Hello, dear Python programming enthusiasts! Today, let's talk about the exciting and popular topic of Python data analysis. As a Python blogger, I have always felt that data analysis is one of the most powerful and practical applications of Python. Whether you want to analyze your company's sales data or study stock market trends, mastering Python data analysis skills can give you a great advantage. So, today we'll start from scratch and learn step by step about the core tools of Python data analysis: NumPy, Pandas, and Matplotlib. Ready? Let's begin this wonderful learning journey!
Why Choose Python?
Before we start, you might ask: "Why choose Python for data analysis?" That's a great question! Having once juggled multiple programming languages, I can confidently say that Python is definitely one of the best choices for data analysis. Why?
First, Python's syntax is simple and clear, making it ideal for rapid development and prototyping. I remember being amazed the first time I wrote a data analysis script in Python, realizing that just a few lines of code could accomplish complex data processing tasks, efficiency that is hard to match with other languages.
Second, Python has a wealth of data analysis libraries and tools. Libraries like NumPy, Pandas, and Matplotlib provide powerful support for data analysis. These libraries are not only powerful but also relatively easy to use, greatly lowering the learning curve.
Finally, Python has widespread applications in data science and machine learning. If you want to pursue these fields in the future, Python is definitely a good starting point.
So, let's begin our Python data analysis journey!
Basics of Data Analysis
Before diving into specific tools, let's first understand the basic steps of data analysis. Data analysis usually includes the following stages:
- Asking questions: Determining the questions we want to answer through data.
- Collecting data: Obtaining the necessary datasets.
- Cleaning data: Handling missing values, outliers, etc.
- Exploring data: Performing preliminary analysis to understand the basic features of the data.
- Modeling data: Using statistical or machine learning methods to analyze the data.
- Interpreting results: Explaining and visualizing the analysis results.
Remembering these steps is important because they remain constant regardless of the tools you use. In the following content, we'll see how to complete these steps using various Python libraries.
NumPy: The Foundation of Numerical Computing
First, let's get to know NumPy. NumPy is the foundational library for Python data analysis, providing high-performance multidimensional array objects and various mathematical functions. I was simply amazed by its powerful features the first time I used NumPy.
NumPy Arrays: The Tool for Multidimensional Data
The core of NumPy is the ndarray object, an N-dimensional array. Compared to Python's lists, NumPy arrays are much more efficient when handling large amounts of data. Let's look at a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
arr_2d = np.array([[1, 2, 3], [4, 5, 6]])
print(arr_2d)
Output:
[1 2 3 4 5]
[[1 2 3]
[4 5 6]]
See? We can easily create one-dimensional and two-dimensional arrays. This is very useful for handling tabular data or matrix operations.
NumPy Magic: Broadcasting
One powerful feature of NumPy is broadcasting. It allows us to perform operations on arrays of different shapes. This might sound a bit abstract, so let me explain with an example:
arr = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
col_add = arr + np.array([100, 200, 300, 400])
print(col_add)
Output:
[[101 202 303 404]
[105 206 307 408]
[109 210 311 412]]
See? We performed addition on each column of the two-dimensional array using a one-dimensional array. That's the magic of broadcasting! It allows for very flexible array operations.
NumPy Mathematical Functions: The Power of Vectorized Operations
NumPy also provides a large number of mathematical functions that can be directly applied to entire arrays, known as vectorized operations. For example:
arr = np.array([1, 2, 3, 4, 5])
sqrt_arr = np.sqrt(arr)
print(sqrt_arr)
exp_arr = np.exp(arr)
print(exp_arr)
Output:
[1. 1.41421356 1.73205081 2. 2.23606798]
[ 2.71828183 7.3890561 20.08553692 54.59815003 148.4131591 ]
These operations are very common in data analysis. Imagine if you needed to perform such operations on an array containing millions of data points; NumPy's vectorized operations can greatly improve efficiency.
Pandas: The Swiss Army Knife of Data Processing
Next, let's look at Pandas. If NumPy is the foundation of data analysis, then Pandas is the high-level tool built on this foundation. Pandas provides two main data structures: Series and DataFrame, which make data processing extremely simple.
