Hello, Python enthusiasts! Today let's talk about a powerful and fascinating feature in Python - List Comprehension. This feature not only makes your code more concise and elegant but also greatly improves data processing efficiency. So, what exactly is it? Let's explore together!
Introduction
List comprehension, as the name suggests, is an expression that creates lists in a concise way. It allows you to complete creation, iteration, filtering, and data transformation operations in a single line of code. Sounds magical, right? Indeed, it is!
Let's look at a basic example:
squares = [x**2 for x in range(10)]
What does this code do? It creates a list containing the squares of numbers from 0 to 9. If written with a traditional for loop, it would look like this:
squares = []
for x in range(10):
squares.append(x**2)
See how list comprehension condenses this process into one line of code! Pretty cool, right?
Syntax Analysis
So, what's the syntax structure of list comprehension? Generally, it includes these parts:
[expression for item in iterable if condition]
- expression: operation performed on each element
- item: iteration variable
- iterable: iterable object (like lists, tuples, strings, etc.)
- condition: optional filtering condition
Sounds abstract? Don't worry, let's look at some concrete examples to understand better.
Practical Examples
Basic Application
Let's say we want to create a list of all even numbers between 1 and 20, we can write it like this with list comprehension:
even_numbers = [x for x in range(1, 21) if x % 2 == 0]
print(even_numbers) # Output: [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
See that? We completed iteration, judgment, and filtering operations in one line of code. Using traditional for loops would require at least 3-4 lines.
String Processing
List comprehension is also very convenient for processing strings. For example, if we want to extract all vowels from a string:
text = "Hello, how are you today?"
vowels = [char for char in text.lower() if char in 'aeiou']
print(vowels) # Output: ['e', 'o', 'o', 'a', 'e', 'o', 'u', 'o', 'a']
This example shows how list comprehension elegantly handles strings. We first convert the string to lowercase, then check if each character is a vowel.
Nested Lists
List comprehension can also be used to handle nested lists. Suppose we have a list containing multiple sublists and want to flatten all elements into a new list:
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [num for sublist in nested_list for num in sublist]
print(flattened) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
This example might look complicated, but if you observe carefully, you'll see it's actually a combination of two for loops. The first for loop iterates through the outer list, and the second for loop iterates through each sublist.
Advanced Techniques
Conditional Expressions
List comprehensions can also use conditional expressions (also known as ternary operators). This allows us to choose different values based on conditions:
numbers = [1, 2, 3, 4, 5]
result = ['even' if x % 2 == 0 else 'odd' for x in numbers]
print(result) # Output: ['odd', 'even', 'odd', 'even', 'odd']
In this example, we decide whether to output 'even' or 'odd' based on whether the number is even or odd.
Multiple Iterations
List comprehension also supports multiple iterations. For example, we can use it to generate all possible combinations of two lists:
colors = ['red', 'green', 'blue']
sizes = ['S', 'M', 'L']
combinations = [(color, size) for color in colors for size in sizes]
print(combinations)
This example generates all combinations of colors and sizes. Using traditional nested for loops would make the code much longer!
Performance Considerations
You might wonder about the performance of list comprehension. The good news is that in most cases, list comprehension performs better than equivalent for loops. This is because list comprehension is implemented at the C level, not in pure Python code.
However, this doesn't mean you should use list comprehension everywhere. When logic becomes complex, using regular for loops might be easier to understand and maintain. Remember, code readability is often more important than minor performance improvements.
Common Pitfalls
Although list comprehension is powerful, there are some potential pitfalls to watch out for:
-
Overuse: Don't use list comprehension just for the sake of using it. If a simple for loop is clearer, use the for loop.
-
Complex Logic: If expressions or conditions become too complex, consider breaking them down into multiple lines or using regular for loops.
-
Large Datasets: For very large datasets, list comprehension might consume a lot of memory since it generates the entire list at once. In such cases, consider using generator expressions.
Generator Expressions
Speaking of generator expressions, they're similar to list comprehension but use parentheses instead of square brackets:
gen = (x**2 for x in range(10))
Generator expressions don't generate all results at once, but rather generate them as needed, which is particularly useful when dealing with large datasets.
Practical Application Scenarios
List comprehension is widely used in data processing, text processing, mathematical calculations, and many other fields. For example:
-
Data Cleaning:
python data = ['1', '2', '3', '', '4', '5', None, '6'] clean_data = [int(x) for x in data if x and x.isdigit()] print(clean_data) # Output: [1, 2, 3, 4, 5, 6]
-
File Processing:
python import os python_files = [f for f in os.listdir('.') if f.endswith('.py')]
-
Matrix Transposition:
python matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] transposed = [[row[i] for row in matrix] for i in range(len(matrix[0]))] print(transposed) # Output: [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
Summary
List comprehension is a very powerful and flexible feature in Python. It allows you to create and process lists in a concise way, improving code readability and efficiency. However, like all programming tools, the key is to use it appropriately.
When using list comprehension, always consider code readability and complexity. If list comprehension makes your code harder to understand, then using traditional for loops might be a better choice. Remember, we write code not just for machines, but more importantly for people to read and understand.
What do you think about list comprehension? Do you use it often in your daily programming? Feel free to share your thoughts and experiences in the comments!
If you want to learn more advanced Python features in depth, I recommend checking out the official documentation and some high-quality Python books. Keep learning, keep practicing, and you'll definitely become an excellent Python programmer!
That's all for today's sharing. I hope this article has been helpful to you. If you have any questions or thoughts, feel free to communicate with me anytime. The world of Python is vast and boundless, let's explore more excitement together!
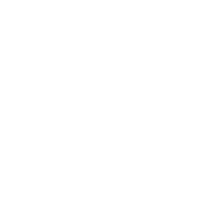
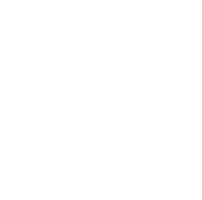