Hey everyone! Today let's talk about an important but often overlooked topic in game development - collision detection optimization. Have you encountered situations where your game starts lagging when there are too many objects? Or maybe you've written collision detection code but feel the results aren't quite ideal? Today I'll share some experiences and insights I've gathered during my development journey.
Basic Concepts
Before diving deep, let's understand the basic concept of collision detection. Simply put, collision detection determines whether objects in the game world have made contact or overlapped. Sounds simple, right? But doing it well isn't easy.
Simplest Implementation
Let's look at how basic rectangular collision detection is implemented:
import pygame
pygame.init()
rect1 = pygame.Rect(100, 100, 50, 50)
rect2 = pygame.Rect(120, 120, 50, 50)
if rect1.colliderect(rect2):
print("Collision detected: The rectangles collided!")
else:
print("Collision detection: The rectangles did not collide.")
pygame.quit()
This code demonstrates the most basic collision detection method. Seems pretty simple, right? Indeed, this is perfectly fine for just two objects. But real games aren't this simple.
Have you played games like "Plants vs. Zombies"? Imagine what happens when dozens of zombies and peas appear on screen simultaneously - what would happen if we used the simplest method to detect collisions between them?
Problems with Brute Force Detection
Let's see what it looks like using the brute force method to detect collisions between multiple objects:
import pygame
import random
pygame.init()
rects = [pygame.Rect(random.randint(0, 800), random.randint(0, 600), 50, 50)
for _ in range(100)]
collision_count = 0
for i in range(len(rects)):
for j in range(i + 1, len(rects)):
if rects[i].colliderect(rects[j]):
collision_count += 1
print(f"Collision detection (brute force): Detected {collision_count} collisions.")
pygame.quit()
This code doesn't look complex either, but analyzing it reveals the issue. With n objects, we need n*(n-1)/2 checks. What does this mean? Let me break it down:
- 10 objects: 45 checks needed
- 100 objects: 4950 checks needed
- 1000 objects: 499500 checks needed
Seeing these numbers, you should understand why games lag! And this is just the calculation for one frame! Remember, a smooth game needs at least 60 frames per second, meaning hundreds of thousands of collision checks per second!
Optimization Solutions
So, how can we improve this situation? This is where spatial partitioning techniques come in.
Grid Partitioning
The easiest optimization to understand is grid partitioning. Imagine dividing the game world into small cells - we only need to check for collisions between objects in the same cell. Wouldn't this greatly reduce the number of checks?
Here's how to implement it:
class Grid:
def __init__(self, width, height, cell_size):
self.cell_size = cell_size
self.cols = width // cell_size
self.rows = height // cell_size
self.cells = [[[] for _ in range(self.cols)] for _ in range(self.rows)]
def get_cell(self, x, y):
col = x // self.cell_size
row = y // self.cell_size
return max(0, min(col, self.cols - 1)), max(0, min(row, self.rows - 1))
def insert_object(self, obj):
cell_x, cell_y = self.get_cell(obj.rect.x, obj.rect.y)
self.cells[cell_y][cell_x].append(obj)
def get_nearby_objects(self, obj):
cell_x, cell_y = self.get_cell(obj.rect.x, obj.rect.y)
nearby = []
for dy in [-1, 0, 1]:
for dx in [-1, 0, 1]:
new_x = cell_x + dx
new_y = cell_y + dy
if 0 <= new_x < self.cols and 0 <= new_y < self.rows:
nearby.extend(self.cells[new_y][new_x])
return nearby
This method is simple to implement and works well when objects are evenly distributed. However, if objects are unevenly distributed, like concentrated in one area, the effectiveness decreases.
