You are a professional linguist specializing in direct translations from other languages to American English.
- Automatically identify the source language of the given text.
- Provide a professional translation from the identified source language to American English.
- Preserve all markdown image links, including

and Obsidian-style links (![[xxx]]
). - Preserve blank lines between paragraphs.
- The translation should be complete and faithful to the original text.
- Output only the translation content, translating whatever the original text contains.
-
Do not provide any explanations or additional text beyond the translation.
-
Output the translation in American English.
- Do not include any comments, annotations, or explanations.
- Do not use any introductory phrases or conclusions.
INPUT:
Hello, dear Python enthusiasts! Today, let's talk about a super important tool in Python data analysis - the DataFrame. I'm sure many of you have heard of this term, but do you really understand its power? Let's dive deep into this data analysis powerhouse!
Getting to Know It
First of all, what is a DataFrame? Simply put, a DataFrame is a two-dimensional, labeled data structure, similar to an Excel spreadsheet. It is the core data structure in Python's Pandas library, providing us with powerful data processing and analysis capabilities.
You might ask, "Why use a DataFrame? Can't I just use lists or dictionaries?" Good question! Let me give you an example. Suppose you are a data analyst who needs to analyze a company's sales data. The data includes date, product name, sales volume, and sales amount. If you store this data in lists or dictionaries, you'll find that:
- The data organization is not intuitive.
- It's difficult to perform complex data operations, such as filtering and sorting.
- It's not convenient for statistical analysis.
Using a DataFrame, these problems are solved effortlessly!
Creating a DataFrame
So, how do you create a DataFrame? Pandas provides multiple methods, and the most common one is to create it from a dictionary. For example:
import pandas as pd
data = {
'date': ['2023-01-01', '2023-01-02', '2023-01-03'],
'product': ['A', 'B', 'A'],
'sales_volume': [100, 150, 120],
'sales_amount': [1000, 1800, 1200]
}
df = pd.DataFrame(data)
print(df)
Output:
date product sales_volume sales_amount
0 2023-01-01 A 100 1000
1 2023-01-02 B 150 1800
2 2023-01-03 A 120 1200
See how the data becomes clear and organized immediately?
Exploring Data
After creating a DataFrame, we can start exploring the data. DataFrame provides many convenient methods for viewing and analyzing data.
Basic Information
Want to quickly understand the basic information about the data? Try these:
print(df.info()) # Show basic information about the DataFrame
print(df.describe()) # Show statistical summaries for numeric columns
print(df.head()) # Show the first few rows of data
These methods can help you quickly understand the data structure, types, and basic statistical features. I particularly like the describe()
method, as it gives you a glimpse of the data distribution at a glance.
Accessing Data
DataFrame provides multiple ways to access data:
print(df['product']) # Access a single column
print(df[['date', 'product']]) # Access multiple columns
print(df.loc[0]) # Access a row by label
print(df.iloc[0]) # Access a row by position
See, it's much more convenient than manipulating lists or dictionaries, isn't it?
Data Filtering
The real power of DataFrame lies in its filtering capabilities:
print(df[df['sales_volume'] > 100]) # Filter records with sales volume greater than 100
print(df[(df['product'] == 'A') & (df['sales_amount'] > 1000)]) # Compound condition filtering
This flexible filtering makes data analysis so easy!
Data Operations
In addition to viewing and filtering data, DataFrame also provides rich data operation functionalities.
Adding Columns
Want to add a new column? Piece of cake:
df['profit'] = df['sales_amount'] - df['sales_volume'] * 8 # Assuming a cost of 8
print(df)
Sorting
Need to sort the data? No problem:
print(df.sort_values('sales_amount', ascending=False)) # Sort by sales amount in descending order
Grouping and Aggregation
Grouping and aggregation are common needs in data analysis:
print(df.groupby('product').agg({
'sales_volume': 'sum',
'sales_amount': 'mean'
}))
This operation will group the data by product, and calculate the total sales volume and mean sales amount for each product. Convenient, isn't it?
