Hey, Python enthusiasts! Today let's talk about a super interesting topic - Python data analysis. Are you often overwhelmed by large amounts of data? Don't worry, let's explore how to tame these data beasts using Python, this powerful tool!
Why Choose Python?
You might ask, why choose Python for data analysis? Good question! Let me tell you, Python is like the Swiss Army knife of data analysis. Not only is its syntax simple to learn, but it also has a bunch of powerful libraries specifically designed for data analysis. Imagine having a transformable magic wand in your hand that can complete various complex data processing tasks with just a wave - isn't that cool?
I remember when I first started learning data analysis, I was quite intimidated by all the technical terms and complex code. But when I started using Python, especially libraries like Pandas, I was amazed! Data analysis could be so intuitive and fun. For example, with just a few lines of code, you can extract valuable information from a huge mess of data, like performing magic!
Essential Toolkit
Speaking of tools, in the world of Python data analysis, there are several "superstars" you absolutely can't miss:
-
NumPy: This is like the "super calculator" of the data analysis world. It lets you handle large amounts of numerical data as easily as playing with building blocks.
-
Pandas: If NumPy is a calculator, then Pandas is a smart spreadsheet processor. It lets you manipulate data like Excel, but much more powerfully.
-
Matplotlib: This is your personal artist. Whatever charts you want, it can draw them for you.
-
Seaborn: Think of it as Matplotlib's artistic friend. It can make your charts more beautiful and professional.
My favorite is Pandas. I still remember the first time I used Pandas to process a huge CSV file, I was so excited I jumped up. With just a few lines of code, I completed work that would have taken several hours to do manually. That feeling was like suddenly gaining superpowers!
Data Analysis Steps
Now that we have our tools, let's learn how to use them. Data analysis typically includes these steps:
-
Data Collection: This is like preparing ingredients. You need to collect data from various sources, which could be CSV files, databases, or even web APIs.
-
Data Cleaning: Imagine you're cooking - you need to clean the ingredients first, right? Same with data, we need to handle missing values, duplicate data, and outliers.
-
Data Exploration: This step is like tasting the ingredients. We use statistical methods and visualization tools to understand the characteristics of the data.
-
Data Modeling: Now we start the actual "cooking." We apply various algorithms to analyze the data and find patterns.
-
Results Interpretation: Finally, it's time to taste the results. We need to analyze the model output, draw conclusions, and make decisions based on them.
Each step is important and indispensable. I remember once I skipped the data cleaning step, and the conclusions I drew were completely wrong! That experience taught me the deep meaning of "garbage in, garbage out."
Practical Example
Let's look at a concrete example. Suppose we have a CSV file recording a coffee shop's sales data for a year. We want to analyze which type of coffee is most popular and how sales change over time.
import pandas as pd
import matplotlib.pyplot as plt
data = pd.read_csv('coffee_sales.csv')
print(data.head())
cleaned_data = data.dropna()
coffee_sales = cleaned_data.groupby('coffee_type')['quantity'].sum().sort_values(descending=True)
plt.figure(figsize=(10, 6))
coffee_sales.plot(kind='bar')
plt.title('Coffee Sales Ranking')
plt.xlabel('Coffee Type')
plt.ylabel('Sales Quantity')
plt.show()
monthly_sales = cleaned_data.groupby(pd.to_datetime(cleaned_data['date']).dt.to_period('M'))['total_price'].sum()
plt.figure(figsize=(12, 6))
monthly_sales.plot(kind='line', marker='o')
plt.title('Monthly Sales Trend')
plt.xlabel('Month')
plt.ylabel('Sales')
plt.show()
See, it's that simple! We used Pandas to read the data, cleaned it, then used the groupby function for simple data aggregation. Finally, we used Matplotlib to draw two charts: one showing sales volumes for different types of coffee, and another showing monthly sales trends.
When I first saw the charts generated by my code, that sense of achievement was indescribable. You know what? This kind of visualization not only helps us better understand the data but also makes our analysis results easier for others to understand. In the business world, this is a very important skill!
Advanced Techniques
Of course, data analysis goes far beyond this. As your skills improve, you'll find many advanced techniques waiting to be explored:
-
Data Preprocessing: Techniques like standardization and normalization can make your data more suitable for modeling.
-
Feature Engineering: This is an art - how to extract useful features from raw data often determines your model's performance.
-
Advanced Statistical Analysis: Things like hypothesis testing and regression analysis can help you understand data more deeply.
-
Machine Learning Integration: Combining data analysis with machine learning can achieve more powerful prediction and classification capabilities.
I remember once when analyzing user behavior data, I couldn't see any patterns just looking at the raw data. But when I applied some feature engineering techniques, like calculating user activity levels and consumption frequency as derived features, the data suddenly came alive, and user behavior patterns became clear. At that moment, I truly experienced the charm of data analysis!
Continuous Learning
In the field of data analysis, learning never stops. Technology keeps advancing, and new libraries and tools keep emerging. I suggest:
-
Read Books: "Python for Data Analysis" is an excellent book, written by the creator of Pandas, with very practical content.
-
Practice: Theory is important, but practice is more important. Find some real datasets to analyze, and you'll learn more.
-
Join Communities: Join some Python or data analysis online communities to exchange experiences with other learners.
-
Stay Current: Regularly check popular projects on GitHub to learn about the latest tools and technologies.
Remember, every data analysis expert started as a beginner. Keep your curiosity, continue learning and practicing, and you too can become a master in the field of data analysis!
Well, that's all for today's sharing. What do you think? Are you interested in Python data analysis? Or are you already using Python for data analysis? Feel free to share your experiences and thoughts in the comments. Let's explore this magical world of data together!
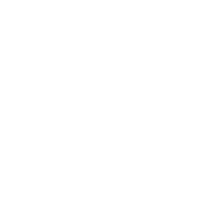
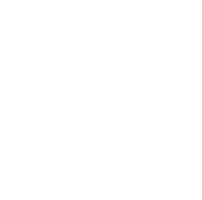