Origin
Have you ever faced a pile of data and didn't know where to start? Or felt inefficient using clumsy Excel when processing data? As a data analyst, I deeply understand the importance of mastering Python for data analysis work. Today, let me guide you into the world of Python data analysis.
Fundamentals
Before we start hands-on work, we need to understand the essence of data analysis. Data analysis isn't simply running data through Python programs; it's a process requiring rigorous thinking and creativity. I often tell my students that data analysis is like solving a case - data are the clues, and we're searching for the truth behind these clues.
First, let's meet our "investigation tools" - the core libraries for Python data analysis. Just as detectives need magnifying glasses and microscopes, we need specific tools to help us analyze data:
- NumPy: This is our basic toolbox, providing efficient array computation capabilities
- Pandas: This is our main weapon for data processing and analysis
- Matplotlib/Seaborn: These are our plotting tools for data visualization
Let me use a real example to show how these tools work together. Suppose we need to analyze sales data from a chain store:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
sales_data = pd.read_csv('sales.csv')
sales_data['sales_amount'] = sales_data['sales_amount'].fillna(0)
summary = sales_data['sales_amount'].describe()
plt.figure(figsize=(10, 6))
sns.histplot(data=sales_data, x='sales_amount')
plt.title('Sales Amount Distribution')
plt.show()
Advanced Level
After mastering the basic tools, we need to understand the data analysis process more deeply. I remember when I first started doing data analysis, I was always eager for quick results. But experience has taught me that a complete data analysis process must include these steps:
-
Data Collection: This is the most fundamental step. Like detectives gathering evidence, we need to ensure data integrity and reliability.
-
Data Cleaning: This might be the most time-consuming step, but it's the most crucial. Common issues I encounter include: missing values, outliers, duplicate data. Let me share some practical data cleaning code:
def clean_data(df):
# Remove duplicate rows
df = df.drop_duplicates()
# Handle missing values
numeric_columns = df.select_dtypes(include=[np.number]).columns
df[numeric_columns] = df[numeric_columns].fillna(df[numeric_columns].mean())
# Handle outliers
for col in numeric_columns:
Q1 = df[col].quantile(0.25)
Q3 = df[col].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
df[col] = df[col].clip(lower_bound, upper_bound)
return df
Practical Application
Now that we've covered the theory, let's look at a complete practical case. Suppose we need to analyze user purchasing behavior on an e-commerce platform:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.cluster import KMeans
from sklearn.preprocessing import StandardScaler
df = pd.read_csv('ecommerce_data.csv')
df['purchase_frequency'] = df['total_purchases'] / df['days_since_first_purchase']
df['average_order_value'] = df['total_spent'] / df['total_purchases']
df['customer_value'] = df['purchase_frequency'] * df['average_order_value']
scaler = StandardScaler()
features = ['purchase_frequency', 'average_order_value', 'customer_value']
X = scaler.fit_transform(df[features])
kmeans = KMeans(n_clusters=4, random_state=42)
df['cluster'] = kmeans.fit_predict(X)
plt.figure(figsize=(12, 8))
sns.scatterplot(data=df, x='purchase_frequency', y='average_order_value',
hue='cluster', palette='deep')
plt.title('Customer Clustering Results')
plt.show()
Insights
Throughout my data analysis career, I've gradually grasped several important principles:
-
Data Quality is Supreme: Garbage in, garbage out. Ensuring data quality is the first step of analysis.
-
Business Understanding is Essential: Pure technical analysis isn't enough; we need deep understanding of business context. Once, I spent a long time analyzing abnormal data, only to find it was due to a company promotion.
-
The Power of Visualization: A picture is worth a thousand words. Good visualization can make complex data intuitive and easy to understand.
-
Continuous Learning: The field of data analysis develops rapidly, and we need to constantly learn new tools and methods.
Looking Forward
The future of Python data analysis is full of opportunities and challenges. With the advent of the big data era, we need to handle increasingly large volumes of data and more complex data types. This requires us to continuously upgrade our skills.
I suggest you continue deeper learning in these areas:
- Deep dive into machine learning algorithms
- Master big data processing tools
- Learn automated data analysis processes
- Study deep learning applications in data analysis
Remember, data analysis isn't just technology; it's an art. It requires us to use creative thinking to discover hidden value in data. What do you think? Feel free to share your thoughts and experiences in the comments.
(End of article)
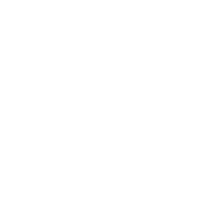
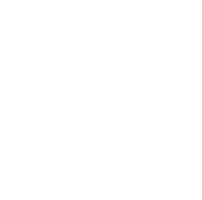