Hello, dear readers! Today, we're going on a wonderful journey to explore the magical world of Python programming. Are you curious about coding and can't wait to start? Don't rush; let's take it step by step and dive into the exciting world of Python!
Getting to Know Python
Do you remember how you felt when you first heard the name "Python"? Did it sound cool and mysterious? Actually, Python isn’t some unattainable entity; it's like an old friend, always ready to help us solve problems.
Python was created by Guido van Rossum in 1991. Did you know? The name Python doesn’t come from the slithering reptile but from Guido's love for the British comedy "Monty Python’s Flying Circus." Doesn’t that make Python feel a lot more approachable?
So, what makes Python special? First, its syntax is very simple and clear, almost like reading English. Does it remind you of those complex math formulas? In contrast, Python is like a patient teacher, explaining how to solve problems in the simplest language.
Secondly, Python is a general-purpose language, meaning it can be used in almost any field. Whether you want to develop websites, analyze data, or study artificial intelligence, Python can be your helpful assistant. Imagine holding a Swiss Army knife; Python is that multifunctional tool ready for any situation.
Embarking on the Journey
Now that we have a basic understanding of Python, are you eager to write your first program? Hold on; before we start, let's get to know Python's basic data types, like preparing for a trip.
Numbers: The Foundation of Python
In Python, numbers are one of the most basic data types. You can think of them as building blocks for constructing complex programs. There are mainly two types of numbers in Python: integers (int) and floating-point numbers (float).
Integers are the whole numbers we use in daily life, like 1, 100, -50, etc. Floating-point numbers are numbers with decimal points, such as 3.14, -0.01, etc.
Let's look at a simple example:
age = 25
height = 1.75
print(f"I am {age} years old and {height} meters tall.")
See, isn’t it simple? We just defined two variables, one with an integer age and the other with a floating-point height, and then printed them with a simple print statement.
Did you notice we used a special string formatting method? This is the f-string introduced in Python 3.6. It allows us to embed variables directly within a string, making the code more concise and readable. Doesn’t Python feel thoughtful?
Strings: The Art of Text
Next, let's get to know strings. In Python, a string is a series of characters enclosed in quotes. It can be single, double, or even triple quotes.
name = 'Alice'
greeting = "Hello, World!"
long_text = '''This is a
multi-line
long text.'''
print(name)
print(greeting)
print(long_text)
See, we can define strings in different ways. Single and double quotes are equivalent in Python, so you can choose according to personal preference. Triple quotes allow us to define multi-line strings, which is especially useful for handling long texts.
Strings have many interesting operations, like concatenation and slicing. Let's look at an example:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(full_name) # Output: John Doe
print(full_name[0:4]) # Output: John
print(full_name[-3:]) # Output: Doe
See? We can use the plus sign to concatenate strings and brackets with colons to slice them. Slicing allows us to extract a part of the string, which is very convenient.
Booleans: The World of True and False
In Python, booleans only have two values: True and False. You can think of them as switches, either on (True) or off (False).
is_python_fun = True
is_coding_hard = False
print(is_python_fun) # Output: True
print(is_coding_hard) # Output: False
Booleans are often used in conditional statements and loops, which we’ll cover in detail later.
Lists: Containers of Data
Lists are one of the most commonly used data structures in Python. You can think of them as boxes that can hold various items.
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
mixed = ["hello", 42, True, 3.14]
print(fruits)
print(numbers)
print(mixed)
Lists are very flexible; you can store any type of data in them, even nested lists. We can access elements in the list using indices:
print(fruits[0]) # Output: apple
print(numbers[-1]) # Output: 5
Lists have many useful methods, such as append() to add elements and pop() to remove elements. We’ll cover these in more detail in later chapters.
