What's What?
Hey, Python learning newbies! Welcome to my blog. Today, let's talk about the basics of the Python programming language.
You've probably heard of Python, but what exactly is Python? Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. It was invented by Guido van Rossum, a Dutchman, in 1989, with the first public release in 1991. Python is known for its simplicity and elegance, with clear and readable syntax that allows you to quickly get started with programming.
Python has now become one of the most popular programming languages. Whether it's web development, data analysis, artificial intelligence, system operations, or game development, Python's presence can be seen everywhere. So, mastering Python gives you a pass to various industries in the future.
Data Types
Python supports multiple data types, common ones include integers, floating-point numbers, booleans, strings, lists, and dictionaries. Let's look at a few simple examples:
Strings
name = "John Doe"
print(name.upper()) # Outputs "JOHN DOE"
print(name.lower()) # Outputs "john doe"
greeting = "Hello, " + name # String concatenation
print(greeting) # Outputs "Hello, John Doe"
age = 30
info = f"My name is {name} and I'm {age} years old." # String formatting
print(info) # Outputs "My name is John Doe and I'm 30 years old."
String case conversion, concatenation, and formatting are common operations when handling strings. You can flexibly use these techniques to make your program output more user-friendly.
Lists
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Outputs "apple"
fruits.append("orange") # Add an element
print(fruits) # Outputs ['apple', 'banana', 'cherry', 'orange']
for fruit in fruits:
print(fruit) # Output each element in the list
A list is an ordered collection, you can access elements in the list through indexing. Lists also support rich operations such as adding, removing, and sorting, which are very useful.
Dictionaries
person = {"name": "John Doe", "age": 30}
print(person["name"]) # Outputs "John Doe"
person["city"] = "New York" # Add a new key-value pair
print(person) # Outputs {'name': 'John Doe', 'age': 30, 'city': 'New York'}
for key, value in person.items():
print(f"{key}: {value}")
A dictionary is an unordered collection of key-value pairs. Values are accessed through keys rather than indices, making dictionaries particularly efficient when handling hash data.
There are more data types than these, but once you've mastered these basic types, you can start writing simple Python programs. We'll continue to delve into other data types and related operations later.
Built-in Functions and Modules
Python has many built-in functions and modules that can help us develop quickly. For example, the print()
function used in the previous examples is a built-in Python function for output.
If you want to understand how to use a certain function or module, you can use the help()
function to view the documentation:
help(print)
It will output the documentation for the print()
function, including function definition, parameter description, etc., making it clear at a glance.
If you're not sure what attributes or methods an object has, you can list them using dir()
:
import math
print(dir(math))
This will output a list of all available attributes and functions in the math
module, making it easy for you to understand and use.
The Python standard library also includes a large number of useful modules, such as the datetime
module which can conveniently handle dates and times. However, we won't go into depth today, we'll discuss it in detail after you've gotten started.
Practical Exercise
We've talked about so much theory, let's do some practical exercise! Let's complete a simple requirement: calculate the time difference between two military times.
import datetime
print("Please enter the first time (24-hour format, e.g., 0800):")
first_time = input()
print("Please enter the second time:")
second_time = input()
first_hour = int(first_time[:2])
first_minute = int(first_time[2:])
second_hour = int(second_time[:2])
second_minute = int(second_time[2:])
first_dt = datetime.datetime(2000, 1, 1, first_hour, first_minute)
second_dt = datetime.datetime(2000, 1, 1, second_hour, second_minute)
time_diff = second_dt - first_dt
print(f"The time difference is {time_diff.total_seconds() // 3600} hours {(time_diff.total_seconds() % 3600) // 60} minutes")
This code first imports the datetime
module, then prompts the user to input two military times (such as 0800, 1630, etc.). Then, through string slicing and type conversion, it converts the input strings into integer values for hours and minutes.
Next, it creates two datetime
objects, representing the two input time points. Since we only care about the time difference, the date is set to January 1, 2000 for both.
Finally, it calculates the time difference through subtraction of the two datetime
objects, and formats the result as hours and minutes for output.
Isn't it simple? You can try running it yourself to experience the process of writing a Python program. Of course, this is just a small part of Python, there are many more powerful features waiting for you to explore.
In Conclusion
Alright, that's all for today's introduction. While Python is easy to get started with, mastering it is not an overnight affair. However, as long as you persist, continuously learn and practice, I believe everyone can progress step by step and eventually become Python experts.
If you have any questions about today's content, feel free to leave a comment for discussion. Let's learn from each other and progress together! On the Python journey, we're here with you all the way.
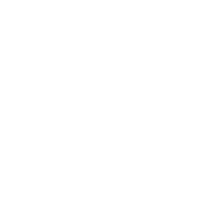
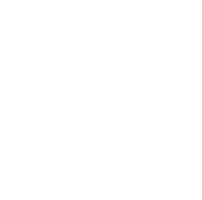