Series: A One-Dimensional Array with Labels
A Series can be thought of as a one-dimensional array with labels. Let's create a Series:
import pandas as pd
s = pd.Series([1, 3, 5, np.nan, 6, 8])
print(s)
Output:
0 1.0
1 3.0
2 5.0
3 NaN
4 6.0
5 8.0
dtype: float64
See? A Series not only stores data but also provides an index for each data point. This is particularly useful when handling time series data.
DataFrame: The Workhorse of Data Analysis
The DataFrame is the most commonly used data structure in Pandas, and it can be thought of as a table. Let's create a DataFrame:
df = pd.DataFrame({
'A': [1, 2, 3, 4],
'B': pd.date_range(start='20230101', periods=4),
'C': pd.Series(1, index=list(range(4)), dtype='float32'),
'D': np.array([3] * 4, dtype='int32'),
'E': pd.Categorical(["test", "train", "test", "train"]),
'F': 'foo'
})
print(df)
Output:
A B C D E F
0 1 2023-01-01 1.0 3 test foo
1 2 2023-01-02 1.0 3 train foo
2 3 2023-01-03 1.0 3 test foo
3 4 2023-01-04 1.0 3 train foo
This DataFrame contains multiple data types: integers, floats, dates, categorical data, and strings. This flexibility makes the DataFrame an ideal tool for handling complex datasets.
Data Cleaning: Handling Real-World Messy Data
In actual data analysis, we often encounter issues like missing values and duplicate data. Pandas provides various methods to handle these issues. For example:
df.fillna(value=5, inplace=True)
df.drop_duplicates(inplace=True)
df.rename(columns={'A': 'Alpha', 'B': 'Beta'}, inplace=True)
These operations make data cleaning straightforward. I remember being amazed at how intuitive and easy to use these features were when I first used them.
Data Grouping and Aggregation: Delving into Data
Pandas' groupby function allows us to group and aggregate data, which is very useful in data analysis. Let's look at an example:
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'three', 'two', 'two', 'one', 'three'],
'C': np.random.randn(8),
'D': np.random.randn(8)
})
grouped = df.groupby('A')['C'].mean()
print(grouped)
Output might be similar to:
A
bar -0.020141
foo 0.130459
Name: C, dtype: float64
This example shows how to group data by the values of one column and then calculate the mean of another column. This kind of operation is very useful when analyzing patterns and trends in data.
Matplotlib: The Art of Data Visualization
Finally, let's look at Matplotlib. Data visualization is a very important part of data analysis because it helps us intuitively understand the data. Matplotlib is one of the most popular plotting libraries in Python, offering rich plotting functionalities.
Basic Plotting: Starting Simple
Let's start with a simple line plot:
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * np.pi, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y)
plt.title('Sine Wave')
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.grid(True)
plt.show()
This code generates a plot of a sine wave. Isn't it simple? With just a few lines of code, we created a professional-looking chart.
Multiple Subplots: Displaying Multiple Charts at Once
In actual analysis, we often need to display multiple subplots in one figure. Matplotlib makes this very easy:
fig, axs = plt.subplots(2, 2, figsize=(12, 10))
axs[0, 0].plot(x, np.sin(x))
axs[0, 0].set_title('Sine')
axs[0, 1].plot(x, np.cos(x))
axs[0, 1].set_title('Cosine')
axs[1, 0].plot(x, np.tan(x))
axs[1, 0].set_title('Tangent')
axs[1, 1].plot(x, np.exp(x))
axs[1, 1].set_title('Exponential')
plt.tight_layout()
plt.show()
This code creates a 2x2 grid of plots, each showing different mathematical functions. This layout is very useful when comparing different datasets or showing different aspects of the data.