Quadtree Optimization
For unevenly distributed objects, we can use a quadtree data structure which dynamically divides space:
class QuadTreeNode:
def __init__(self, boundary, capacity):
self.boundary = boundary
self.capacity = capacity
self.objects = []
self.divided = False
self.northwest = None
self.northeast = None
self.southwest = None
self.southeast = None
def insert(self, obj):
if not self.boundary.contains(obj.rect):
return False
if len(self.objects) < self.capacity:
self.objects.append(obj)
return True
if not self.divided:
self.subdivide()
return (self.northwest.insert(obj) or
self.northeast.insert(obj) or
self.southwest.insert(obj) or
self.southeast.insert(obj))
def subdivide(self):
x = self.boundary.x
y = self.boundary.y
w = self.boundary.width // 2
h = self.boundary.height // 2
self.northwest = QuadTreeNode(pygame.Rect(x, y, w, h), self.capacity)
self.northeast = QuadTreeNode(pygame.Rect(x + w, y, w, h), self.capacity)
self.southwest = QuadTreeNode(pygame.Rect(x, y + h, w, h), self.capacity)
self.southeast = QuadTreeNode(pygame.Rect(x + w, y + h, w, h), self.capacity)
self.divided = True
This method is particularly effective when handling unevenly distributed objects. For example, in a large RPG game where players might interact with many NPCs in a village while other areas are sparse, a quadtree can automatically adapt to this distribution pattern.
Further Optimizations
You might ask: are there more advanced optimization methods? Of course!
Multithreading Optimization
If your game needs to handle hundreds or thousands of objects, consider using multithreading for parallel collision detection:
import threading
class CollisionDetector:
def __init__(self, num_threads=4):
self.num_threads = num_threads
self.threads = []
self.results = []
def detect_collisions(self, objects_chunk):
collisions = []
for i in range(len(objects_chunk)):
for j in range(i + 1, len(objects_chunk)):
if objects_chunk[i].rect.colliderect(objects_chunk[j].rect):
collisions.append((objects_chunk[i], objects_chunk[j]))
self.results.append(collisions)
def parallel_detect(self, objects):
chunk_size = len(objects) // self.num_threads
self.results = []
for i in range(self.num_threads):
start = i * chunk_size
end = start + chunk_size if i < self.num_threads - 1 else len(objects)
chunk = objects[start:end]
thread = threading.Thread(target=self.detect_collisions, args=(chunk,))
self.threads.append(thread)
thread.start()
for thread in self.threads:
thread.join()
return [collision for chunk_result in self.results for collision in chunk_result]
GPU Acceleration
For larger-scale collision detection, we can even consider using GPU acceleration. While this requires additional technical expertise, the results are significant. However, this topic might need its own article.
Practical Experience Sharing
In my development experience, choosing the right collision detection solution often requires balancing multiple factors:
-
Game Type: Different games have different collision detection requirements. Fighting games need extremely precise collision detection, while strategy games might not need such precision.
-
Number of Objects: If a game has few objects, the simplest method might be better; but with many objects, spatial partitioning optimization becomes necessary.
-
Object Distribution: If objects are evenly distributed, grid partitioning is sufficient; if unevenly distributed, consider using a quadtree.
-
Hardware Conditions: Mobile platform games need more performance optimization; PC platform games can use more complex algorithms.
Performance Testing
To help understand the performance differences between methods more intuitively, I created a simple test:
import time
import random
def performance_test(num_objects=1000, iterations=100):
# Create test objects
objects = [pygame.Rect(
random.randint(0, 800),
random.randint(0, 600),
20, 20
) for _ in range(num_objects)]
# Test brute force method
start_time = time.time()
for _ in range(iterations):
collisions = []
for i in range(len(objects)):
for j in range(i + 1, len(objects)):
if objects[i].colliderect(objects[j]):
collisions.append((i, j))
bruteforce_time = time.time() - start_time
# Test quadtree method
quadtree = QuadTree(pygame.Rect(0, 0, 800, 600), 4)
start_time = time.time()
for _ in range(iterations):
quadtree.clear()
for obj in objects:
quadtree.insert(obj)
collisions = quadtree.get_all_collisions()
quadtree_time = time.time() - start_time
print(f"Brute force time: {bruteforce_time:.2f} seconds")
print(f"Quadtree time: {quadtree_time:.2f} seconds")
Running this test shows: - With 100 objects, performance difference isn't noticeable - With 1000 objects, quadtree method's advantages become apparent - With over 10000 objects, brute force becomes unusable while quadtree maintains good performance
Practical Advice
Finally, here are some practical tips:
-
Start Simple: Use the simplest collision detection method during initial development. Only consider optimization when performance issues arise.
-
Analyze Requirements: Carefully analyze what kind of collision detection your game really needs. Sometimes a simplified collision detection scheme might be more suitable than a perfect but complex one.
-
Test-Driven: When implementing complex collision detection systems, write comprehensive test cases. Collision detection bugs are often hard to spot visually.
-
Performance Monitoring: Conduct regular performance tests during development to identify and resolve performance issues early.
