Hello, dear Python learner! Today, let's talk about a very useful data structure in Python—lists. Lists are like a versatile tool in Python programming; they're flexible, powerful, and can help us easily manage and manipulate data. So, let's dive into this amazing data structure!
Getting Acquainted
Do you remember the first time you encountered Python lists? I was captivated by their simplicity and power. Lists are like magical containers that can hold all sorts of data. Whether it's numbers, strings, or other objects, lists handle them with ease.
Let's see how to create a list:
my_list = [1, 2, 3, 4, 5]
mixed_list = [1, "hello", 3.14, True]
See, it's quite simple! Just use square brackets []
to enclose the elements you want, separated by commas.
Endless Applications
Index Access
Each element in a list has its position, called an index. In Python, indexing starts at 0. For example:
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Output: apple
print(fruits[2]) # Output: cherry
You see, we can easily access any element in the list by its index. It's like labeling each element and calling it by its number.
Slicing
But wait, what if I want to get multiple elements at once? Don't worry, Python lists provide slicing:
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
print(numbers[2:5]) # Output: [2, 3, 4]
print(numbers[:3]) # Output: [0, 1, 2]
print(numbers[7:]) # Output: [7, 8, 9]
print(numbers[::2]) # Output: [0, 2, 4, 6, 8]
Slicing is like slicing a cake; you can specify start and end positions, even set the step. This feature is especially useful when handling large amounts of data, allowing you to extract exactly what you need.
Ever-Changing
Adding Elements
The charm of lists is also in their mutability. We can add new elements at any time:
todo_list = ["Learn Python", "Write code"]
todo_list.append("Take a break")
print(todo_list) # Output: ['Learn Python', 'Write code', 'Take a break']
todo_list.insert(1, "Have a coffee")
print(todo_list) # Output: ['Learn Python', 'Have a coffee', 'Write code', 'Take a break']
The append()
method adds elements to the end of the list, while insert()
allows you to insert elements at a specified position. It's like adding new tasks to your to-do list anytime.
Removing Elements
Of course, sometimes we need to remove elements from a list:
fruits = ["apple", "banana", "cherry", "date"]
removed_fruit = fruits.pop(1)
print(removed_fruit) # Output: banana
print(fruits) # Output: ['apple', 'cherry', 'date']
fruits.remove("cherry")
print(fruits) # Output: ['apple', 'date']
The pop()
method not only deletes the element at a specified position but also returns it. The remove()
method deletes a specified element directly. It's like crossing items off your shopping list once you've bought them.
Sorting and Organizing
Sometimes, we need to sort elements in a list:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3]
numbers.sort()
print(numbers) # Output: [1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
fruits = ["banana", "apple", "cherry", "date"]
sorted_fruits = sorted(fruits)
print(sorted_fruits) # Output: ['apple', 'banana', 'cherry', 'date']
print(fruits) # Output: ['banana', 'apple', 'cherry', 'date']
The sort()
method modifies the original list, while the sorted()
function returns a new sorted list, leaving the original unchanged. It's like organizing your bookshelf; you can choose to rearrange the books directly or sort them in a specific order.
List Comprehensions
Speaking of Python lists, how can we not mention list comprehensions? These are gems in Python, allowing us to create new lists concisely:
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
even_numbers = [x for x in range(20) if x % 2 == 0]
print(even_numbers) # Output: [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
List comprehensions are like magical formulas, allowing us to complete creation, iteration, and filtering in one line. They not only make the code more concise but are often more efficient than traditional for loops when handling large data sets.
Practical Application
Let's look at a practical example of analyzing a group of student scores:
scores = [85, 92, 78, 65, 88, 72, 90, 81, 70, 93]
average_score = sum(scores) / len(scores)
print(f"Average Score: {average_score:.2f}")
highest_score = max(scores)
lowest_score = min(scores)
print(f"Highest Score: {highest_score}, Lowest Score: {lowest_score}")
pass_count = len([score for score in scores if score >= 60])
print(f"Pass Count: {pass_count}")
sorted_scores = sorted(scores, reverse=True)
print(f"Sorted Scores (High to Low): {sorted_scores}")
In this example, we use several list features and related functions to analyze student scores. We calculate the average, find the highest and lowest scores, count the number of passing scores, and sort the scores. By flexibly using lists, we can easily complete these data analysis tasks.
In-Depth Considerations
While lists are powerful, there are a few details to keep in mind:
-
Lists are mutable, meaning we can directly modify elements. This gives us great flexibility, but be careful not to unintentionally change data that shouldn't be modified.
-
For large data sets, list operations may be slow. If you need to frequently insert or delete elements at the beginning or middle of a sequence, consider using
collections.deque
. -
If your list contains only numbers, consider using
numpy
arrays, which are more efficient for numerical computations. -
While list comprehensions are powerful, overuse can affect code readability. In complex situations, using conventional for loops may be clearer.
Conclusion
Python lists are like treasure chests, full of treasures waiting to be discovered. Through today's exploration, do you have a new understanding of lists? Have you thought about how you can use lists in your projects?
Remember, the charm of programming lies in continuous learning and practice. I hope this article inspires you to further explore Python lists. If you have any questions or ideas, feel free to leave a comment, and let's discuss and learn together!
So, are you ready to embark on your Python list adventure? Let's dive into the ocean of code and discover more Python mysteries!
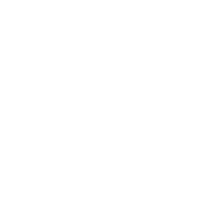
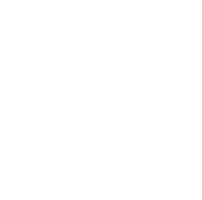