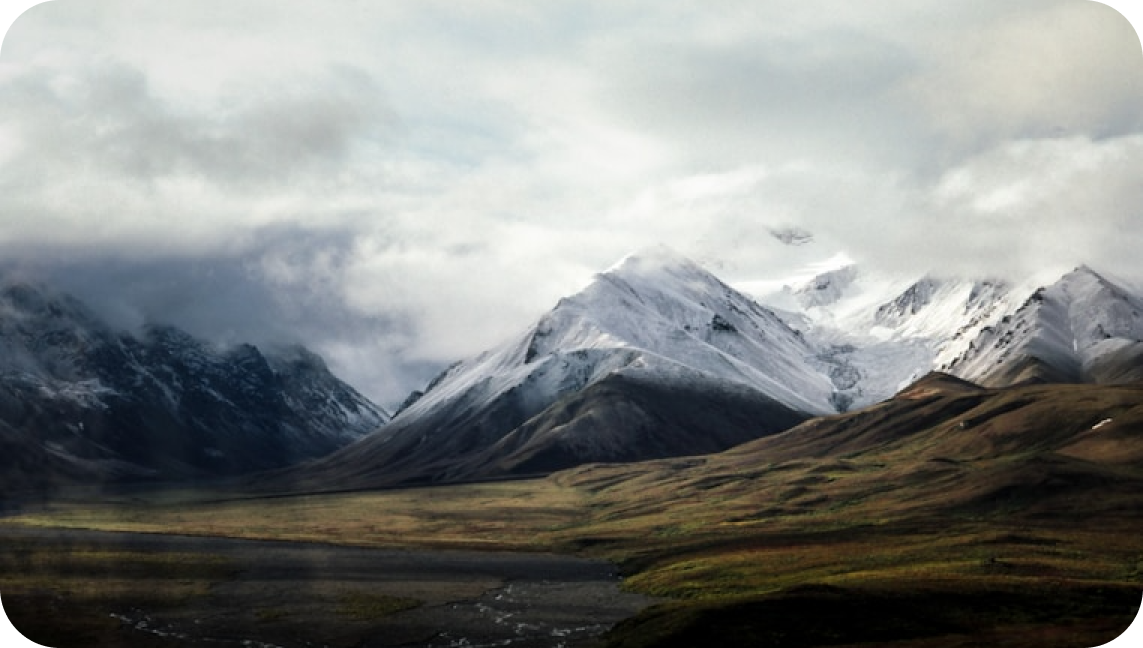
Beginner's Guide
Are you also curious about the Python programming language? Don't hesitate, let's start the learning journey together now!
First, let's look at who Python is suitable for. Whether you're a student, developer, or someone working with text or numerical processing, Python is a great choice. It uses simple English words as keywords, has concise and easy-to-understand syntax, and is very suitable for beginners in programming. Moreover, the Python interpreter is free and comes with a powerful IDE, allowing you to focus on programming itself.
Besides being easy to start with, Python's application areas are also very broad. It's often used as a supporting language, such as for automated testing and build control. If you're involved in web development, Python is an excellent choice with many outstanding web frameworks like Django and Flask. Additionally, Python excels in fields like data analysis and scientific computing.
Python's advantage also lies in its efficient development. Compared to C++ or Java, Python code is more concise and has shorter development cycles. At the same time, Python provides powerful string and list operation functions, which can efficiently process text and numerical data. This is very helpful for some special application scenarios.
Overall, Python is a highly attractive programming language. Easy to start, widely applicable, and efficient in development - these are its notable advantages. Let's start learning together now!
Time Handling
In programming, handling time is a very common requirement. For example, you might need to calculate the difference between two time points, or format the display of a certain time. In Python, we can use the datetime
module to accomplish these tasks.
I saw a popular question on Stack Overflow: how to calculate the time difference between two military times? Let's see how this highly upvoted answer does it:
import datetime
first = input("Enter the first time in military hours:")
second = input("Enter the second time in military hours:")
first_t = datetime.time(hour=int(first[0:2]), minute=int(first[2:4]))
second_t = datetime.time(hour=int(second[0:2]), minute=int(second[2:4]))
time_diff = datetime.datetime.combine(datetime.date.today(), second_t) - datetime.datetime.combine(datetime.date.today(), first_t)
print(f"{time_diff.hours} hours {time_diff.minutes} minutes")
This code first gets the input of two military times, then uses datetime.time
to convert them into time objects. Then, by calculating the difference between the two time objects, we can get the time difference. You see, although the code is not long, the steps are very clear and solve this problem in one go.