Custom Styles: Making Your Charts Stand Out
Matplotlib provides rich customization options, allowing you to create unique chart styles:
plt.style.use('seaborn')
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y1, label='sin(x)', color='#ff9999', linewidth=2)
ax.plot(x, y2, label='cos(x)', color='#66b3ff', linewidth=2)
ax.set_title('Sine and Cosine Waves', fontsize=20)
ax.set_xlabel('x', fontsize=14)
ax.set_ylabel('y', fontsize=14)
ax.legend(fontsize=12)
ax.grid(True, linestyle='--', alpha=0.7)
ax.set_facecolor('#f0f0f0')
plt.show()
This example shows how to use elements like color, line width, and font size to create a visually appealing chart. Remember, good data visualization should not only accurately represent the data but also be aesthetically pleasing and easy to read.
Practical Case: Stock Data Analysis
Now, let's apply what we've learned to a practical case. Suppose we want to analyze the past year's stock data of a company. We'll use Yahoo Finance data, read it with Pandas, perform some calculations with NumPy, and finally plot the charts with Matplotlib.
import yfinance as yf
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
stock_data = yf.download('AAPL', start='2022-01-01', end='2023-01-01')
stock_data['Daily Return'] = stock_data['Close'].pct_change()
stock_data['Cumulative Return'] = (1 + stock_data['Daily Return']).cumprod()
stock_data['MA50'] = stock_data['Close'].rolling(window=50).mean()
stock_data['MA200'] = stock_data['Close'].rolling(window=200).mean()
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(12, 10), sharex=True)
ax1.plot(stock_data.index, stock_data['Close'], label='Close Price')
ax1.plot(stock_data.index, stock_data['MA50'], label='50-day MA')
ax1.plot(stock_data.index, stock_data['MA200'], label='200-day MA')
ax1.set_title('AAPL Stock Price 2022')
ax1.set_ylabel('Price')
ax1.legend()
ax2.plot(stock_data.index, stock_data['Cumulative Return'])
ax2.set_title('AAPL Cumulative Return 2022')
ax2.set_xlabel('Date')
ax2.set_ylabel('Cumulative Return')
plt.tight_layout()
plt.show()
print(f"Average Daily Return: {stock_data['Daily Return'].mean():.4f}")
print(f"Return Standard Deviation: {stock_data['Daily Return'].std():.4f}")
print(f"Annualized Return: {(stock_data['Cumulative Return'].iloc[-1]**(252/len(stock_data))-1):.4f}")
This example demonstrates how to: 1. Use Pandas to read and process stock data 2. Perform some financial calculations with NumPy 3. Create complex multi-subplot visualizations with Matplotlib
Through this case, we can see the power of Python data analysis tools. In just a few dozen lines of code, we completed the entire process of data acquisition, processing, analysis, and visualization.
Conclusion and Outlook
We have traversed the basics of Python data analysis, from NumPy's array operations to Pandas' data processing, and finally to Matplotlib's data visualization. These tools provide us with powerful data analysis capabilities, allowing us to extract valuable information from raw data.
However, this is just the tip of the iceberg in the world of data analysis. As you become more familiar with these tools, you'll find more advanced features waiting to be explored. For example:
- Advanced data processing techniques in Pandas, such as pivot tables and time series analysis
- Advanced mathematical operations in NumPy, such as linear algebra and Fourier transforms
- Interactive plotting and animation features in Matplotlib
In addition, there are many other Python data analysis libraries worth learning, such as Seaborn (a statistical data visualization library based on Matplotlib), Plotly (an interactive plotting library), Scikit-learn (a machine learning library), and more.
Remember, data analysis is a process of continuous learning and practice. Each new dataset you analyze may teach you new techniques or insights. Stay curious and be willing to try, and you'll find data analysis to be a field full of fun and challenges.
Finally, I want to share my personal experience: the most important thing in learning these tools is to practice a lot. Find some datasets that interest you and try to analyze them using different methods. You might encounter various problems, but solving these problems is the best learning opportunity.
I hope this article inspires your interest in Python data analysis and helps you embark on your data analysis journey. If you have any questions or ideas, feel free to leave a comment for discussion. Let's explore the ocean of data together!
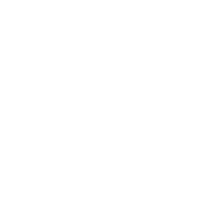
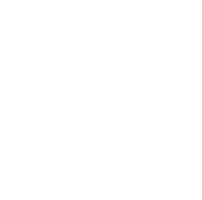