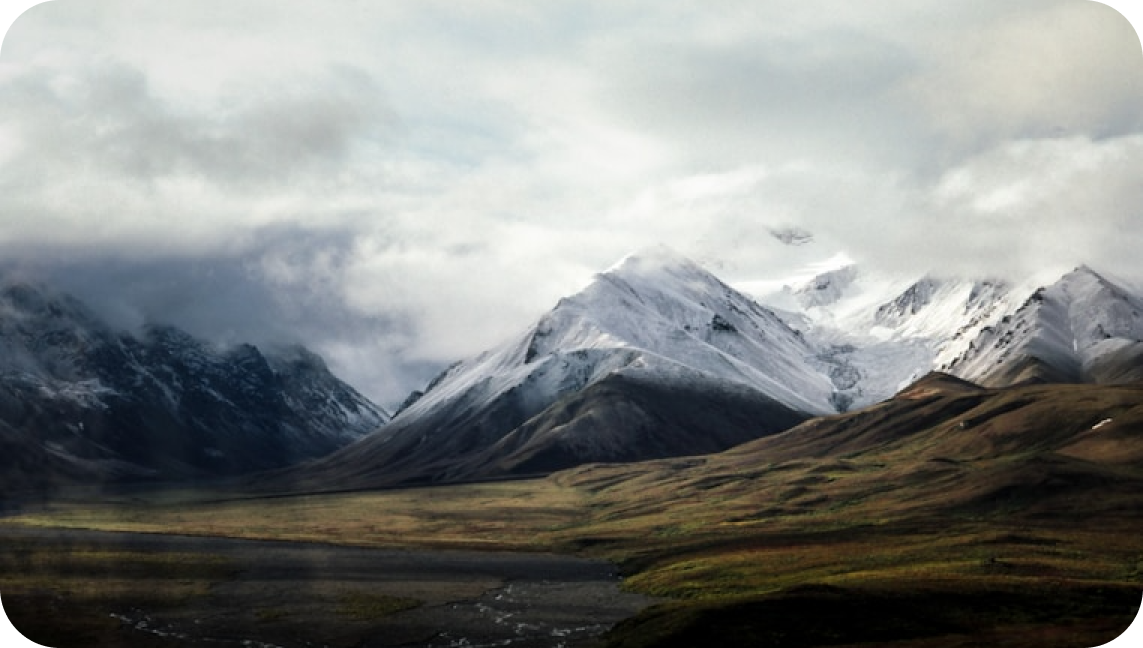
Origin
Have you ever wanted to develop your own game? As a Python programmer, I often get asked: "Can you really develop games with Python?" The answer is definitely yes. Today, I'll guide you step by step through developing a simple but complete 2D side-scrolling action game using Python.
Technology Selection
When it comes to Python game development, many people's first thought is Pygame. But before formally introducing Pygame, we need to understand why we choose Python for game development.
Python's strengths lie in its concise syntax and rich ecosystem. I remember when I first started learning game development, I was overwhelmed by C++'s complex syntax and memory management. In comparison, Python's learning curve is much gentler.
Of course, Python's performance isn't as good as C++. But for 2D games, Python's performance is completely adequate. My first game project was completed using Python, and it ran quite smoothly.
Environment
Before starting development, we need to set up an appropriate development environment. Python 3.8 or higher is recommended, as newer versions provide more useful features. For example, the walrus operator (:=) introduced in 3.8 is particularly useful in game loops.
