Introduction
Have you encountered these frustrations? Memory is always insufficient when processing large-scale data; matrix operations are frustratingly slow. Actually, this might be because you haven't mastered the correct usage of sparse matrices. Today, I'll share with you my insights from working with sparse matrices.
Understanding
When it comes to sparse matrices, you might think it's a very academic topic. But it's actually all around us. Think about it - in your social network, haven't you only established connections with a small portion of people? On shopping websites, haven't you only rated a tiny fraction of products? These are typical applications of sparse matrices.
Let's understand what a sparse matrix is with a simple example. Suppose we have a social networking platform with 1000 users. If we use a traditional dense matrix to store user follow relationships, we need a 1000x1000 matrix, which means 1 million storage units. However, in reality, each user might only follow 20-30 other users, meaning over 99% of the matrix elements are 0. In such cases, using sparse matrix storage becomes particularly important.
Practice
Let's look at how to use sparse matrices in Python. First, we need to import the necessary libraries:
import numpy as np
from scipy import sparse
import time
def create_sparse_matrix(size, density):
# Randomly generate sparse matrix
matrix = np.random.choice([0, 1], size=(size, size), p=[1-density, density])
return sparse.csr_matrix(matrix)
size = 10000
density = 0.001
sparse_matrix = create_sparse_matrix(size, density)
dense_matrix = sparse_matrix.toarray()
print(f"Sparse matrix memory usage: {sparse_matrix.data.nbytes / 1024:.2f} KB")
print(f"Dense matrix memory usage: {dense_matrix.nbytes / 1024:.2f} KB")
Why did I choose the CSR format? This is because CSR format provides a good performance balance in most cases. It's particularly suitable for matrix-vector multiplication operations, which are core operations in many machine learning algorithms.
Optimization
In practical work, I've found that many developers have misconceptions when using sparse matrices. For example, some believe that using sparse matrix format will always improve performance. This thinking is incorrect. Let's look at a specific example:
def compare_performance(size, density):
# Create test matrices
sparse_matrix = create_sparse_matrix(size, density)
dense_matrix = sparse_matrix.toarray()
# Test matrix multiplication performance
start_time = time.time()
sparse_result = sparse_matrix @ sparse_matrix
sparse_time = time.time() - start_time
start_time = time.time()
dense_result = dense_matrix @ dense_matrix
dense_time = time.time() - start_time
return sparse_time, dense_time
densities = [0.001, 0.01, 0.1, 0.5]
size = 1000
for density in densities:
sparse_time, dense_time = compare_performance(size, density)
print(f"Density: {density}")
print(f"Sparse matrix computation time: {sparse_time:.4f} seconds")
print(f"Dense matrix computation time: {dense_time:.4f} seconds")
print("---")
Through this test, you'll discover an interesting phenomenon: when matrix density is high (e.g., over 10%), using sparse matrices actually reduces performance. This is because sparse matrix format requires additional index information, and when there are many non-zero elements, this overhead exceeds the space saved.
Advanced
Speaking of this, I want to share a problem I encountered in a real project. We needed to process a large-scale user-item rating matrix with about 1 million users and 500,000 items. Using traditional dense matrix storage would require:
1,000,000 × 500,000 × 8 bytes ≈ 3.7TB
This is clearly impractical. By analyzing the data characteristics, we found that the matrix density was only about 0.01%. After using sparse matrices, memory usage dropped to a few GB level, which is a huge improvement.
Here are some key techniques for handling large-scale sparse matrices:
def process_large_sparse_matrix():
# Use generator for batch processing
def data_generator(total_rows, batch_size):
for start in range(0, total_rows, batch_size):
end = min(start + batch_size, total_rows)
# Simulate data generation here
yield create_sparse_matrix(end - start, 0.001)
# Process large matrix in batches
total_rows = 1000000
batch_size = 1000
result = sparse.csr_matrix((batch_size, batch_size))
for batch in data_generator(total_rows, batch_size):
# Perform matrix operations
result = result + batch.T @ batch
return result
Reflection
In the process of learning and using sparse matrices, I've summarized several important experiences:
-
Data analysis is crucial. Before using sparse matrices, you must first analyze data sparsity. I usually calculate matrix density, and only consider using sparse format if density is below 5%.
-
Format selection should be careful. Different sparse matrix formats (CSR, CSC, COO, etc.) are suitable for different scenarios. For example, if frequent matrix element modifications are needed, COO format might be a better choice.
-
Performance testing is essential. In practical applications, you must perform performance tests on different solutions. Sometimes, what seems like a better solution might not suit your specific scenario.
Future Outlook
After all this discussion, you might ask: what are the future development trends for sparse matrices? I think there are two main directions:
First is hardware optimization. With the development of GPUs and specialized hardware, there's still great potential for improving sparse matrix operation performance. For example, NVIDIA's latest GPUs have begun to specifically optimize for sparse matrix operations.
Second is algorithmic innovation. Researchers are already exploring sparse matrix processing methods combined with machine learning, which might bring about qualitative leaps in performance.
What do you think? Feel free to share your thoughts and experiences in the comments. If you've encountered any problems while using sparse matrices, you can also leave a message for discussion. Let's grow together through practice.
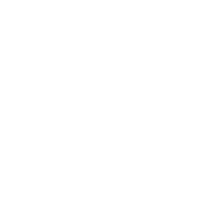