Hello, Python enthusiasts! Today we'll talk about one of the most commonly used and flexible data structures in Python - Lists. Lists are like the Swiss Army knife of the programming world - versatile and easy to use. Whether you're processing everyday data or building complex algorithms, lists are your reliable assistant. Let's dive deep into this powerful tool!
Creating Lists
First, let's look at how to create a list. In Python, creating a list is very simple - just wrap elements in square brackets []
and separate them with commas.
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
mixed = [1, "hello", 3.14, True]
See how simple it is? You can put any type of data in a list, and even mix different data types. This is the charm of Python lists - their flexibility.
I remember when I first started learning Python, I was amazed by this simple yet powerful syntax. Compared to the cumbersome array declarations in other languages, Python lists are like a breath of fresh air!
Accessing List Elements
After creating a list, we need to learn how to access its elements. In Python, we use indices to access list elements. Note that Python indices start at 0.
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
print(fruits[-1]) # Output: cherry (negative indices count from the end)
See that? We can use positive indices to access elements from the beginning of the list, and negative indices to access elements from the end. This flexible indexing allows us to conveniently access any element in the list.
Slicing Operations
Speaking of list access, we must mention Python's "slicing" operation. Slicing allows us to get a portion of the list. The syntax is list[start:end:step]
, where start
is the starting index, end
is the ending index (not included), and step
is the step size.
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(numbers[2:5]) # Output: [2, 3, 4]
print(numbers[:5]) # Output: [0, 1, 2, 3, 4]
print(numbers[5:]) # Output: [5, 6, 7, 8, 9]
print(numbers[::2]) # Output: [0, 2, 4, 6, 8]
print(numbers[::-1]) # Output: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
Isn't slicing powerful? I particularly like using [::-1]
to quickly reverse a list - this trick is very useful in many scenarios.
Modifying Lists
Python lists are mutable, which means we can modify them after creation. We can change, add, or remove elements from the list.
fruits = ["apple", "banana", "cherry"]
fruits[1] = "kiwi"
print(fruits) # Output: ['apple', 'kiwi', 'cherry']
fruits.append("orange")
print(fruits) # Output: ['apple', 'kiwi', 'cherry', 'orange']
fruits.insert(1, "mango")
print(fruits) # Output: ['apple', 'mango', 'kiwi', 'cherry', 'orange']
fruits.remove("kiwi")
print(fruits) # Output: ['apple', 'mango', 'cherry', 'orange']
popped = fruits.pop()
print(popped) # Output: orange
print(fruits) # Output: ['apple', 'mango', 'cherry']
These operations allow us to flexibly manage data in lists. I find the append()
and pop()
methods particularly interesting - they allow lists to be used like stacks, which is very convenient when solving certain algorithmic problems.
List Comprehensions
When talking about the power of Python lists, how can we not mention list comprehensions? List comprehensions are a very elegant and powerful feature in Python that allows us to create complex lists with just one line of code.
squares = [x**2 for x in range(1, 11)]
print(squares) # Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
evens = [x for x in range(20) if x % 2 == 0]
print(evens) # Output: [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
words = ["hello", "world", "python", "programming"]
lengths = [len(word) for word in words]
print(lengths) # Output: [5, 5, 6, 11]
List comprehensions are truly one of my favorite features in Python. They not only make code more concise but also perform excellently when handling large amounts of data. Every time I solve a problem using list comprehensions, I'm amazed by Python's elegance and power.
Common List Methods
Python lists have many other useful methods. Let's look at some of them:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3]
numbers.sort()
print(numbers) # Output: [1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
numbers.reverse()
print(numbers) # Output: [9, 6, 5, 5, 4, 3, 3, 2, 1, 1]
print(numbers.count(1)) # Output: 2
print(numbers.index(5)) # Output: 2
numbers.extend([7, 8])
print(numbers) # Output: [9, 6, 5, 5, 4, 3, 3, 2, 1, 1, 7, 8]
These methods allow us to easily manipulate and analyze data in lists. I particularly like the sort()
method - it allows us to quickly sort lists, which is very useful when handling large amounts of data.
Performance Considerations
At this point, we must mention the performance aspects of lists. Although lists are very flexible and convenient, they might not be the best choice in certain situations.
For example, if you need to frequently insert or delete elements at the beginning of a list, the performance might be poor because these operations require moving all other elements in the list. In such cases, using collections.deque
might be a better choice.
from collections import deque
queue = deque(["Eric", "John", "Michael"])
queue.appendleft("Terry") # Add element on the left
queue.popleft() # Remove element from the left
Also, if your list contains many duplicate elements and you frequently need to check if elements exist, using a set might be more efficient.
numbers = [1, 2, 3, 4, 5, 1, 2, 3]
number_set = set(numbers)
print(3 in number_set) # This is faster than 3 in numbers
In actual programming, choosing the appropriate data structure is very important for program performance. We should choose the most suitable data structure based on specific use cases.
List Application Scenarios
Lists are widely used in Python programming. Here are some scenarios where I frequently use lists:
- Data Processing: When we read data from files or databases, we usually store the data in lists for processing.
numbers = [int(line.strip()) for line in open('numbers.txt')]
average = sum(numbers) / len(numbers)
print(f"The average is: {average}")
- Task Queues: Lists can be used as simple task queues where we add tasks to the end of the list and take tasks from the beginning.
tasks = ["Task1", "Task2", "Task3"]
while tasks:
current_task = tasks.pop(0)
print(f"Executing: {current_task}")
- Caching: Lists can be used as simple caches to store recently used items.
cache = []
def get_item(item):
if item in cache:
return cache[cache.index(item)]
else:
# Assume this is a time-consuming operation
result = expensive_operation(item)
cache.append(result)
if len(cache) > 10: # Only keep the 10 most recent items
cache.pop(0)
return result
- Multidimensional Data: Lists of lists can be used to represent two-dimensional or multidimensional data.
board = [
['X', 'O', 'X'],
['O', 'X', 'O'],
['O', 'X', 'X']
]
print(board[1][1]) # Output center position value: X
These are just the tip of the iceberg for list applications. In actual programming, you'll find lists are almost everywhere - their flexibility allows them to adapt to various scenarios.
Summary
Today, we've deeply explored Python lists. From creating and accessing lists, to modifying and manipulating them, to list comprehensions and common methods, we've covered all aspects of lists. We've also discussed performance considerations and some common application scenarios.
Lists are truly powerful and flexible data structures in Python. They're simple to use yet powerful, and are indispensable tools in Python programming. I hope through today's sharing, you've gained a deeper understanding of Python lists and can better utilize them in your future programming.
Remember, programming is like cooking in a kitchen - lists are your cutting board, and various data are your ingredients. Mastering lists will help you navigate the Python world with ease, creating all kinds of delicious "code meals."
So, what are your insights or questions about Python lists? Feel free to leave comments, let's discuss and grow together. The world of programming is vast - let's explore the mysteries of Python together!
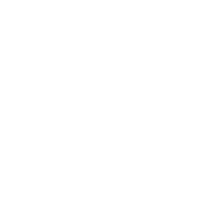
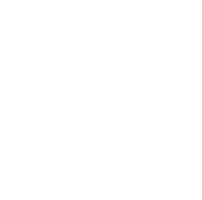