However, in practical applications, time handling can be more complex. For example, you might need to convert time formats. One of my personal tips is: if you find a time format unfamiliar, try searching for its specification online first. This way, you can quickly understand its meaning and process it correctly.
Development Environment
When it comes to Python development environments, my favorite is Jupyter Notebook. You must have used it too, right? As a web application, it allows you to write, run, and debug code in your browser, and it supports multiple formats such as rich text, images, and videos, which is very suitable for data analysis and visualization.
However, you might not know that Jupyter Notebook has a very cool feature: auto-reload! With it, you can view the effects of code modifications in real-time without restarting the Notebook. Here are the specific steps:
- Create a Python file, for example,
mytest.py
- Run in the Notebook:
%reload_ext autoreload
%autoreload 2
from mytest import MyTest
- Modify the
mytest.py
file, run step 2 again
It's that simple, you'll find that the Notebook automatically reloads your code, allowing you to view the modification effects in real-time. Isn't it convenient?
Of course, the principle of auto-reload is not complicated. However, if you're interested in its implementation, you can also study the related source code. Understanding these internal mechanisms is very helpful for better use of Jupyter.
Advanced Features
If you've already mastered the basics of Python, it's time to advance to higher realms. In this section, we'll learn some advanced features of Python that will make your code more robust and easier to maintain.
One very useful feature is the definition of generic types and collections. By using the typing
module, we can specify generic types for classes, functions, or collections. Let's look at a specific example:
from typing import Mapping, Sequence, TypeVar
T = TypeVar('T')
class MyMapping(Mapping[str, T]):
def __getitem__(self, key: str) -> T:
...
def __iter__(self) -> Sequence[str]:
...
def __len__(self) -> int:
...
Here, we define a generic class MyMapping
that inherits from Mapping[str, T]
. T
is a type variable that can be specified when using MyMapping
.
With type hints, our code is not only more readable, but IDEs can also provide better type checking and auto-completion functions, greatly improving development efficiency. This is particularly useful when writing complex collection classes or general data structures.
Of course, generic types are just one feature provided by the typing
module. It has many other powerful functions, such as type annotations, runtime type checking, and so on. If you're interested in this, you might want to study it more. I believe you'll definitely benefit a lot.
Testing and Documentation
When it comes to code quality, testing and documentation are almost the two most important aspects. Excellent code must withstand various tests and have clear documentation for others to read and maintain.
In Python, the doctest
module provides us with an elegant way of document testing. It allows you to embed some example usages and expected outputs in the docstring of your code, then automatically execute this code and verify if the output matches the expectation.
Let's look at a simple example. Suppose we have a function like this:
def add(a, b):
"""
Add two numbers.
>>> add(2, 3)
5
>>> add(1.5, 2.7)
4.2
"""
return a + b
Here, we've added two examples in the function's docstring, testing the addition of integers and floating-point numbers respectively. To run this test, just run in the command line:
python -m doctest mymodule.py
doctest
will automatically find all examples in the docstrings, execute them, and verify if the output matches the expectation.
However, there's one thing to note. When executing tests multiple times, the state of the Python interpreter may change, affecting the results of subsequent tests. In this case, we can use reload(sys)
to reset the interpreter state, ensuring that each test runs in a clean environment.
>>> a = 1
>>> a
1
>>> a = 2
>>> a
2
>>> reload(sys)
<module 'sys' (built-in)>
>>> a
1
You see, by reloading the sys
module, we successfully reset the value of a
to its initial state.
Overall, doctest
is a very powerful module that allows us to write test cases directly in the code documentation, thus ensuring that the documentation stays in sync with the implementation. Of course, besides doctest
, Python has many other testing frameworks, such as unittest
, pytest
, etc. Whichever you use, testing is a key part of improving code quality, and I hope you'll pay attention to it in your projects.
Summary
Through this blog, we've learned various aspects of Python programming together. From basics to advanced features, from time handling to development environment, from generic types to testing documentation, I've tried to explain the charm of Python with vivid language and rich examples.
As a simple and efficient language, Python has wide applications in various fields. Whether you're a programming novice or an experienced developer, you can benefit greatly from it. Let's practice together, starting with the various techniques and best practices mentioned in this article, to make our Python skills more proficient!
