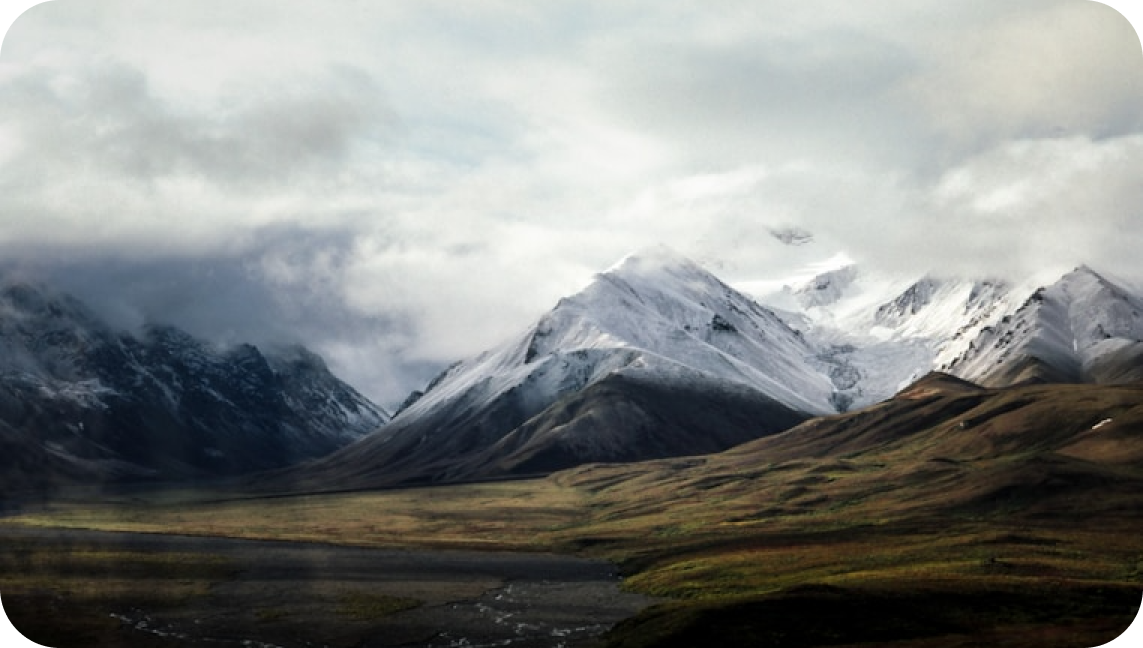
Hello everyone, we meet again! Today, let's talk about some basic but important concepts in Python, and see if you have truly mastered them. These concepts, although simple, are crucial for writing high-quality code. Let's get started!
Generators Are Efficient
Generators in Python are special functions that use the yield
keyword to produce a series of values. Unlike ordinary functions that return a list of values, generators return only one value at a time, saving memory space.
You might ask, "Why use generators? Can't we just return a list?" Well, if the data volume is small, there's indeed not much difference. But when dealing with large amounts of data, generators show their advantages. Imagine if you need to generate a list containing a billion elements, that would occupy a lot of memory! With generators, you can generate data one by one, without needing to store all the data.
The execution process of generator functions is also interesting. When you call a generator function, it doesn't immediately execute the function body, but returns a generator object. Each time you call the next()
method on this object, the function body executes until the next yield
statement, returning the produced value. You can think of yield
as a resumable return
statement.
Let's look at a simple example:
def count_up_to(n):
i = 0
while i < n:
yield i
i += 1
counter = count_up_to(3)
print(next(counter)) # outputs 0
print(next(counter)) # outputs 1
print(next(counter)) # outputs 2
print(next(counter)) # raises StopIteration exception
In this example, count_up_to
is a generator function. When we call it, it returns a generator object counter
. Each time we call next(counter)
, the function body executes until the next yield
statement, returning the corresponding value. Until the function terminates, calling next
again will raise a StopIteration
exception.