Conclusion
Well, dear readers, today we've briefly introduced the basic usage of DataFrame. Can you feel its power? DataFrame not only organizes data more intuitively but also provides rich data operation and analysis functionalities, greatly improving our work efficiency.
Of course, this is just the tip of the iceberg when it comes to DataFrame's capabilities. If you want to learn more, I recommend checking out the official Pandas documentation, which has more detailed introductions and examples.
Have you ever used DataFrame in your actual work? What interesting use cases have you encountered? Feel free to share your experiences in the comments! Let's discuss and grow together!
Remember, in the world of data analysis, DataFrame is your powerful assistant. Master it, and you'll find data analysis to be so simple and fun!
Next time, we'll explore how to perform more complex data analysis tasks with DataFrame. Stay tuned!
Hi, dear Python data analysis enthusiasts! Today, we'll dive deep into a crucial yet often overlooked aspect of the data analysis process - data cleaning and transformation. You might wonder, "Why is this topic so important?" Let me tell you, in the real world, the data we encounter is often messy, incomplete, or inconsistent in format. If we don't properly clean and transform this data, our subsequent analysis work will become challenging, and we might even draw incorrect conclusions. So, let's learn how to leverage Python's powerful tools to tackle these tricky problems!
Data Cleaning: Breathe New Life into Your Data
Handling Missing Values
When dealing with real-world data, missing values are almost inevitable. They might be caused by errors in the data collection process, system failures, or simply because some information is unavailable. Regardless of the reason, we need to handle these missing values properly to ensure the accuracy of our analysis results.
Pandas provides various methods for handling missing values. Let's look at a few common ones:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan, 4],
'B': [5, np.nan, np.nan, 8],
'C': [9, 10, 11, 12]
})
print(df.dropna())
print(df.fillna(0))
print(df.fillna(method='ffill'))
print(df.fillna(method='bfill'))
See how convenient it is? However, be aware that different handling methods may have different impacts on your analysis results. For example, dropping missing values might reduce the sample size, while filling missing values might introduce bias. Therefore, choose the appropriate method based on your specific situation.
Handling Duplicate Values
Duplicate values are another common data quality issue. Sometimes, due to errors in the data collection or processing, the same record might be entered multiple times. Not only does this waste storage space, but it can also introduce bias in the analysis results.
Fortunately, Pandas provides simple methods to detect and handle duplicate values:
df = pd.DataFrame({
'A': [1, 2, 2, 3, 3],
'B': ['a', 'b', 'b', 'c', 'c']
})
print(df.duplicated())
print(df.drop_duplicates())
print(df.drop_duplicates(keep='last'))
It's that simple, isn't it? But remember, before dropping duplicate values, make sure to carefully check if these duplicates are truly data errors or if there are other reasonable explanations.
Data Type Conversion
Sometimes, we need to change the data type to perform specific operations or analysis. For example, converting a string date to a datetime type, or converting an object-type numeric value to a float type. Pandas provides various methods for these conversions:
df = pd.DataFrame({
'A': ['1', '2', '3'],
'B': ['2023-01-01', '2023-01-02', '2023-01-03'],
'C': ['1.1', '2.2', '3.3']
})
df['A'] = df['A'].astype(int)
df['B'] = pd.to_datetime(df['B'])
df['C'] = df['C'].astype(float)
print(df.dtypes)
See? With simple operations, we've completed the data type conversions. This not only makes subsequent data operations more convenient but also improves data processing efficiency.
Data Transformation: Unleashing Your Data's Potential
Reshaping Data
Sometimes, we need to change the shape of the data to better suit our analysis needs. Pandas provides powerful melt()
and pivot()
functions to achieve this:
df_wide = pd.DataFrame({
'name': ['John', 'Jane'],
'math': [90, 95],
'english': [85, 100]
})
df_long = df_wide.melt(id_vars=['name'], var_name='subject', value_name='score')
print(df_long)
df_wide_again = df_long.pivot(index='name', columns='subject', values='score')
print(df_wide_again)
This operation is very useful in data analysis. For example, when you need to compare scores across different subjects, the long format might be more suitable; while when you need to view each student's overall performance, the wide format might be more intuitive.
