Hey, dear Python enthusiasts! Today we're embarking on an exciting journey into data analysis. Have you ever faced a large pile of raw data, not knowing where to start? Or maybe you've already mastered some basics but feel like you're missing that extra edge? Don't worry, this article will take you deep into the mysteries of Python data analysis, from basics to advanced, step by step unveiling the mystique of data analysis. Are you ready? Let's begin!
First Encounter
Remember when you first encountered Python? Didn't you find it simple to learn yet powerful? In the field of data analysis, Python really shines. And when it comes to Python data analysis, we can't help but mention our protagonist - the Pandas library.
Pandas is like the Swiss Army knife of the data analysis world, compact yet fully-featured. It provides two core data structures: Series and DataFrame. A Series is like an enhanced list, while a DataFrame can be seen as a two-dimensional table composed of multiple Series.
Come, let's first look at how to create a simple Series:
import pandas as pd
s = pd.Series([1, 3, 5, np.nan, 6, 8])
print(s)
Output result:
0 1.0
1 3.0
2 5.0
3 NaN
4 6.0
5 8.0
dtype: float64
See that? Isn't it intuitive? Series can store not only numerical values, but also strings, boolean values, and even Python objects. It's like a labeled one-dimensional array that can be accessed both by index and by label.
What about DataFrame? It's more like an Excel spreadsheet or SQL table. Let's create a simple DataFrame:
df = pd.DataFrame({
'A': [1, 2, 3, 4],
'B': pd.date_range(start='20230101', periods=4),
'C': pd.Series(1, index=list(range(4)), dtype='float32'),
'D': np.array([3] * 4, dtype='int32'),
'E': pd.Categorical(["test", "train", "test", "train"]),
'F': 'foo'
})
print(df)
Output result:
A B C D E F
0 1 2023-01-01 1.0 3 test foo
1 2 2023-01-02 1.0 3 train foo
2 3 2023-01-03 1.0 3 test foo
3 4 2023-01-04 1.0 3 train foo
Look, this is a DataFrame! It can contain different types of data, with each column potentially being a different data type. Doesn't it look like a table? That's right, DataFrame is just that intuitive and powerful.
Cleaning
The first step in data analysis is often data cleaning. You might ask, why do we need to clean data? Imagine if your data was full of missing values, duplicates, or inconsistently formatted data, do you think you could get accurate analysis results?
Pandas provides a series of powerful tools to help us clean data. For example, handling missing values:
df.dropna(how='any') # Delete rows containing any NaN
df.fillna(value=5) # Fill NaN with 5
Or removing duplicates:
df.drop_duplicates()
Sometimes, we also need to convert data types:
df['E'] = df['E'].astype('category')
Data cleaning might seem tedious, but trust me, it's one of the most important steps in data analysis. A clean, consistent dataset will make subsequent analysis much easier.
Visualization
After cleaning the data, we can start exploring it. The most intuitive way to explore data is through data visualization. Python provides two powerful visualization libraries: Matplotlib and Seaborn.
Matplotlib is the pioneer of Python data visualization, providing very flexible plotting capabilities. Come, let's draw a simple line chart:
import matplotlib.pyplot as plt
plt.figure(figsize=(10,6))
plt.plot(df['A'], df['C'], label='A vs C')
plt.title('A simple line plot')
plt.xlabel('A')
plt.ylabel('C')
plt.legend()
plt.show()
This code will generate a simple line chart showing the relationship between column A and column C.
Seaborn, on the other hand, is a more advanced statistical visualization library based on Matplotlib. It provides more aesthetically pleasing default styles and a more concise API. For example, we can easily draw a scatter plot with Seaborn:
import seaborn as sns
sns.scatterplot(x='A', y='C', hue='E', data=df)
plt.title('A scatter plot with Seaborn')
plt.show()
This graph not only shows the relationship between A and C but also distinguishes different categories in column E with colors. Don't you feel the data has suddenly come to life?
Machine Learning
When talking about data analysis, how can we not mention machine learning? Python's Scikit-learn library provides a rich set of machine learning algorithms and tools.
For example, we can easily implement a simple linear regression with Scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
X = df[['A']]
y = df['C']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f'Mean squared error: {mse}')
print(f'R-squared score: {r2}')
This code implements a simple linear regression model. We first split the data into training and test sets, then train the model, and finally evaluate the model's performance.
Of course, machine learning is far more than just linear regression. Scikit-learn also provides various classification algorithms, clustering algorithms, dimensionality reduction algorithms, and more. For example, we can easily implement a K-means clustering:
from sklearn.cluster import KMeans
X = df[['A', 'C']]
kmeans = KMeans(n_clusters=3, random_state=42)
kmeans.fit(X)
df['Cluster'] = kmeans.labels_
plt.figure(figsize=(10,6))
sns.scatterplot(x='A', y='C', hue='Cluster', data=df)
plt.title('K-means clustering result')
plt.show()
This code implements a K-means clustering and visualizes the clustering results. Don't you think machine learning isn't so scary after all?
Advanced
Alright, now you've mastered the basics of data analysis. But if you want to go a step further, there are many advanced techniques waiting for you to explore.
For example, Pandas' groupby() function allows you to easily group and aggregate data:
df.groupby('E')['A'].mean()
Or, you can use Scikit-learn's feature selection tools to select the most important features:
from sklearn.feature_selection import SelectKBest
from sklearn.feature_selection import f_regression
X = df[['A', 'C', 'D']]
y = df['E']
selector = SelectKBest(score_func=f_regression, k=2)
X_new = selector.fit_transform(X, y)
selected_features = X.columns[selector.get_support()]
print("Selected features:", selected_features)
This is just the tip of the iceberg. The world of data analysis and machine learning is vast, with many interesting techniques waiting for you to explore. For instance, have you heard of Principal Component Analysis (PCA)? It can help us reduce the dimensionality of data while retaining most of the information. Or, do you know about cross-validation? It can help us more accurately evaluate the performance of models.
Summary
Our journey into data analysis ends here. We started from the basic data structures of Pandas, went through data cleaning, visualization, all the way to machine learning and some advanced techniques. This is just a glimpse of the world of data analysis, there's still much more exciting content waiting for you to explore.
Remember, data analysis is not just about mastering some tools and techniques, more importantly, it's about cultivating data thinking. When you face a dataset, what questions will you ask? How will you verify your hypotheses? How do you extract valuable information from the data? These are the core of data analysis.
Finally, I want to say that data analysis is a process of continuous learning and practice. Don't be afraid of making mistakes, every failure is an opportunity to learn. Maintain curiosity, be brave to try new methods and tools. Remember, in the world of data analysis, you will never feel bored!
So, are you ready to start your journey into data analysis? Let's explore together in this ocean of data!
Which part are you most interested in? Is it the magic of data cleaning, the art of data visualization, or the wisdom of machine learning? Feel free to share your thoughts in the comments. If you have any questions, you're also welcome to leave a message for discussion. Let's learn together and grow together!
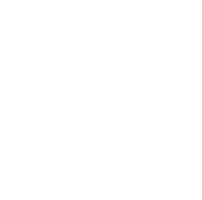