Generators are useful in many scenarios, such as returning content line by line when reading large files, calculating Fibonacci sequences, etc. You only need to care about what the next value is, without generating all values at once.
Metaclasses Are Advanced
Alright, after talking about generators, let's look at metaclasses. Metaclasses are "classes" used to create classes. It sounds a bit confusing, but it's actually an advanced metaprogramming tool in Python.
In Python, everything is an object, including classes. So, what creates classes? The answer is metaclasses. The built-in type
function in Python is actually a metaclass, and all classes are created by it.
You can understand it this way: ordinary objects are produced by instantiating classes, while class objects are produced by instantiating metaclasses.
Usually, you don't need to define metaclasses yourself. But if you want to control the class creation process, add some special behaviors to classes, then you can consider using metaclasses.
Let's look at a simple example, defining a metaclass that adds a hello
method to all created classes:
class MetaHello(type):
def __new__(cls, name, bases, attrs):
attrs['hello'] = lambda self: print("Hello from", self.__class__.__name__)
return super(MetaHello, cls).__new__(cls, name, bases, attrs)
class Person(metaclass=MetaHello):
pass
p = Person()
p.hello() # outputs "Hello from Person"
In this example, we define a MetaHello
metaclass, whose __new__
method is called when creating a class. We add a hello
method to the class in this method. Then, we create the Person
class by specifying metaclass=MetaHello
, and this class will automatically have the hello
method.
Metaclasses are one of the most advanced and complex features in Python. If you have a deep understanding of how classes work, you can use metaclasses to implement some very powerful tricks, such as automatically tracking instantiated objects, automatically generating documentation, and so on. But if you're just an ordinary Python developer, it's enough to know that metaclasses exist.
File Handling Is Common
In daily Python development, file handling is a very basic and common task. For example, reading configuration files, processing log files, parsing data files, and so on. Therefore, it's important to master some common file operation techniques.
First, let's look at how to check if a file exists. There are multiple methods to achieve this:
os.path.exists(path)
: Check if the specified path exists, can be a file or directoryos.path.isfile(path)
: Check if the specified path is a filepathlib.Path(path).exists()
: Use thePath
object in thepathlib
module to check if the path existsos.access(path, os.F_OK)
: Check if the specified path is accessible, i.e., if it exists
Different methods have their pros and cons, you can choose the appropriate one based on your actual needs. For example, if you only care about whether the file exists, then os.path.isfile
might be the best choice. If you also need to check the existence of directories, then you can use os.path.exists
.
Besides checking if a file exists, another common operation is to execute different code logic based on conditions. In Python, we have a very concise ternary conditional operator that we can use:
value = true_value if condition else false_value
This operator allows you to implement simple conditional judgments in one line of code. However, if the conditional logic is more complex, you should still use the regular if...else
statement to maintain code readability.
In addition to the ternary operator, there are some other tricks to implement ternary operations in Python, such as:
value = (false_value, true_value)[condition]
value = {True: true_value, False: false_value}[condition]
These tricks look cool, but they're not very readable. So, my suggestion is: if the logic is very simple, you can consider using the ternary operator; otherwise, just use if...else
honestly.
Modules Have Patterns
Finally, let's talk about modules in Python. Each .py
file is actually a module that can be imported and used by other Python programs.
In a module, there's a special variable __name__
that records how the module is being used. When the module is run directly, __name__
equals "__main__"
. When the module is imported by other programs, __name__
is the name of the module.
Taking advantage of this, we can add some code in the module that only executes when it's run directly, such as running test cases, starting program entry points, etc. This is usually implemented by adding the following code at the end of the module:
if __name__ == "__main__":
# Add code here that only executes when run directly
...
This approach allows the same module to be run as an independent program and also be imported and used by other programs as a library, thereby improving code reusability and modularity.
Did you gain some small insights? Generators, metaclasses, file operations, and modules are all concepts in Python basics, but mastering them is crucial for writing high-quality code. I hope that through today's sharing, you can understand and apply these concepts more deeply.