Of course, the road of learning is long, and this article is just a beginning. The ocean of Python is too vast, and we always have new areas to explore and discover. So, let's move forward together, arm ourselves with curiosity and thirst for knowledge, and forge ahead on this fun-filled programming path!
Next
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.
Next
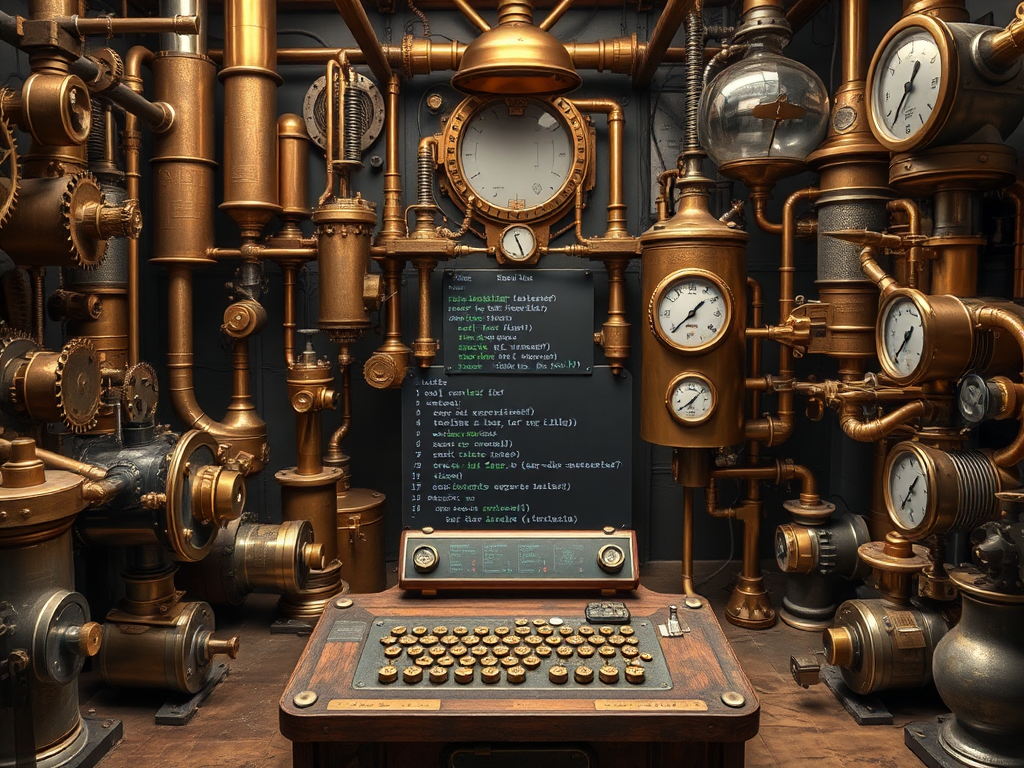
Python Basics: From Beginner to Advanced
This article delves into Python fundamentals, covering core concepts such as data types, functional programming, time handling, and exception handling. It aims
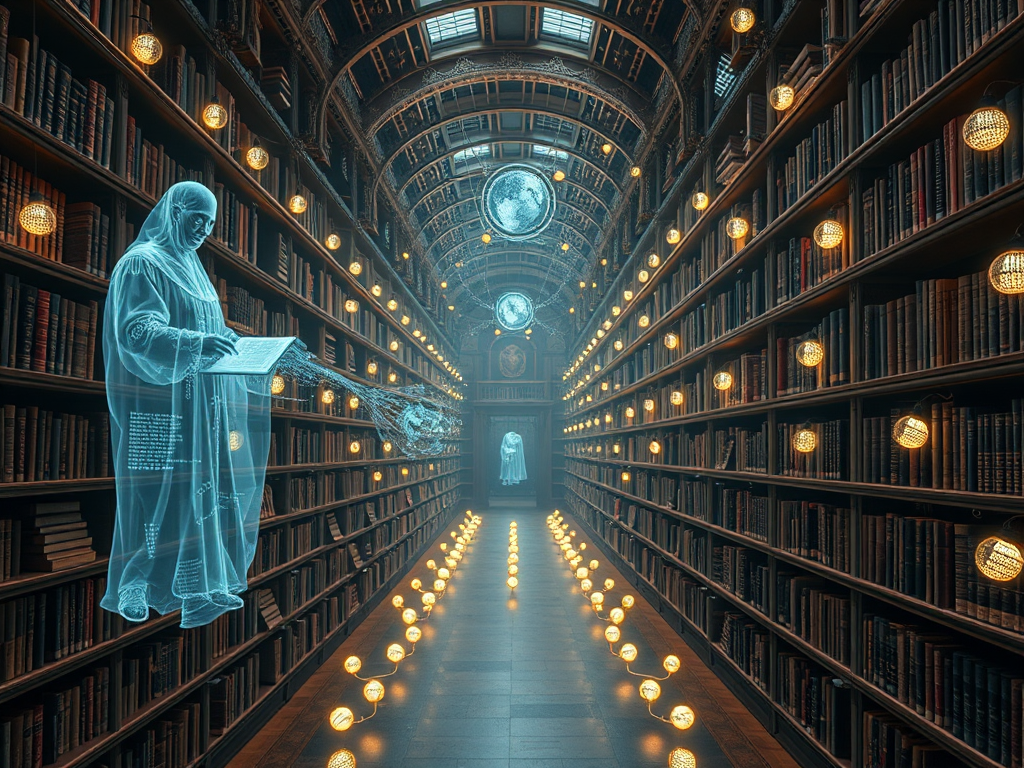
