Data Basics
The most fundamental part of Python programming is data types and data structures. As a beginner, you might be unfamiliar with them, but don't worry, follow along with me step by step, and you'll soon become proficient!
List Comprehension
When it comes to list comprehension, you might think the name sounds profound. However, listen to this example and you'll understand:
squares = [x**2 for x in range(10)]
Look, this single line of code creates a list containing the squares of numbers from 0 to 9: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
. Isn't it super concise? How many lines of code would it take to write the same functionality using a loop?
I personally love this list comprehension syntax, it's both simple and less prone to errors. If you fall in love with it too, use it more often. Many data transformation operations in Python can be expressed this way.
Dictionaries are Useful
Besides lists, another super practical data type in Python is the dictionary. As the name suggests, it's like a dictionary, storing various key-value pairs:
info = {
"name": "Xiao Ming",
"age": 25,
"gender": "male"
}
Here we created an info
dictionary, storing Xiao Ming's name, age, and gender information. To access the values, you can simply use the key as an index:
print(info["name"]) # Output: Xiao Ming
Dictionaries come in handy in many scenarios, such as caching data, counting word occurrences, and so on. Master its usage, and you've taken another step forward in your programming journey!
Functions and Exceptions
Besides the data itself, we also need to learn how to process and manipulate data. Functions and exception handling are two important concepts.
What are Functions Used For
Many of you have probably learned some programming languages, so the concept of functions shouldn't be unfamiliar. The syntax for defining functions in Python is as follows:
def square(x):
return x ** 2
This square
function takes a parameter x
and returns its square. To call it, you write:
result = square(4)
print(result) # Outputs 16
What's the advantage of functions? The biggest benefit is code reuse. Once a function is defined, you can call this logic repeatedly anywhere in the program, saving the trouble of rewriting. This not only improves development efficiency but also benefits code maintainability.
So when writing code, if you find repeated logic, encapsulate it into a function. You can reuse it in the future, killing multiple birds with one stone!
Be Kind to Exceptions
During programming, you might occasionally encounter unexpected situations, such as user input problems, files that can't be opened, and so on. If not handled, the program will directly report an error and terminate. This is obviously not what we want to see.
Fortunately, Python provides the try/except
statement to catch and handle these exceptional situations:
try:
result = 10 / 0 # This will raise an exception
except ZeroDivisionError:
print("You can't divide by zero")
In the above example, we first try to perform the illegal operation 10 / 0
. Of course, it will raise a ZeroDivisionError
exception. We caught this exception through the except
statement and output a friendly prompt message.
This way, even if an exception occurs, the program won't crash and can continue running. For users, the experience will also be more friendly. So when programming, be sure to handle exceptional situations to make your program run more robustly.
Time Machine
As a Python programmer, you occasionally need to handle date and time data. This skill can be useful in many scenarios, such as log analysis, task scheduling, and so on.
This is How You Calculate Time Differences
Here's an interesting interview question, let's start with this example:
from datetime import datetime
first = input("Please enter the first time (24-hour format, e.g., 0830):")
second = input("Please enter the second time:")
first_time = datetime.strptime(first, "%H%M")
second_time = datetime.strptime(second, "%H%M")
time_difference = second_time - first_time
hours = abs(time_difference.seconds // 3600)
minutes = abs((time_difference.seconds % 3600) // 60)
print(f"The time difference is {hours} hours {minutes} minutes")
The purpose of this code is to calculate the hours and minutes difference between two input times.
The key is using the datetime
module to convert string times to time objects, then through the difference between two time objects, you can calculate the difference in seconds.
Finally, dividing the seconds by 3600 and 60 respectively, you can get the difference in hours and minutes.
Aren't you a bit shocked? It turns out calculating time differences is so simple! Python's date and time handling is really user-friendly.
Time Travel
Of course, besides calculating time differences, Python's datetime
functionality goes far beyond this. You can add or subtract a period of time, format time output, and so on, which has a wide range of applications. In the future, whether you're processing logs or doing data analysis, time data will be a piece of cake.
Friends, let's start learning Python programming from these basic concepts today and progress step by step! Remember, whether it's data types, functions, exceptions, or time processing, they are all cornerstones of programming. I hope that through my explanation, you can lay a good foundation and pave the way for your future learning journey. Come on, let's advance towards the goal of becoming programming experts together!
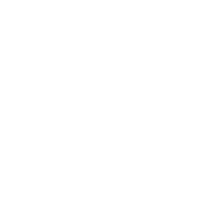
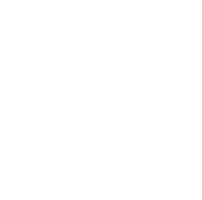