Remember, the path of programming is gradual. We need to take it step by step, continuously learning and practicing, to become true Python experts. Keep going, you can definitely do it! Happy coding~
Next
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.
Next
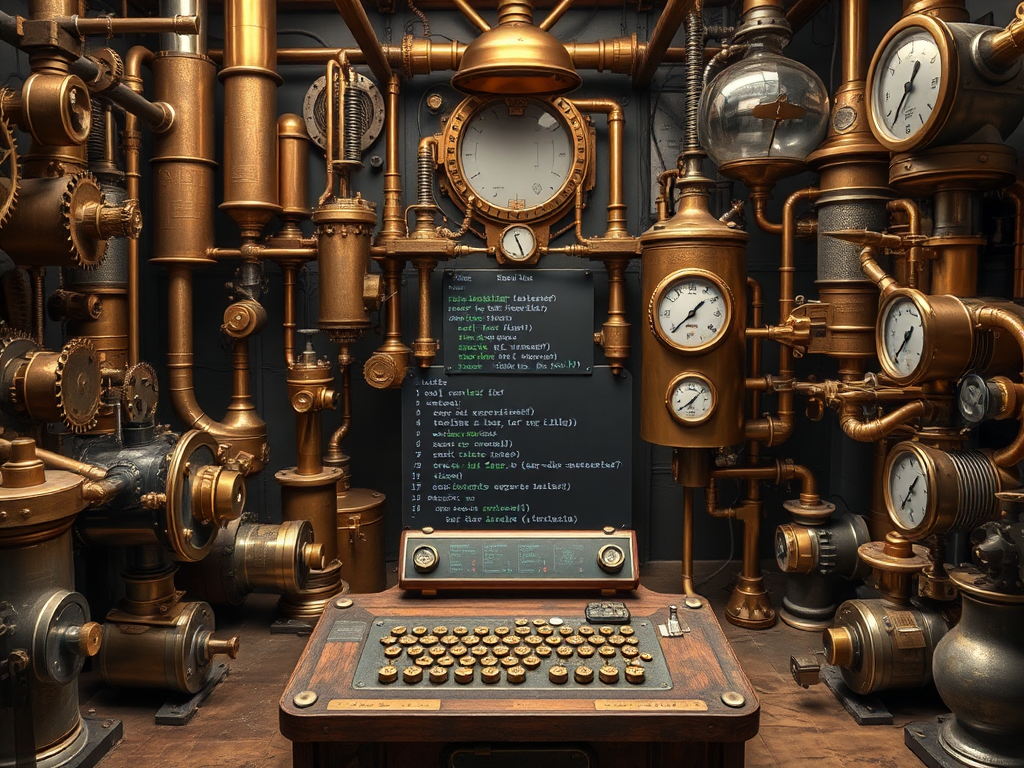
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
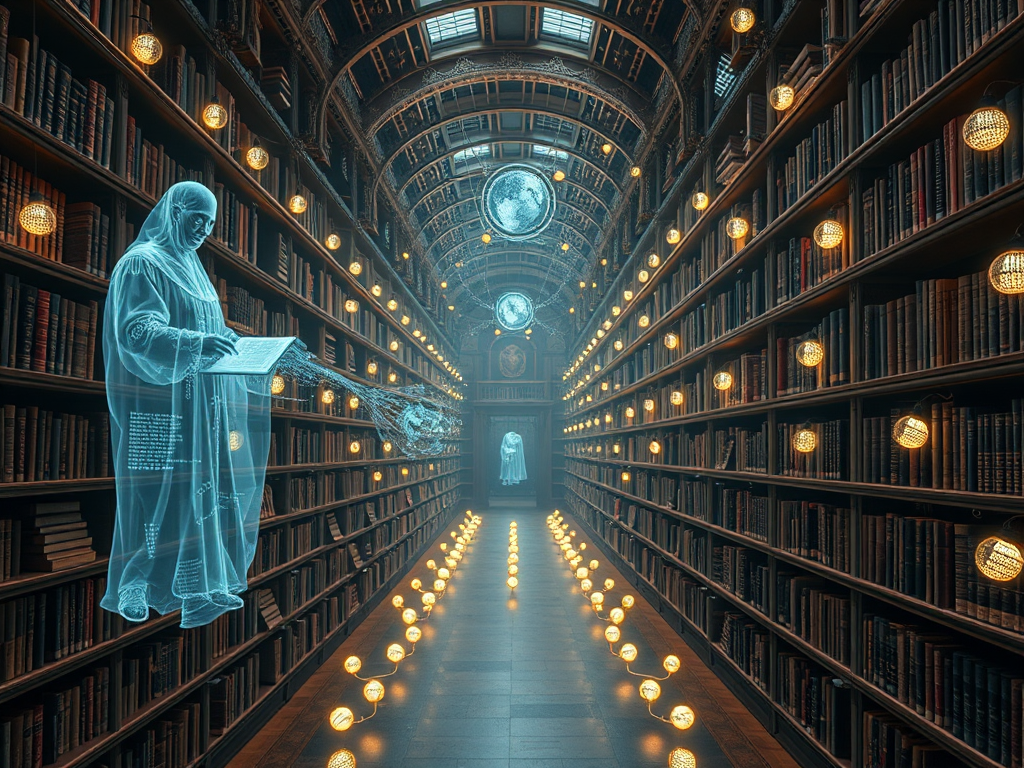
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
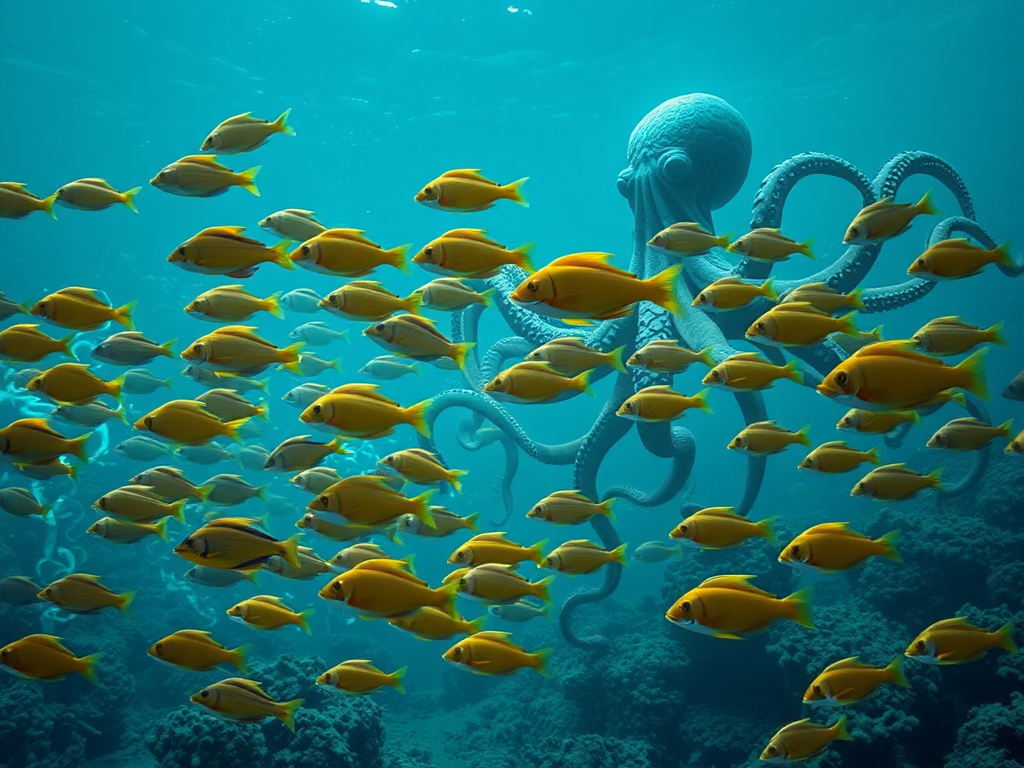
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.