Dictionaries: The World of Key-Value Pairs
Dictionaries are another very useful data structure. You can think of them as a real dictionary, where each word (key) has a definition (value).
person = {
"name": "Alice",
"age": 30,
"city": "New York"
}
print(person["name"]) # Output: Alice
print(person.get("age")) # Output: 30
Dictionaries are defined using curly braces, with each key-value pair separated by a colon and different pairs separated by commas. We can use brackets or the get() method to access values in a dictionary.
Control Flow: The Brain of the Program
Now that we understand Python's basic data types, let's see how to control the flow of a program. This is like giving our program a brain, allowing it to make different decisions based on varying conditions.
Conditional Statements: if-elif-else
Conditional statements allow us to execute different code based on conditions. It’s like deciding whether to take an umbrella based on the weather.
weather = "sunny"
if weather == "rainy":
print("Remember to bring an umbrella!")
elif weather == "sunny":
print("Don't forget your sunglasses!")
else:
print("Enjoy your day!")
In this example, we make different decisions based on the weather. The if statement checks the first condition, executes the corresponding code block if true, or moves to the elif (short for else if) condition if false. If all conditions are false, the code block after else executes.
Loops: The Art of Repetition
Loops allow us to repeat certain operations. There are two main types of loops in Python: for loops and while loops.
For loops are usually used to iterate over a sequence (like a list or string):
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(f"I like {fruit}")
This code iterates over each element in the fruits list and prints it.
While loops execute as long as a condition is true:
count = 0
while count < 5:
print(count)
count += 1
This code prints the numbers 0 to 4.
Functions: The Building Blocks of Code
Functions are reusable blocks of code. You can think of them as building blocks to construct more complex programs.
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
In this example, we define a function named greet that takes a parameter name and returns a greeting.
Functions make our code more modular and easier to maintain. Imagine needing the same code in multiple places; you can encapsulate it in a function and call it wherever needed instead of copying and pasting.
Modules: Python’s Supermarket
Modules are files containing Python code. You can think of them as different departments in a supermarket, each providing different goods (functions).
Python has many built-in modules, like the math module for various mathematical functions and the random module for generating random numbers. We can use the import statement to import these modules:
import math
print(math.pi) # Output: 3.141592653589793
print(math.sqrt(16)) # Output: 4.0
Besides built-in modules, Python has thousands of third-party modules that can be installed using pip, Python’s package manager. These modules greatly extend Python’s capabilities, allowing us to easily accomplish complex tasks.
Conclusion: The Infinite Possibilities of Python
Well, dear readers, our basic journey into Python has temporarily come to an end. But remember, this is just the beginning. The world of Python is vast, and what we've learned today is just the tip of the iceberg.
Have you noticed how simple and elegant Python’s syntax is? It’s like an artistic language that lets us express complex ideas in the simplest way. Moreover, Python’s ecosystem is so rich that whatever you want to do, you'll find the right tools and libraries.
I remember the excitement when I first used Python. When I wrote my first "Hello, World!" program, I knew I had fallen in love with this language. I hope you can feel that excitement and enjoy the fun and sense of accomplishment in programming.
Next, I suggest you write more code and practice. Try solving some practical problems with what we learned today. For example, you might write a simple calculator program or a number-guessing game. Remember, the best way to learn programming is by doing.
Finally, I want to say, don’t be afraid of making mistakes. Every programmer encounters errors and bugs; it's an inevitable part of learning. When you face problems, don’t get discouraged. Try to understand the error messages, consult documentation, or seek help from others. Remember, solving each problem brings you closer to becoming a great programmer.
So, are you ready to continue your Python journey? There is more exciting content ahead waiting for us to explore. Let’s swim in the world of Python and create amazing things together!
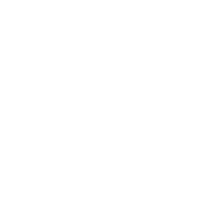
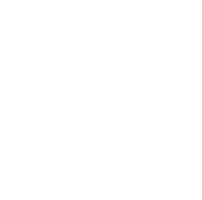