-
Optimization Timing: Don't optimize too early. Focusing too much on performance optimization before the game is fully formed might be counterproductive.
Future Outlook
As game technology continues to evolve, collision detection technology also advances. Some research is now exploring using machine learning to optimize collision detection. While these technologies aren't mature yet, they show great potential.
How do you think collision detection technology will develop in the future? Feel free to share your thoughts in the comments. If you've encountered collision detection issues in your development, you can also discuss them.
Remember, programming is like solving puzzles - each problem solved helps us improve. I hope this article helps you go further in game development. Next time we can discuss other interesting game development topics, like implementing smooth physics effects or optimizing game rendering performance.
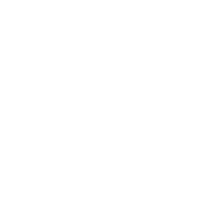
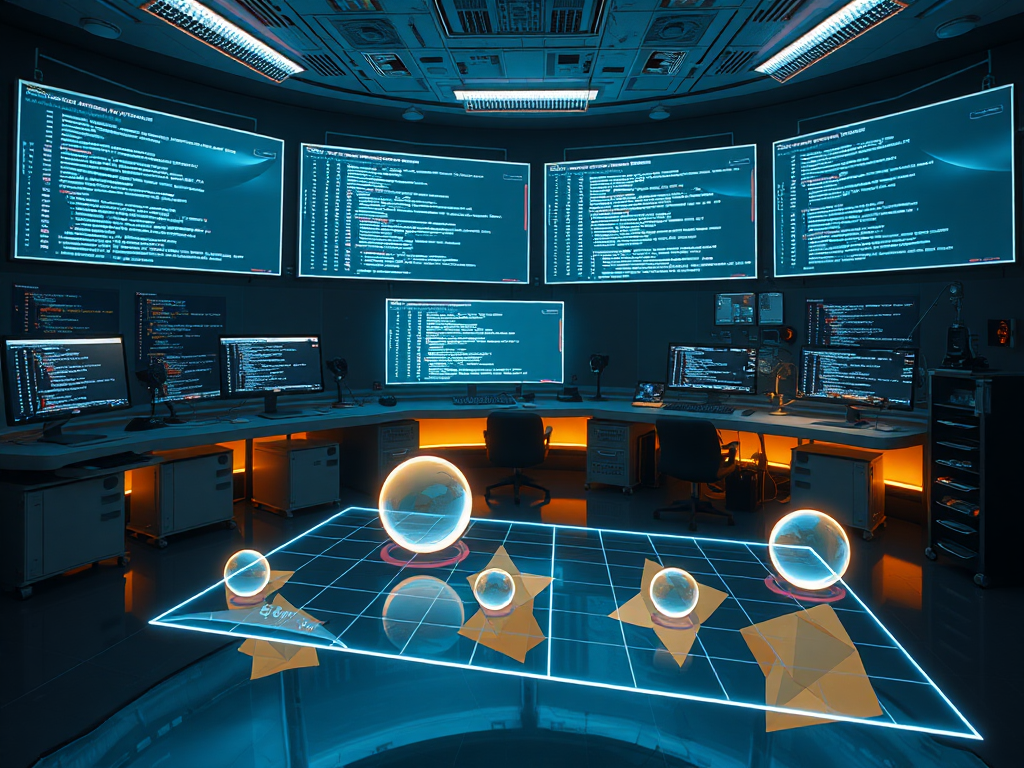
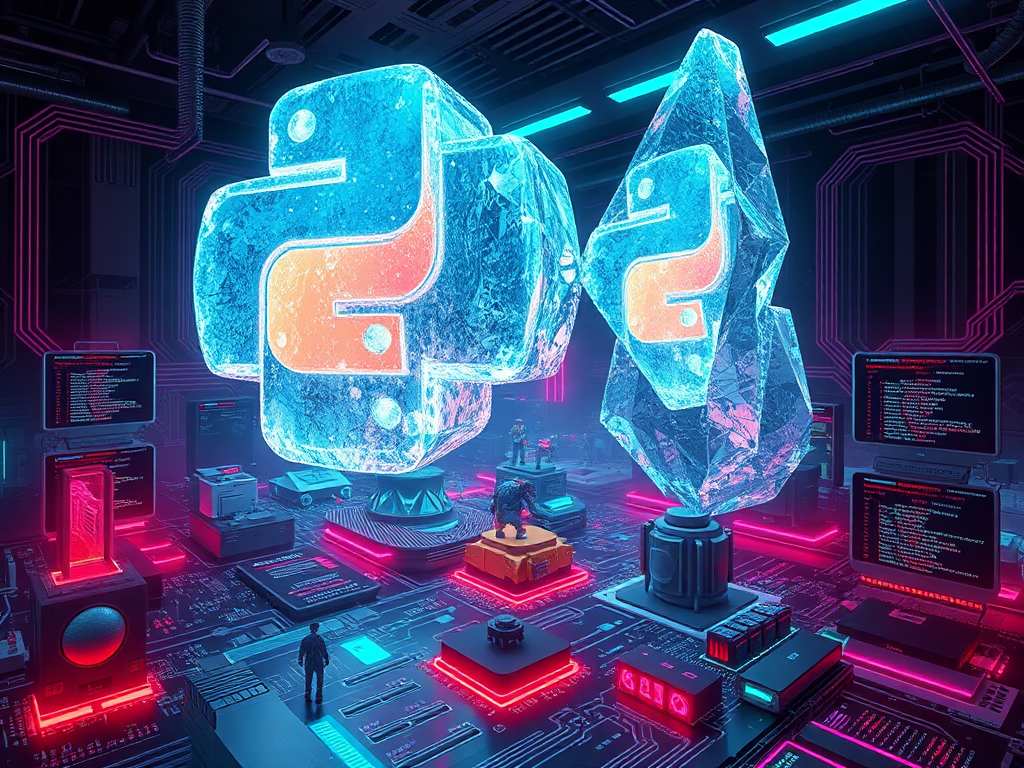
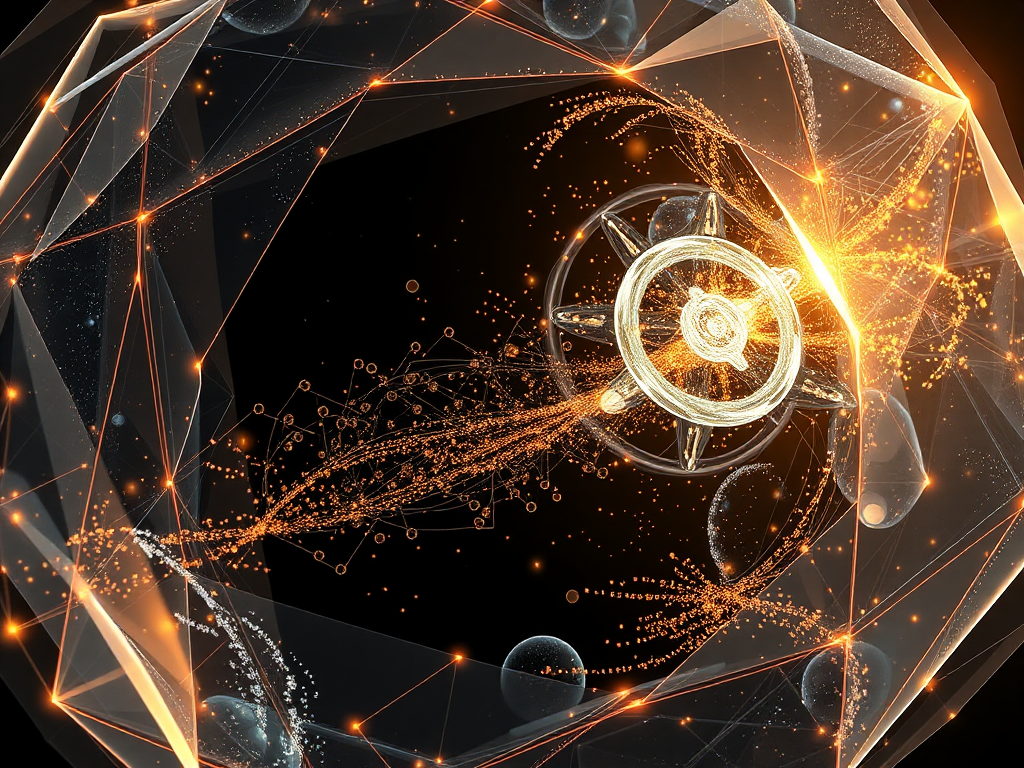