Python Object-Oriented Programming: From Beginner to Master, A Complete Guide to Classes and Objects
A comprehensive guide covering Python programming fundamentals and advanced features, including environment setup, language elements, modular programming, object-oriented programming, and domain-specific development
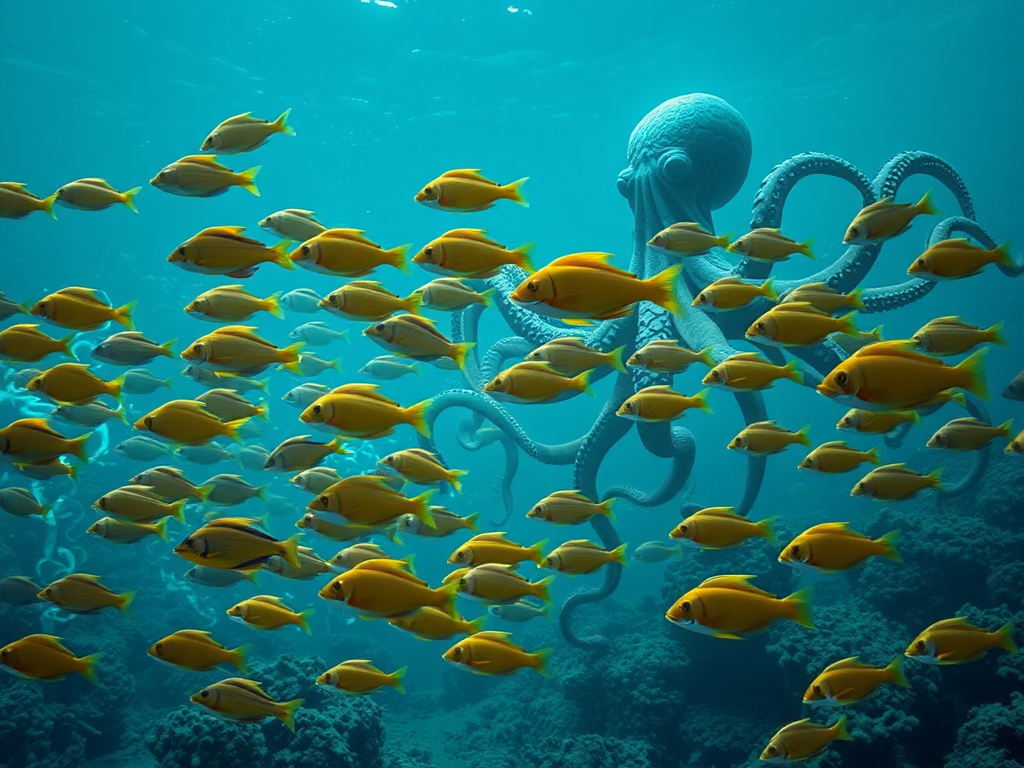
Python Programming Beginner's Guide: Master This Simple and Easy-to-Learn Programming Language
This article introduces key knowledge points for getting started with Python programming, including an introduction to Python, basic syntax, practical examples, and recommended learning resources, helping beginners quickly master this simple and widely applicable programming language.