generating all values at once. This is very useful for handling large amounts of data, as you don't need to load all the data into memory at once.
Let's look at a simple example:
def count_up_to(n):
i = 0
while i < n:
yield i
i += 1
counter = count_up_to(3)
print(next(counter)) # outputs 0
print(next(counter)) # outputs 1
print(next(counter)) # outputs 2
print(next(counter)) # raises StopIteration exception
In this example, count_up_to
is a generator function. When we call it, it returns a generator object counter
. Each time we call next(counter)
, the function executes until it encounters the yield
statement, then returns the value after yield
(i.e., i
). When i
reaches 3, the function terminates and raises a StopIteration
exception.
Application Scenarios for Generators
Generators are very useful in many scenarios, such as:
-
Reading large files: Generators can be used to read part of a file's content at a time, avoiding loading the entire file into memory.
-
Processing network stream data: When processing data obtained from a network stream, generators can be used to gradually acquire and process data without waiting for all data to arrive.
-
Generating infinite sequences: Generators can be used to generate infinite sequences, such as Fibonacci sequences, prime number sequences, etc.
-
Lazy evaluation: Generators support lazy evaluation, only calculating the next value when needed, which can improve efficiency and reduce memory usage.
-
Coroutines: Generators are the basis for implementing coroutines, which can be used for concurrent programming.
Generator Expressions
In addition to using generator functions, Python also provides a more concise syntax for creating generators, called generator expressions. Generator expressions are similar to list comprehensions, but use parentheses instead of square brackets.
For example, the following generator expression generates even numbers from 0 to 9:
even_numbers = (n for n in range(10) if n % 2 == 0)
for num in even_numbers:
print(num)
Generator expressions are usually more efficient than list comprehensions because they don't need to generate all values at once, but generate them as needed.
Summary
Generators are a powerful tool in Python that can help you efficiently handle large amounts of data while saving memory usage. Mastering the use of generators can enable you to write more elegant and efficient Python code. If you're not familiar with generators yet, try using them in your projects and experience the convenience they bring. You'll surely fall in love with this programming style!
Next
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.
Next
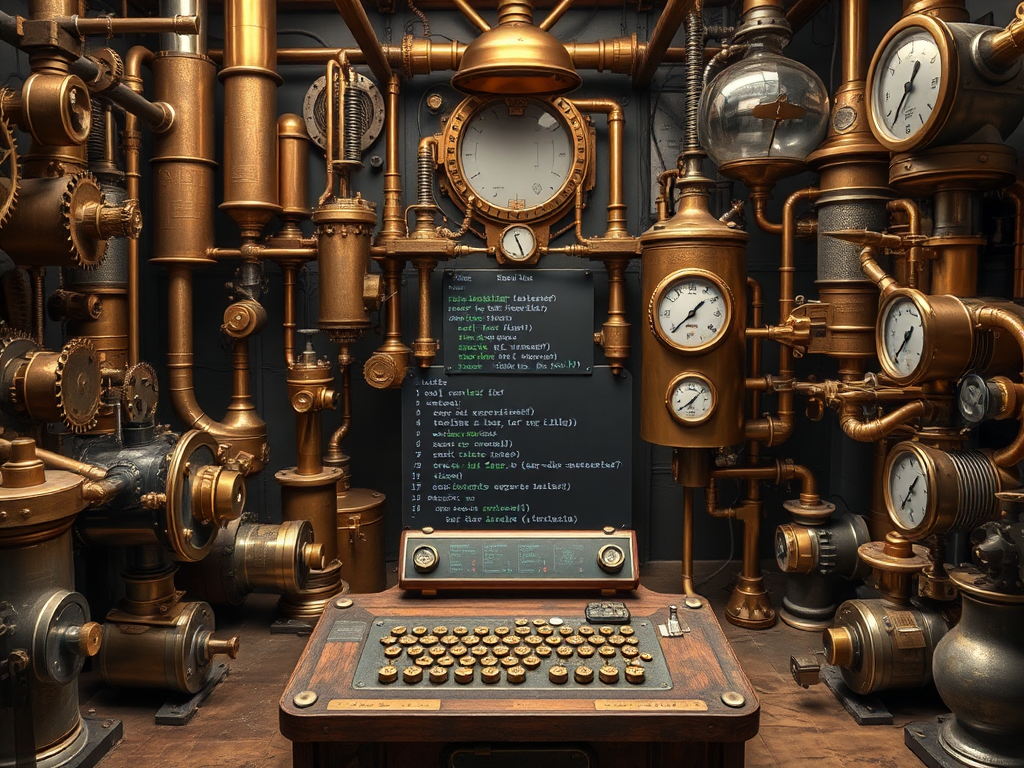
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
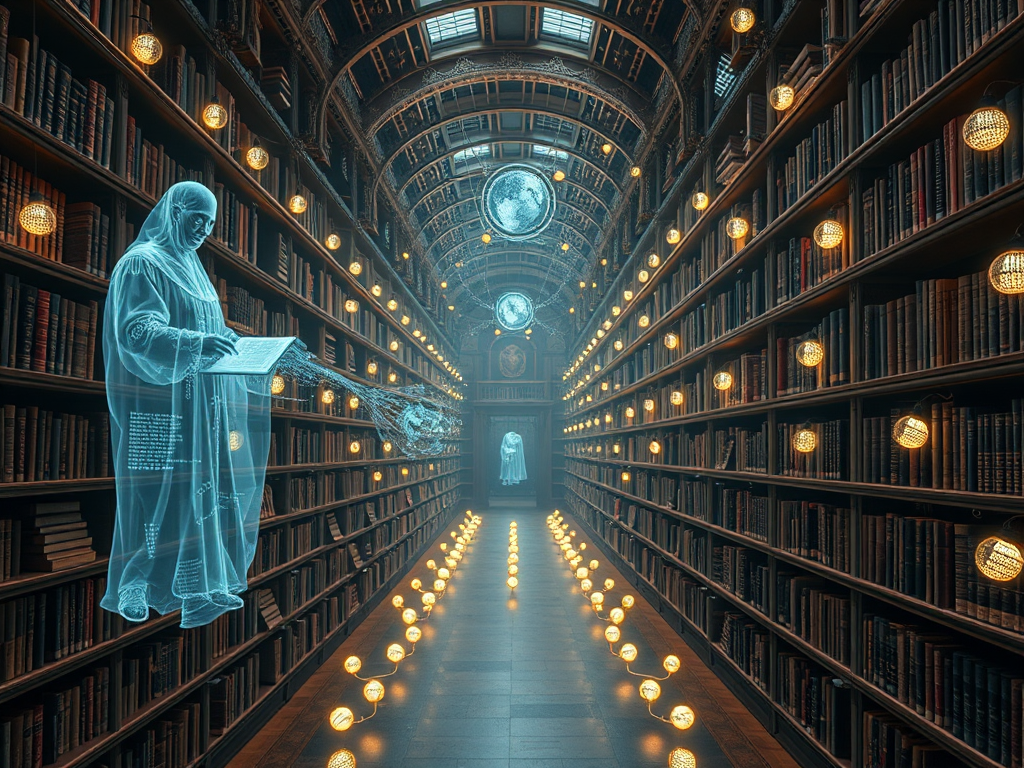
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
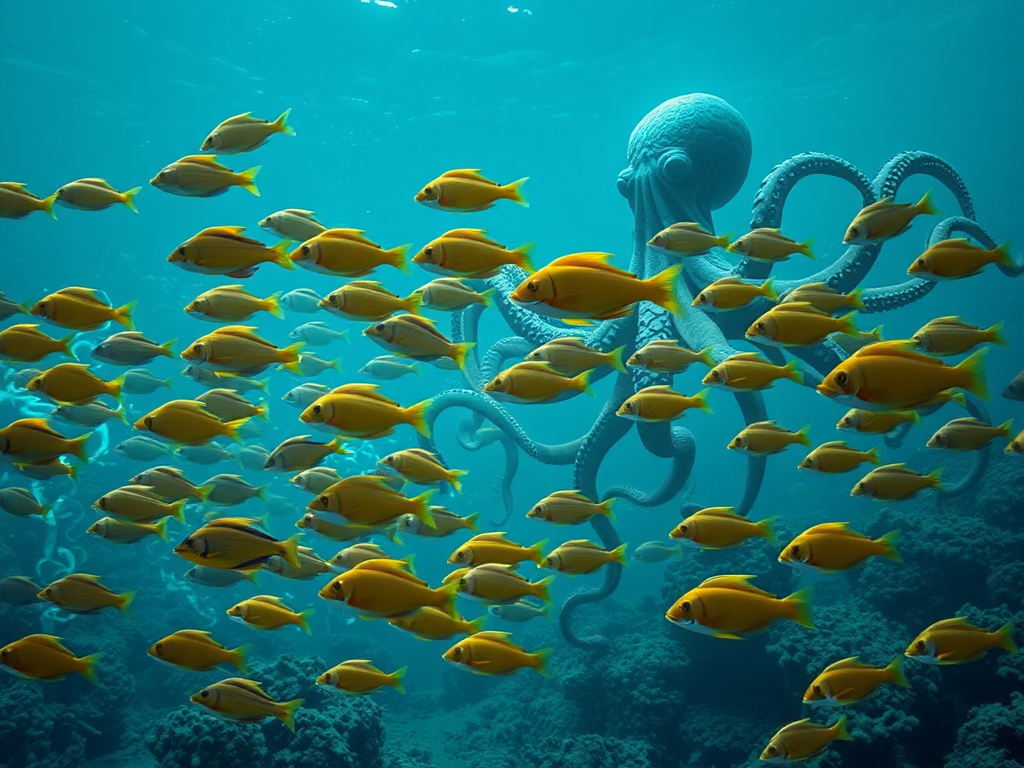
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.