Data Grouping and Aggregation
Data grouping and aggregation are common operations in data analysis. They can help us discover patterns and trends in the data. Pandas' groupby()
function provides powerful grouping and aggregation capabilities:
df = pd.DataFrame({
'category': ['A', 'B', 'A', 'B', 'A', 'B'],
'value1': [1, 2, 3, 4, 5, 6],
'value2': [10, 20, 30, 40, 50, 60]
})
grouped = df.groupby('category').mean()
print(grouped)
grouped = df.groupby('category').agg({
'value1': ['mean', 'max'],
'value2': ['min', 'sum']
})
print(grouped)
See how simple operations can yield interesting statistical results? This is very useful in actual data analysis work. For example, you can use this method to analyze sales performance across different product categories or customer behavior across different regions.
Applying Custom Functions
Sometimes, built-in functions might not meet our needs. In such cases, we can use the apply()
function to apply custom functions:
df = pd.DataFrame({
'name': ['John', 'Jane', 'Mike'],
'age': [25, 30, 35],
'city': ['New York', 'London', 'Paris']
})
def age_category(age):
if age < 30:
return 'Young'
elif age < 40:
return 'Middle'
else:
return 'Old'
df['age_category'] = df['age'].apply(age_category)
print(df)
Through this approach, we can transform and process data in any way we need, greatly increasing the flexibility of data processing.
Conclusion
Well, dear readers, today we've explored the important topic of data cleaning and transformation. We've learned how to handle missing values and duplicate values, how to perform data type conversions, and how to reshape data, perform grouping and aggregation, and apply custom functions. These skills are incredibly useful in actual data analysis work.
Remember, data cleaning and transformation is not a one-off process. It requires a deep understanding of your data, as well as patience and attention to detail. However, once you master these skills, you'll be able to extract valuable information from messy raw data, laying a solid foundation for subsequent analysis work.
Have you encountered any challenging data cleaning problems in your work? How did you solve them? Feel free to share your experiences and thoughts in the comments! Let's learn and grow together!
Next time, we'll explore how to perform data visualization in Python. Stay tuned!
Hi, dear Python data analysis enthusiasts! Today, we'll discuss an exciting topic - data visualization. Have you ever encountered a situation where you spent a lot of time cleaning and analyzing data, reached some great conclusions, but when you presented these results to others, they looked at you with blank stares? If so, data visualization is your savior!
Data visualization not only makes your analysis results more intuitive and understandable but also helps you discover hidden patterns and trends in the data. Today, we'll explore how to create various beautiful charts using Python's two powerful visualization tools - Matplotlib and Seaborn. Are you ready? Let's begin this visual feast!
Matplotlib: The Foundation of Plotting
Matplotlib is Python's most fundamental and widely used plotting library. It provides a MATLAB-like plotting API and can create various static, dynamic, and interactive plots. Although it might not be the most intuitive at times, its flexibility and customizability are unparalleled.
Line Plots: Showcasing Trends
Line plots are one of the most common chart types, particularly suitable for showing trends over time. Let's look at an example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='sin(x)')
plt.title('Sine Wave')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.grid(True)
plt.show()
See, we created a beautiful sine wave plot with just a few lines of code! You can experiment with adjusting various parameters, such as line color, thickness, title font size, and more, to create the desired effect.
Scatter Plots: Exploring Correlations
Scatter plots are a great tool for observing the relationship between two variables. Let's see how to create a scatter plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.random.randn(100)
y = 2*x + np.random.randn(100)
plt.figure(figsize=(10, 6))
plt.scatter(x, y, alpha=0.5)
plt.title('Scatter Plot')
plt.xlabel('x')
plt.ylabel('y')
plt.grid(True)
plt.show()
This plot clearly shows the positive correlation between x and y. You can emphasize different information by adjusting the point size, color, transparency, and more.