pip install pygame
import pygame
pygame.init()
print(f"Pygame version: {pygame.version.ver}")
Architecture
The most important aspect of game development is proper architectural design. Through multiple projects, I've developed a architectural pattern suitable for small games:
class Game:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((800, 600))
self.clock = pygame.time.Clock()
self.running = True
def handle_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.running = False
def update(self):
# Game logic update
pass
def render(self):
# Render game screen
self.screen.fill((0, 0, 0))
pygame.display.flip()
def run(self):
while self.running:
self.handle_events()
self.update()
self.render()
self.clock.tick(60)
Why did I choose this architecture? Mainly based on these considerations:
- Clear separation of responsibilities
- Easy to expand and maintain
- Follows common game development patterns
Sprites
In 2D games, sprites are the most basic game objects. Let's implement a player sprite:
class Player(pygame.sprite.Sprite):
def __init__(self, pos_x, pos_y):
super().__init__()
self.image = pygame.Surface((32, 32))
self.image.fill((255, 0, 0))
self.rect = self.image.get_rect()
self.rect.x = pos_x
self.rect.y = pos_y
# Physical properties
self.velocity_x = 0
self.velocity_y = 0
self.acceleration = 0.5
self.max_speed = 8
self.jump_power = -12
self.on_ground = False
def update(self):
# Gravity
if not self.on_ground:
self.velocity_y += self.acceleration
# Limit maximum speed
self.velocity_x = max(min(self.velocity_x, self.max_speed), -self.max_speed)
# Update position
self.rect.x += self.velocity_x
self.rect.y += self.velocity_y
Physics
Game physics is key to making a game feel realistic. Let's implement a simple physics system:
class PhysicsEngine:
def __init__(self, gravity=0.5):
self.gravity = gravity
self.platforms = pygame.sprite.Group()
def add_platform(self, platform):
self.platforms.add(platform)
def update(self, player):
# Apply gravity
if not player.on_ground:
player.velocity_y += self.gravity
# Detect collisions
hits = pygame.sprite.spritecollide(player, self.platforms, False)
if hits:
lowest = hits[0]
for hit in hits:
if hit.rect.bottom > lowest.rect.bottom:
lowest = hit
if player.velocity_y > 0:
player.rect.bottom = lowest.rect.top
player.velocity_y = 0
player.on_ground = True
Animation
Game animations make games more lively. Here we implement a simple sprite animation system:
class Animation:
def __init__(self, frames, frame_duration):
self.frames = frames
self.frame_duration = frame_duration
self.current_frame = 0
self.timer = 0
def update(self, dt):
self.timer += dt
if self.timer >= self.frame_duration:
self.timer = 0
self.current_frame = (self.current_frame + 1) % len(self.frames)
def get_current_frame(self):
return self.frames[self.current_frame]
Sound Effects
Good sound effects can greatly enhance the game experience. My experience is: it's better to have no sound effects than to use inappropriate ones.
class SoundManager:
def __init__(self):
self.sounds = {}
def load_sound(self, name, path):
self.sounds[name] = pygame.mixer.Sound(path)
def play_sound(self, name):
if name in self.sounds:
self.sounds[name].play()
Optimization
Optimization is an eternal topic in game development. Here are some optimization tips I've summarized from practice:
- Use Object Pool
class ObjectPool:
def __init__(self, create_func, pool_size=100):
self.create_func = create_func
self.pool = [create_func() for _ in range(pool_size)]
self.active_objects = []
def get_object(self):
if self.pool:
obj = self.pool.pop()
self.active_objects.append(obj)
return obj
return self.create_func()
def return_object(self, obj):
if obj in self.active_objects:
self.active_objects.remove(obj)
self.pool.append(obj)
- Quadtree Spatial Partitioning
class QuadTree:
def __init__(self, boundary, capacity):
self.boundary = boundary
self.capacity = capacity
self.objects = []
self.divided = False
def insert(self, obj):
if not self.boundary.contains(obj):
return False
if len(self.objects) < self.capacity:
self.objects.append(obj)
return True
if not self.divided:
self.subdivide()
return (self.northeast.insert(obj) or
self.northwest.insert(obj) or
self.southeast.insert(obj) or
self.southwest.insert(obj))
Debugging
Debugging is an essential part of game development. I developed a simple debugging tool:
class DebugInfo:
def __init__(self, game):
self.game = game
self.font = pygame.font.Font(None, 24)
self.show = True
def render(self, screen):
if not self.show:
return
info = [
f"FPS: {int(self.game.clock.get_fps())}",
f"Objects: {len(self.game.all_sprites)}",
f"Player pos: ({self.game.player.rect.x}, {self.game.player.rect.y})"
]
for i, text in enumerate(info):
surface = self.font.render(text, True, (255, 255, 255))
screen.blit(surface, (10, 10 + i * 25))
Insights
Developing this game project made me deeply realize that game development is not just programming, but a comprehensive art. Here are some of my insights:
-
Start Small Don't try to make a big game from the start. My suggestion is to first make a minimum viable version, then gradually add features.
-
Value Architecture Good architecture can make later development much easier. I've learned the hard way that poor architectural design makes later modifications very difficult.
-
Keep It Simple Remember the KISS principle (Keep It Simple, Stupid). Sometimes, simple implementations can actually bring better game experiences.
-
Value Feedback Frequently have others test your game and collect feedback. I've found that player feedback often brings unexpected insights.
Future Outlook
Python game development still has a lot of room for growth. With technological advancement, Python's applications in game development will become increasingly widespread.
Finally, I want to say, don't let technology limit your imagination. Python may not be the fastest language, but it's sufficient to realize your game ideas. What's important is to start acting, learning, and growing through practice.
