Solid Foundations
Hey, are you also encountering some confusion and puzzlement on your Python learning journey? Don't worry, let's explore the basic concepts of Python programming together and lay a solid foundation for later practical applications!
The Data Family
Python offers various data structures for us to use, with lists and tuples being the most common. You might ask, "What's the difference between lists and tuples?"
Well, the differences between these two are mainly reflected in the following aspects:
- Mutability: Lists are mutable, meaning you can modify their elements. Tuples, on the other hand, are immutable, once created they cannot be modified.
- Syntax: Lists are defined using square brackets
[]
, likemy_list = [1, 2, 3]
. Tuples are defined using parentheses()
, likemy_tuple = (1, 2, 3)
. - Performance: Because tuples are immutable, they are faster than lists in some cases, especially in scenarios with frequent access.
- Usage: Lists are typically used for collections that need to be modified, while tuples are often used for storing fixed collections, such as when a function returns multiple values.
The Memory Manager
Alright, now that we understand data structures, how does Python manage memory? Let's take a look!
In Python, each variable is actually a reference to an object. When you create a variable, Python allocates an object in memory and makes the variable name point to the memory address of that object.
You can use the id()
function to view the memory address of an object. For example:
a = 10
print(id(a)) # Outputs the memory address of object a
So, Python manages memory through object references, making variable names point to objects in memory. Isn't that amazing?
Time for Practical Application
Now that we've understood the basic concepts, let's see how to apply Python in practical applications!
Time Master
In programming, time handling is a common requirement. For example, you might need to perform time zone conversions or calculate the difference between two times.
For time zone conversions, we can use Python's built-in datetime
module combined with the pytz
library to handle time zone issues. For example:
from datetime import datetime
import pytz
utc_time = datetime.now(pytz.utc) # Get UTC time
local_time = utc_time.astimezone(pytz.timezone('America/New_York')) # Convert to New York time zone
print(local_time)
If you need to calculate the difference between two times, you can also use the datetime
module. For instance, we can write a program to calculate the difference between two military times:
from datetime import datetime
first = input("Enter the first time in military hours (HHMM): ")
second = input("Enter the second time in military hours (HHMM): ")
first_time = datetime.strptime(first, '%H%M')
second_time = datetime.strptime(second, '%H%M')
difference = second_time - first_time
hours, remainder = divmod(difference.total_seconds(), 3600)
minutes, _ = divmod(remainder, 60)
print(f"{int(hours)} hours {int(minutes)} minutes")
This code prompts the user to input two military times, then calculates the time difference between them, and outputs the hours and minutes. Isn't that practical?
Data Master
Besides time handling, Python also has powerful capabilities in data processing. For example, we can use the Xarray library to manipulate datasets.
In Xarray, we can use the .sel()
method to index and select data based on coordinate values. For example:
import xarray as xr
ds = xr.Dataset({'temperature': (('x', 'y'), [[15, 20], [25, 30]])},
coords={'x': [1, 2], 'y': [10, 20]})
result = ds.sel(x=1, y=10)
print(result)
This code creates a dataset containing temperature data, then uses the .sel()
method to select the corresponding temperature value based on the x
and y
coordinates.
Through this approach, we can flexibly manipulate and analyze data, laying the foundation for subsequent data processing and analysis.
Summary
Alright, today we explored some basic concepts and practical applications in Python programming. From data structures, memory management, to time handling and data manipulation, I believe you now have a deeper understanding of Python.
Remember, the path of programming is gradual. As long as you persist, keep learning and practicing, one day you will become a Python master! Keep going, keep working hard, looking forward to your next exciting project!
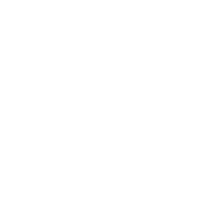
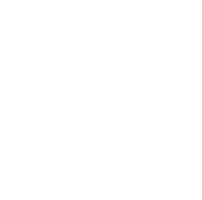