Have you ever wondered how to make your Python code more concise and readable while retaining its powerful functionality? If so, you need to learn about Python decorators! This magical feature can take your code to the next level, and today we'll explore how.
Introduction to Decorators
What is a decorator? Simply put, it's a function used to modify the functionality of other functions. Sounds a bit abstract? Don't worry, let's understand it through an example.
Imagine you have a simple function to print a greeting:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice")) # Output: Hello, Alice!
Now, if you want to log every time this function is called, what would you do? You might modify the function like this:
def greet(name):
print("Function was called") # Add log
return f"Hello, {name}!"
print(greet("Alice"))
But what if you have many functions that need logging? Do you have to modify each one? This is where decorators come in handy!
The Magic of Decorators
Let's see how to achieve the same functionality using decorators:
def log_decorator(func):
def wrapper(*args, **kwargs):
print("Function was called")
return func(*args, **kwargs)
return wrapper
@log_decorator
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
See that? We just need to add @log_decorator
above the original function to easily add logging without modifying the original function's code. That's the magic of decorators!
How Decorators Work
You might ask, how is this achieved? Actually, the @log_decorator
syntax sugar is equivalent to:
greet = log_decorator(greet)
What does this line do? It passes the greet
function as an argument to the log_decorator
function, and then replaces the original greet
function with the new function returned. So, each time greet
is called, it's actually calling the wrapper
function, which adds the log before calling the original greet
function.
Isn't it amazing? That's the charm of Python!
Parameterized Decorators
Decorators can also have parameters, making them even more flexible. For example, we can let the log decorator support custom log messages:
def log_decorator(message):
def decorator(func):
def wrapper(*args, **kwargs):
print(message)
return func(*args, **kwargs)
return wrapper
return decorator
@log_decorator("greet function was called")
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Here, we define a parameterized decorator. It accepts a message
parameter and returns a decorator function. This way, we can customize different log messages for different functions.
Using Multiple Decorators
Did you know? We can use multiple decorators at the same time! They execute from top to bottom. Check out this example:
def bold(func):
def wrapper(*args, **kwargs):
return f"<b>{func(*args, **kwargs)}</b>"
return wrapper
def italic(func):
def wrapper(*args, **kwargs):
return f"<i>{func(*args, **kwargs)}</i>"
return wrapper
@bold
@italic
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
In this example, the greet
function is first decorated by the italic
decorator, and then by the bold
decorator. The result is that the returned string is first wrapped in italic tags, then in bold tags.
Practical Uses of Decorators
Decorators have many uses in real-world development. Besides logging, they can be used for:
- Performance testing: Measuring function execution time
- Permission validation: Checking if a user is authorized to execute a function
- Caching: Storing function results to avoid recalculating
- Error handling: Uniformly handling exceptions a function might throw
Let's look at an example of measuring function execution time:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} execution time: {end_time - start_time:.5f} seconds")
return result
return wrapper
@timing_decorator
def slow_function():
time.sleep(2)
return "Function completed"
print(slow_function())
In this example, we create a timing_decorator
that measures the execution time of the decorated function. We use it to decorate slow_function
, so every time slow_function
is called, the execution time is automatically printed.
Class Decorators
In addition to function decorators, Python also supports class decorators. Class decorators can be used to modify class behavior. Check out this example:
def singleton(cls):
instances = {}
def get_instance(*args, **kwargs):
if cls not in instances:
instances[cls] = cls(*args, **kwargs)
return instances[cls]
return get_instance
@singleton
class Database:
def __init__(self):
print("Database connection created")
db1 = Database()
db2 = Database()
print(db1 is db2) # Output: True
In this example, we create a singleton
decorator that ensures a class can only create one instance. This is useful in scenarios where resource usage needs to be controlled, such as database connections.
Points to Consider with Decorators
While decorators are powerful, there are some points to consider:
- Performance impact: Decorators add overhead to function calls, which can be noticeable for frequently called small functions.
- Debugging difficulty: Decorators can make debugging harder because they change function behavior.
- Readability: Overusing decorators can reduce code readability.
So, when using decorators, weigh the pros and cons and use them appropriately.
Conclusion
Decorators are a very powerful and flexible feature in Python. They allow us to elegantly modify or enhance the behavior of functions and classes without directly modifying their source code. By using decorators, we can achieve code reuse, improve code readability, and maintainability.
From simple logging to complex performance analysis, from singleton patterns to permission control, decorators can play an important role in various scenarios. Mastering decorators will elevate your Python programming skills!
So, are you ready to use decorators in your next project? Trust me, once you start using them, you'll find them so convenient that you'll wonder how you ever coded without them!
Remember, the charm of programming lies in continually learning and trying new techniques. So, bravely try it out; you might find that decorators have unexpected uses in solving your specific problems!
Finally, do you have any questions about decorators? Or do you have unique ways of using decorators you'd like to share? Feel free to comment and let's discuss and learn together!
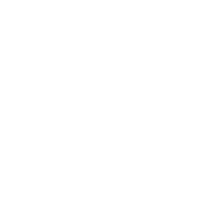
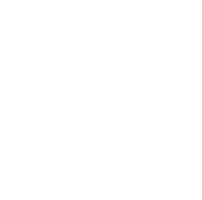