What do you think about this article? Feel free to share your game development experiences and thoughts in the comments. If you have any questions, you can also ask me anytime. Let's continue forward together on the path of Python game development.
Next
Python Game Development in Practice: Building a 2D Side-Scrolling Action Game from Scratch
An in-depth exploration of Python programming in game development, covering Python syntax features, game development processes, Pygame framework applications, and game AI development
Advanced Python Game Development
Explore the application of Python in game development, including its advantages, commonly used tools, and core technologies. The article provides a detailed int
A Guide to Performance Optimization in Python Game Development: From Beginner to Master
A comprehensive analysis of Python programming language in game development, examining its features, development frameworks, and performance limitations to help developers understand Python's role in game development
Next
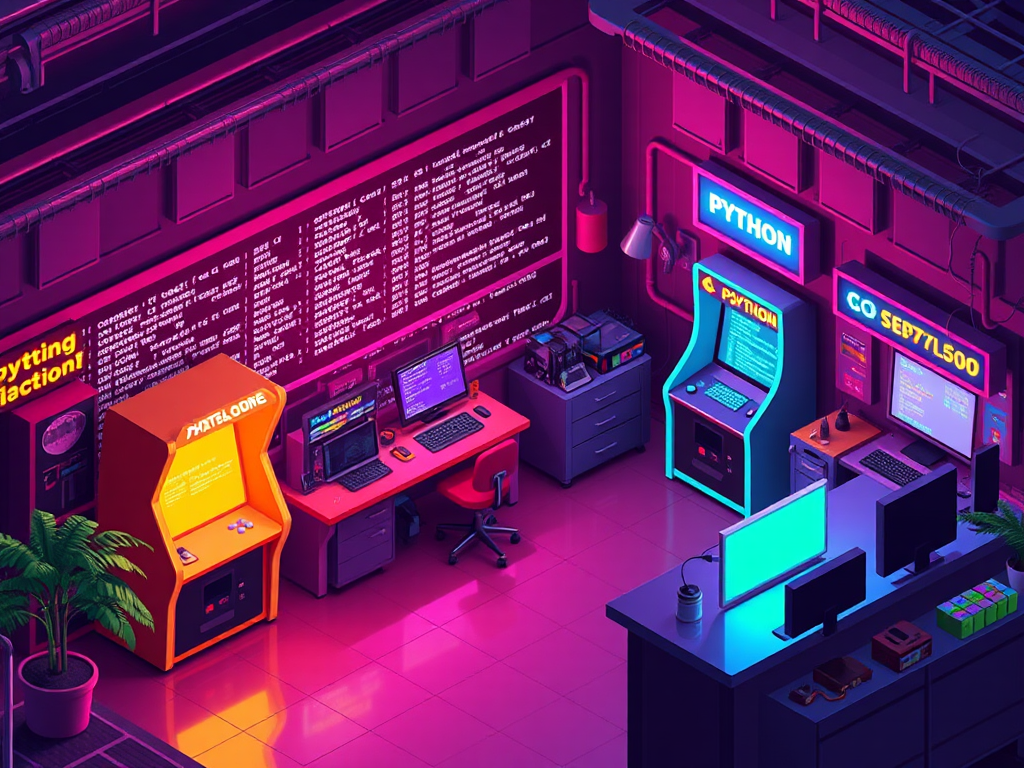
Python Game Development in Practice: Building a 2D Side-Scrolling Action Game from Scratch
An in-depth exploration of Python programming in game development, covering Python syntax features, game development processes, Pygame framework applications, and game AI development
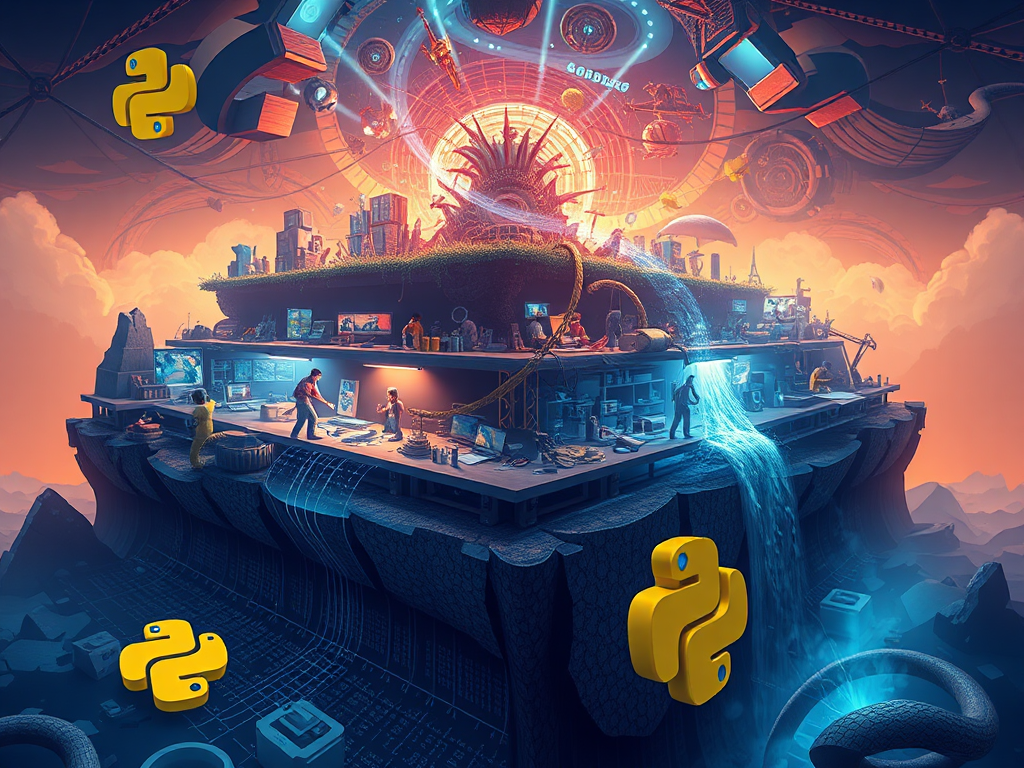
Advanced Python Game Development
Explore the application of Python in game development, including its advantages, commonly used tools, and core technologies. The article provides a detailed int
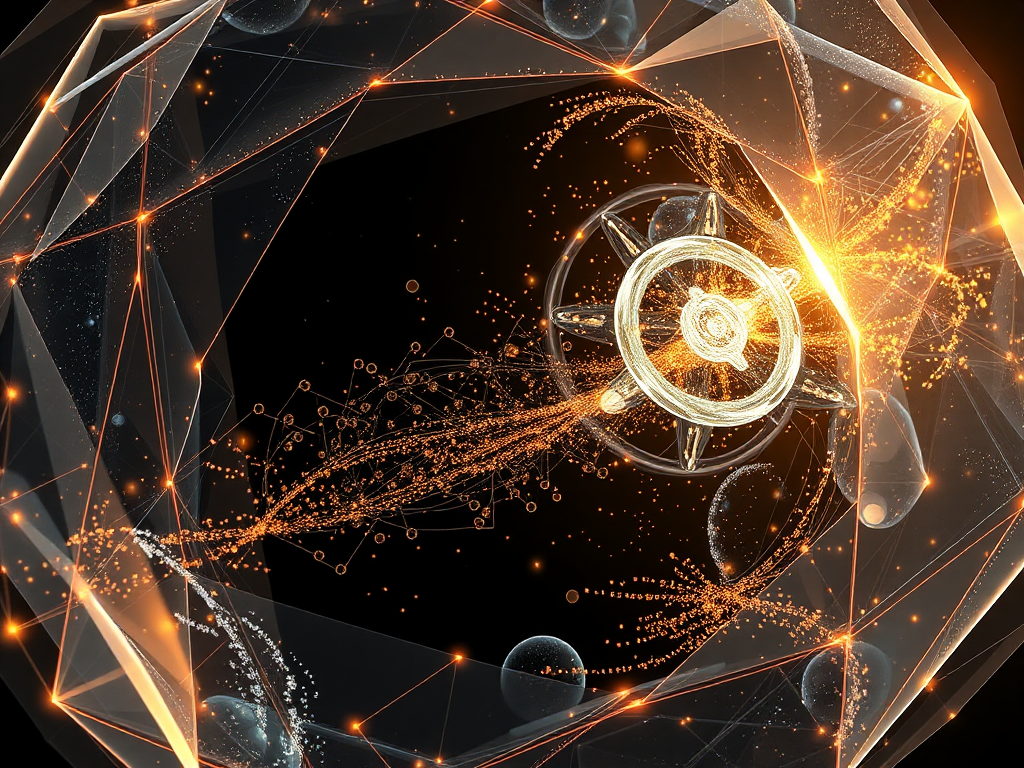
A Guide to Performance Optimization in Python Game Development: From Beginner to Master
A comprehensive analysis of Python programming language in game development, examining its features, development frameworks, and performance limitations to help developers understand Python's role in game development