Bar Charts: Comparing Quantities
Bar charts are suitable for comparing the differences in quantities between different categories. Here's a simple example:
import matplotlib.pyplot as plt
categories = ['A', 'B', 'C', 'D']
values = [4, 7, 2, 5]
plt.figure(figsize=(10, 6))
plt.bar(categories, values)
plt.title('Bar Chart')
plt.xlabel('Category')
plt.ylabel('Value')
plt.show()
Isn't it intuitive? You can easily see the differences between categories. If you want more complex effects, such as stacked bar charts or side-by-side bar charts, Matplotlib can easily handle them too.
Seaborn: The Statistical Visualization Powerhouse
While Matplotlib is powerful, it might sometimes be a bit cumbersome to use. That's where Seaborn comes in handy. Seaborn is a statistical plotting library based on Matplotlib, providing a higher-level interface that makes it easy to create various statistical charts.
Histograms and Kernel Density Plots: Understanding Distributions
Histograms and kernel density plots are great tools for observing data distributions. Seaborn's distplot
function can simultaneously plot both:
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(1000)
plt.figure(figsize=(10, 6))
sns.distplot(data)
plt.title('Distribution Plot')
plt.xlabel('Value')
plt.ylabel('Density')
plt.show()
This plot not only shows the data distribution but also displays the probability density with a smooth curve. Isn't it more informative than a simple histogram?
Box Plots: Understanding Data Structure
Box plots are another powerful statistical chart that can simultaneously display the median, quartiles, and outliers of the data. Let's see how to create a box plot using Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
data = pd.DataFrame({
'group': ['A']*100 + ['B']*100 + ['C']*100,
'value': np.concatenate([np.random.normal(0, 1, 100),
np.random.normal(2, 1, 100),
np.random.normal(-1, 1, 100)])
})
plt.figure(figsize=(10, 6))
sns.boxplot(x='group', y='value', data=data)
plt.title('Box Plot')
plt.show()
See, this plot immediately shows the distribution of data for three groups, including the median, quartile ranges, and outliers. This is particularly useful when comparing distribution differences between groups.
Heatmaps: Visualizing Correlation Matrices
Heatmaps are a great tool for displaying variable correlations. Let's see how to create a heatmap using Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
np.random.seed(0)
data = np.random.randn(10, 10)
corr = np.corrcoef(data)
plt.figure(figsize=(10, 8))
sns.heatmap(corr, annot=True, cmap='coolwarm')
plt.title('Correlation Heatmap')
plt.show()
This heatmap clearly shows the correlations between variables, with deeper colors indicating stronger correlations. This is very useful when performing feature selection or exploring variable relationships.
Conclusion
Well, dear readers, today we've explored how to perform data visualization using Matplotlib and Seaborn. We've learned how to create line plots, scatter plots, bar charts, histograms, box plots, and heatmaps. These are all common chart types in data analysis, and mastering them will greatly enhance your data analysis skills.
Remember, data visualization is not just about making your reports look pretty. More importantly, it helps you better understand your data, discover hidden patterns and trends, and effectively communicate your findings to others.
Have you used these charts in your actual work? Which type of chart is your favorite? Have you encountered any interesting visualization challenges? Feel free to share your experiences and thoughts in the comments! Let's learn and grow together!
Next time, we'll explore how to apply machine learning to data analysis. Stay tuned!
Hi, dear Python data analysis enthusiasts! Today, we'll discuss an exciting topic - machine learning. Have you ever heard the term "machine learning" but found it daunting and didn't know where to start? Don't worry, today we'll unveil the mysteries of machine learning and explore how to apply it to our data analysis.
Machine learning can be considered the "ultimate weapon" in data analysis. It can automatically learn patterns and rules from massive amounts of data, and then use this learned knowledge to make predictions or decisions. Sounds magical, doesn't it? But with Python and the powerful scikit-learn machine learning library, implementing these capabilities is not that difficult. Let's explore together!
Scikit-learn: Your Machine Learning Swiss Army Knife
Scikit-learn is one of the most popular machine learning libraries in Python. It provides a wide range of machine learning algorithms, from simple linear regression to complex neural networks. Moreover, its API is designed consistently, making it very convenient to use.
Data Preparation: The First Step in Machine Learning
Before starting with machine learning, we need to prepare our data. This usually involves data cleaning, feature engineering, and data splitting. Let's look at a simple example:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
iris = load_iris()
X, y = iris.data, iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
In this example, we use the built-in iris dataset from scikit-learn. We split the data into training and test sets, which is a common practice in machine learning. The training set is used to train the model, and the test set is used to evaluate the model's performance.
Classification: Predicting Categories
Classification is one of the most common tasks in machine learning. Its goal is to predict which category a sample belongs to. Let's use a decision tree classifier to solve the iris classification problem:
from sklearn.tree import DecisionTreeClassifier
from sklearn.metrics import accuracy_score
clf = DecisionTreeClassifier(random_state=42)
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
See, we implemented a classification model with just a few lines of code! The decision tree is an intuitive model that classifies samples through a series of if-else decisions.
Regression: Predicting Numerical Values
Regression aims to predict a continuous numerical value. For example, we might want to predict someone's income based on their age, education, work experience, and other features. Let's use linear regression to solve a simple regression problem:
from sklearn.datasets import make_regression
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
import numpy as np
X, y = make_regression(n_samples=100, n_features=1, noise=10, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
reg = LinearRegression()
reg.fit(X_train, y_train)
y_pred = reg.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse:.2f}")
Linear regression is one of the simplest regression models, assuming a linear relationship between the features and the target variable. Although simple, it can perform well in many situations.
Clustering: Discovering Groups in Data
Clustering is an unsupervised learning method that aims to group similar samples together. K-means is one of the most commonly used clustering algorithms. Let's see how to use it:
from sklearn.cluster import KMeans
from sklearn.datasets import make_blobs
X, _ = make_blobs(n_samples=300, centers=4, cluster_std=0.60, random_state=0)
kmeans = KMeans(n_clusters=4, random_state=0)
kmeans.fit(X)
labels = kmeans.labels_
The K-means algorithm iteratively adjusts the cluster centers until it partitions the data into K clusters. It has applications in various scenarios, such as customer segmentation, image segmentation, and more.
Model Evaluation: How Good Is Your Model?
Creating a model is just the first step; we also need to evaluate its performance. Different types of problems have different evaluation metrics. For example, for classification problems, we might care about accuracy, precision, recall, and more; for regression problems, we might care about mean squared error, R-squared, and so on.
Scikit-learn provides a rich set of evaluation metrics:
from sklearn.metrics import classification_report, mean_absolute_error, r2_score
print(classification_report(y_test, y_pred))
mae = mean_absolute_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean Absolute Error: {mae:.2f}, R-squared: {r2:.2f}")
Proper evaluation is crucial for understanding the strengths and weaknesses of your model and making informed decisions.
Conclusion
Well, dear readers, today we've unveiled the mysteries of machine learning and explored how to apply it to data analysis using Python and scikit-learn. We've learned about data preparation, classification, regression, clustering, and model evaluation.
Remember, machine learning is not magic; it's a powerful tool that can help us uncover patterns and insights from data. With the right techniques and tools, it can take your data analysis skills to the next level.
Have you applied machine learning to your data analysis work? What challenges have you faced? Feel free to share your experiences and thoughts in the comments! Let's learn and grow together!
Next time, we'll explore more advanced machine learning techniques and their applications in data analysis. Stay tuned!
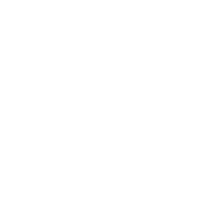
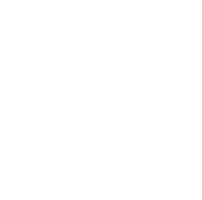